Unveiling the Truth Table: The Hidden Power Behind Logical Operations, Making Understanding Easy
发布时间: 2024-09-15 08:54:27 阅读量: 21 订阅数: 16 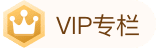
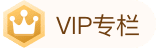
# Demystifying Truth Tables: The Unsung Heroes of Logical Operations for Easy Understanding
## 1. The Basics of Logical Operations**
Logical operations are methods used to manipulate logical propositions to determine their truth values. Logical operators are symbols that perform these operations, converting one or more input values (known as operands) into a single output value (known as the result).
Common logical operators include:
* Logical AND (AND)
* Logical OR (OR)
* Logical NOT (NOT)
* Logical XOR (XOR)
## 2. Truth Tables: Your Guide to Logical Operations
### 2.1 Concept and Structure of Truth Tables
**2.1.1 Components of a Truth Table**
A truth table is a table that shows the output results of a logical operator for all possible combinations of inputs. It consists of the following elements:
- **Input Columns:** List all possible combinations of inputs. For n input variables, there are 2^n possible input combinations.
- **Output Column:** Displays the output results of the logical operator for each input combination.
- **Operator Symbol:** Located at the top of the truth table, indicating the logical operator under consideration.
**2.1.2 How to Interpret a Truth Table**
To interpret a truth table, follow these steps:
1. Determine the specific input combination to be evaluated in the input columns.
2. Find the corresponding output column for that input combination.
3. The value in the output column represents the output result of the logical operator for that input combination.
### 2.2 Truth Tables for Logical Operators
**2.2.1 Logical AND (AND)**
| A | B | A AND B |
|---|---|---|
| 0 | 0 | 0 |
| 0 | 1 | 0 |
| 1 | 0 | 0 |
| 1 | 1 | 1 |
**Logical Analysis:** The AND operator outputs true only when both inputs are true.
**2.2.2 Logical OR (OR)**
| A | B | A OR B |
|---|---|---|
| 0 | 0 | 0 |
| 0 | 1 | 1 |
| 1 | 0 | 1 |
| 1 | 1 | 1 |
**Logical Analysis:** The OR operator outputs true if at least one input is true.
**2.2.3 Logical NOT (NOT)**
| A | NOT A |
|---|---|
| 0 | 1 |
| 1 | 0 |
**Logical Analysis:** The NOT operator inverts the input, changing 0 to 1 and 1 to 0.
**2.2.4 Logical XOR (XOR)**
| A | B | A XOR B |
|---|---|---|
| 0 | 0 | 0 |
| 0 | 1 | 1 |
| 1 | 0 | 1 |
| 1 | 1 | 0 |
**Logical Analysis:** The XOR operator outputs true only when the two inputs are different.
# 3.1 Evaluating Logical Expressions
**3.1.1 Evaluating Logical Expressions Using Truth Tables**
Using a truth table to evaluate logical expressions is a straightforward and effective process. For a given logical expression, we can create a truth table that includes all possible combinations of input variables and their corresponding output values. By examining the truth table, we can determine the truth value of the logical expression.
For example, consider the following logical expression:
```
(A AND B) OR (NOT C)
```
To evaluate this expression, we can create a truth table with all possible combinations of A, B, and C:
| A | B | C | (A AND B) | (NOT C) | (A AND B) OR (NOT C) |
|---|---|---|---|---|---|
| 0 | 0 | 0 | 0 | 1 | 1 |
| 0 | 0 | 1 | 0 | 0 | 0 |
| 0 | 1 | 0 | 0 | 1 | 1 |
| 0 | 1 | 1 | 0 | 0 | 0 |
| 1 | 0 | 0 | 0 | 1 | 1 |
| 1 | 0 | 1 | 0 | 0 | 0 |
| 1 | 1 | 0 | 1 | 1 | 1 |
| 1 | 1 | 1 | 1 | 0 | 1 |
By examining the truth table, we can see that the logical expression (A AND B) OR (NOT C) is true in the following cases:
* When both A and B are true
* When C is false
**3.1.2 Simplifying Logical Expressions**
Truth tables can also be used to simplify logical expressions. Simplification involves converting a logical expression into an equivalent but simpler form. By using truth tables, we can identify and eliminate redundant terms, resulting in a more streamlined expression.
