Truth Tables and Boolean Algebra: Mathematical Bridges of Logical Operations (In-depth Analysis)
发布时间: 2024-09-15 09:00:35 阅读量: 21 订阅数: 17 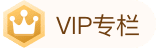
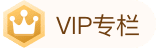
# 1. Introduction to Truth Tables and Boolean Algebra: The Mathematical Bridge of Logical Operations (In-depth Analysis)
## 2. Boolean Algebra Operations and Theorems
### 2.1 Definitions and Properties of Boolean Operations
#### 2.1.1 AND, OR, NOT Operations
Boolean operations are binary operations that act on two Boolean values (true or false) and produce a Boolean value as a result. The basic Boolean operations include:
- **AND operation:** The result is true if both Boolean values are true; otherwise, it is false.
- **OR operation:** The result is true if at least one of the Boolean values is true; otherwise, it is false.
- **NOT operation:** Inverts the Boolean value, changing true to false and false to true.
#### 2.1.2 XOR, IMPLICATION, EQUIVALENCE Operations
In addition to the basic operations, there are other Boolean operations:
- **XOR operation:** The result is true if the two Boolean values are different; otherwise, it is false.
- **IMPLICATION operation:** The result is true if the first Boolean value is true and the second is either true or false; otherwise, it is false.
- **EQUIVALENCE operation:** The result is true if the two Boolean values are the same; otherwise, it is false.
### 2.2 Boolean Algebra Theorems
Boolean algebra is an axiomatic system for manipulating Boolean values. It defines a set of theorems that can be used to simplify and manipulate Boolean expressions.
#### 2.2.1 Absorption, Associativity, and Distributivity Laws
- **Absorption Law:** A + AB = A, A * (A + B) = A
- **Associativity Law:** (A + B) + C = A + (B + C), (A * B) * C = A * (B * C)
- **Distributivity Law:** A * (B + C) = A * B + A * C, A + (B * C) = (A + B) * (A + C)
#### 2.2.2 De Morgan's Theorems and Duality Principle
- **De Morgan's Theorems:** ¬(A + B) = ¬A * ¬B, ¬(A * B) = ¬A + ¬B
- **Duality Principle:** A + B = ¬(¬A * ¬B), A * B = ¬(¬A + ¬B)
**Code Block:**
```python
# AND operation
a = True
b = False
result = a and b
print(result) # Output: False
# OR operation
a = True
b = False
result = a or b
print(result) # Output: True
# NOT operation
a = True
result = not a
print(result) # Output: False
```
**Logical Analysis:**
* AND operation: The result is true if both Boolean values are true; otherwise, it is false.
* OR operation: The result is true if at least one of the Boolean values is true; otherwise, it is false.
* NOT operation: Inverts the Boolean value, changing true to false and false to true.
**Argument Description:**
* `a`: The first Boolean value
* `b`: The second Boolean value
* `result`: The result of the Boolean operation
# 3. Practical Applications of Truth Tables
### 3.1 Applications in Logical Circuits
#### 3.1.1 Truth Tables for Logic Gates
Logic gates are the basic building blocks of digital circuits that perform Boolean operations. Each logic gate has a truth table that lists all possible input and output combinations. For example, the truth table for an AND gate is as follows:
| A | B | A AND B |
|---|---|---|
| 0 | 0 | 0 |
| 0 | 1 | 0 |
| 1 | 0 | 0 |
| 1 | 1 | 1 |
This table shows that the AND gate only outputs true when both A and B are true.
#### 3.1.2 Analysis and Design of Combinational Logic Circuits
A combinational logic circuit is made up of logic gates where the output is only dependent on the current inputs. To analyze a combinational logic circuit, a tr
0
0
相关推荐
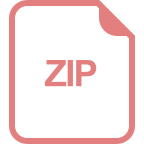
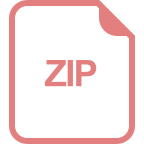
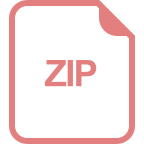
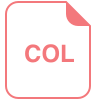
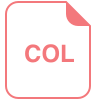
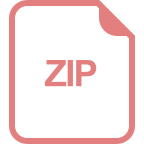
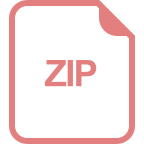
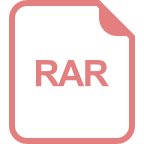
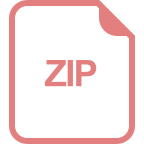