The Role of Truth Tables in Test Verification: Ensuring Correct Functionality of Circuits and Systems (Expert Analysis)
发布时间: 2024-09-15 09:14:59 阅读量: 8 订阅数: 14 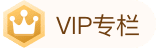
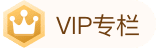
# 1. Introduction to Truth Tables
A truth table is a logical table that displays the output values of a logic function or circuit under all possible combinations of inputs. It is an organized table where the values of input variables are listed in the header and the values of output variables are listed in the table. Truth tables play a crucial role in testing and verification, as they provide a comprehensive view of the behavior of logical circuits and systems.
# 2. Application of Truth Tables in Testing and Verification
### 2.1 Role of Truth Tables in Logical Circuit Testing
#### 2.1.1 Generation and Analysis of Truth Tables
A truth table is a fundamental tool for describing the behavior of a logical circuit. It lists all possible input combinations and their corresponding outputs. By analyzing the truth table, we can determine the function of the circuit, detect faults, and generate test cases.
**Code Block:**
```python
def generate_truth_table(circuit):
"""Generate a truth table for a given logical circuit.
Args:
circuit: A logical circuit object.
Returns:
A truth table containing all possible input combinations and their corresponding outputs.
"""
# Get the input and output variables of the circuit.
inputs = circuit.inputs
outputs = circuit.outputs
# Create a list of all possible input combinations.
input_combinations = []
for i in range(2**len(inputs)):
input_combination = []
for j in range(len(inputs)):
input_combination.append((i >> j) & 1)
input_combinations.append(input_combination)
# Create a truth table containing all possible outputs of the circuit.
truth_table = []
for input_combination in input_combinations:
output_combination = []
for output in outputs:
output_combination.append(circuit.evaluate(input_combination))
truth_table.append(output_combination)
return truth_table
```
**Logical Analysis:**
This code block defines a function `generate_truth_table` that generates the truth table for a given logical circuit. The function first retrieves the input and output variables of the circuit. Then, it creates a list of all possible input combinations. Next, it creates a truth table containing all possible outputs of the circuit. Finally, it returns the truth table.
#### 2.1.2 Fault Detection and Diagnosis
Truth tables can be used to detect and diagnose faults in logical circuits. By comparing the actual outputs of the circuit with the expected outputs from the truth table, we can identify faults.
**Code Block:**
```python
def detect_faults(circuit, truth_table):
"""Detect faults in a given logical circuit.
Args:
circuit: A logical circuit object.
truth_table: The truth table of the circuit.
Returns:
A list containing all detected faults in the circuit.
"""
# Get the input and output variables of the circuit.
inputs = circuit.inputs
outputs = circuit.outputs
# Create a list of all possible input combinations.
input_combinations = []
for i in range(2**len(inputs)):
input_combination = []
for j in range(len(inputs)):
input_combination.append((i >> j) & 1)
input_combinations.append(input_combination)
# Create a list to store detected faults.
faults = []
# Compare the actual output of the circuit with the expected output from the truth table for each possible input combination.
for input_combination, expected_output in zip(input_combinations, truth_table):
actual_output = circuit.evaluate(input_combination)
if actual_output != expected_output:
# A fault has been detected. Add it to the faults list.
faults.append(input_combination)
return faults
```
**Logical Analysis:**
This code block defines a function `detect_faults` that detects faults in a given logical circuit. The function first retrieves the input and output variables of the circuit. Then, it creates a list of all possible input combinations. Next, it creates a list to store detected faults. For each possible input combination, it compares the actu
0
0
相关推荐
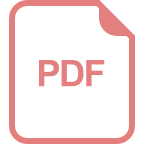
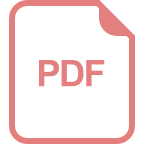
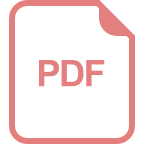
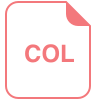