For instance, consider the following logical expression:
```
(A AND B) OR (A AND NOT B)
```
We can create a truth table to simplify this expression:
| A | B | (A AND B) | (A AND NOT B) | (A AND B) OR (A AND NOT B) |
|---|---|---|---|---|
| 0 | 0 | 0 | 0 | 0 |
| 0 | 1 | 0 | 0 | 0 |
| 1 | 0 | 0 | 0 | 0 |
| 1 | 1 | 1 | 0 | 1 |
Upon reviewing the truth table, we can see that (A AND B) OR (A AND NOT B) is equal to A in all cases. Therefore, we can simplify the expression to:
```
(A AND B) OR (A AND NOT B) = A
```
# 4. Extended Applications of Truth Tables
### 4.1 Boolean Algebra and Truth Tables
#### 4.1.1 Basic Theorems of Boolean Algebra
Boolean algebra is an algebraic system defined on the Boolean values (true and false). It consists of the following basic theorems:
- **Commutative Law:** A AND B = B AND A, A OR B = B OR A
- **Associative Law:** (A AND B) AND C = A AND (B AND C), (A OR B) OR C = A OR (B OR C)
- **Distributive Law:** A AND (B OR C) = (A AND B) OR (A AND C), A OR (B AND C) = (A OR B) AND (A OR C)
- **Absorption Law:** A AND (A OR B) = A, A OR (A AND B) = A
- **Identity Element:** A AND TRUE = A, A OR FALSE = A
- **Zero Element:** A AND FALSE = FALSE, A OR TRUE = TRUE
- **De Morgan's Theorem:** NOT (A AND B) = NOT A OR NOT B, NOT (A OR B) = NOT A AND NOT B
#### 4.1.2 Applications of Truth Tables in Boolean Algebra
Truth tables can be used to verify theorems in Boolean algebra. For instance, to verify the commutative law, we can construct a truth table with all possible values for A, B, and A AND B:
| A | B | A AND B |
|---|---|---|
| T | T | T |
| T | F | F |
| F | T | F |
| F | F | F |
From the truth table, we can see that the value of A AND B is the same as B AND A, which verifies the commutative law.
### 4.2 Truth Tables in Computer Science
#### 4.2.1 The Role of Truth Tables in Computer Programming
Truth tables are extensively used in computer programming for:
- **Conditional Statements:** Truth tables can be used to determine the execution flow of conditional statements. For example, the following Python code uses a truth table to decide whether to print a message:
```python
a = True
b = False
if a and b:
print("Message")
```
- **Boolean Expressions:** Truth tables can be used to solve the values of Boolean expressions. For instance, the following truth table shows the values for the expression A OR B:
| A | B | A OR B |
|---|---|---|
| T | T | T |
| T | F | T |
| F | T | T |
| F | F | F |
#### 4.2.2 Applications of Truth Tables in Algorithm Design
Truth tables can be used for:
- **Designing Algorithms:** Truth tables can be used to design algorithms to determine which operations should be executed under specific conditions. For example, the following truth table illustrates an algorithm to determine the maximum value:
| A | B | Maximum |
|---|---|---|
| T | T | A |
| T | F | A |
| F | T | B |
| F | F | B |
- **Optimizing Algorithms:** Truth tables can be used to optimize algorithms by reducing execution time and resource consumption. For instance, by using a truth table, we can identify and eliminate redundant conditions in an algorithm.
# 5. Limitations and Challenges of Truth Tables
### 5.1 Truth Tables Cannot Handle Fuzzy Logic
Truth tables are based on two-valued logic, meaning they only consider true and false. However, many real-world problems possess ambiguity that cannot be explicitly represented as either true or false. For example, a person's health status could be "healthy," "subhealthy," or "unhealthy," and truth tables cannot account for this ambiguity.
### 5.2 Challenges in Applying Truth Tables to Complex Logical Systems
As the complexity of logical systems increases, the size and complexity of truth tables can grow exponentially. For complex logical systems with a large number of input variables, truth tables can become difficult to manage and analyze. For example, a logical system with 10 input variables would require a truth table with 2^10 = 1024 rows.
### 5.3 Alternative Methods to Truth Tables
To address the limitations of truth tables, alternative methods have been proposed for handling fuzzy logic and complex logical systems. These methods include:
- **Fuzzy Logic:** Fuzzy logic employs fuzzy sets to represent ambiguous concepts, allowing for different degrees between true and false.
- **Bayesian Networks:** Bayesian networks are probabilistic graphical models that use probabilities to represent relationships between events, capable of handling uncertainty and ambiguity.
- **Neural Networks:** Neural networks are machine learning models that can learn complex functions and process nonlinear relationships, including those of fuzzy logic.
0
0