Applications of Truth Tables in Cryptography: Building Secure and Reliable Encryption Systems (Expert Interpretation)
发布时间: 2024-09-15 09:06:27 阅读量: 18 订阅数: 18 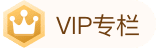
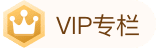
# The Concept and Characteristics of Truth Tables
A truth table is a logical table that displays the output values of a Boolean function for a given set of input values. It is a two-dimensional table in which rows represent combinations of input values, and columns represent output values. An important characteristic of a truth table is its completeness, which means it includes all possible combinations of input values and their corresponding output values.
A truth table also exhibits duality, which means that for any truth table, another truth table can be constructed with the same output values but with the input and output values swapped. Additionally, truth tables can be used to simplify Boolean functions; by using Karnaugh maps or the Quine-McCluskey method, one can find the minimum or maximum terms of a function.
# The Application of Truth Tables in Cryptography
### 2.1 The Principle of Truth Table Application in Cryptography
The application of truth tables in cryptography is primarily based on their logical operation characteristics. Essentially, a truth table is a logical function that maps a set of input values to a set of output values, with the output depending on the truthfulness of the input values. In cryptography, truth tables can be used to perform various logical operations, such as:
- **Boolean operations:** AND, OR, NOT, XOR, and other Boolean operations can be implemented through truth tables. These operations are widely used in cryptographic algorithms for combining and processing data.
- **Conditional operations:** Conditional operations like IF-THEN-ELSE can also be implemented through truth tables. These operations are used in cryptographic protocols to control flow and make decisions.
- **Lookup tables:** A truth table can act as a lookup table to find the output value corresponding to a specific input value. This characteristic is used in cryptographic algorithms for quick lookup and retrieval of data.
### 2.2 The Role of Truth Tables in Cryptographic Algorithms
Truth tables play a crucial role in cryptographic algorithms, specifically:
- **Key generation:** Truth tables can be used to generate random keys or pseudo-random sequences. By randomizing input values or using complex logical operations, truth tables can produce unpredictable outputs, thereby enhancing the security of cryptographic algorithms.
- **Data encryption:** Truth tables can be used to encrypt data. By inputting plaintext into the truth table and generating ciphertext according to its logical operation rules, truth tables can obfuscate and hide the original content of the data.
- **Data decryption:** Truth tables can also be used to decrypt ciphertext. By reversing the logical operation process of the truth table and inputting the correct key, plaintext data can be restored.
- **Hash functions:** Truth tables can be used to implement hash functions. A hash function is a one-way function that maps input data to a fixed-length output. Through complex logical operations, truth tables can generate hash values that are difficult to reverse, used for data integrity verification and digital signatures.
#### Code Block Example:
```python
def true_table_encryption(plaintext, key):
"""
Encrypts plaintext using a truth table.
Parameters:
plaintext: The plaintext data
key: The encryption key
Returns:
The encrypted ciphertext
"""
# Convert plaintext and key to binary representation
plaintext_binary = bin(int(plaintext, 16))[2:]
key_binary = bin(int(key, 16))[2:]
# Create a truth table
truth_table = [
[0, 0, 0],
[0, 1, 1],
[1, 0, 1],
[1, 1, 0]
]
# Initialize ciphertext
ciphertext_binary = ""
# Iterate through each bit of plaintext and key
for i in range(len(plaintext_binary)):
# Get the current bits of plaintext and key
plaintext_bit = int(plaintext_binary[i])
key_bit = int(key_binary[i])
# Find the output based on the truth table
output_bit = truth_table[plaintext_bit][key_bit]
# Append the output bit to the ciphertext
ciphertext_binary += str(output_bit)
# Convert the ciphertext to hexadecimal representation
ciphertext = hex(int(ciphertext_binary, 2))[2:]
# Return the ciphertext
return ciphertext
```
#### Logical Analysis:
The code block above implements a function that encrypts plaintext using a truth table. The function takes plaintext and key as parameters and returns the ciphertext.
1. First, convert plaintext and key to binary representation.
2. Create a truth table containing all possible input and output values.
3. Initialize an empty string to store the ciphertext.
4. Iterate over each bit of plaintext and key.
5. Get the current bits of plaintext and key.
6. Look up the output bit based on the truth table.
7. Append the output bit to the ciphertext.
8. Convert the ciphertext to hexadecimal represen
0
0
相关推荐
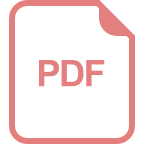
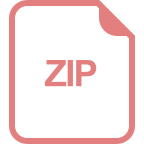
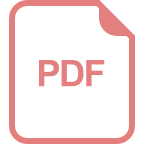





