Truth Tables and the Quine-McCluskey Algorithm: Another Powerful Tool for Logical Simplification (Expert Analysis)
发布时间: 2024-09-15 09:12:00 阅读量: 18 订阅数: 20 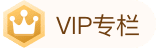
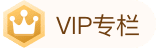
# 1. The Basics of Logical Simplification
Logical simplification is a crucial step in digital circuit design, which optimizes circuit performance by reducing logic gates and circuit complexity. The core of logical simplification technology is to understand the basic principles of logic functions and the application of truth tables.
A truth table is a table that shows the output values of a logic function under all possible combinations of input variables. By analyzing the truth table, we can identify the regularity and redundancy of the logic function, thus simplifying it. The goal of logic simplification is to find an equivalent logic function that uses the fewest logic gates and the simplest logical expression.
# 2. The Mystery of Truth Tables
### 2.1 Basic Concepts and Applications of Truth Tables
**Truth Table** is a tabular representation of logical operations, which shows the output values for all possible combinations of input variables. Truth tables are crucial for understanding the nature and behavior of logical operations.
**Basic Concepts:**
- **Input Variables:** Entries in a truth table represent input variables, which can take the values of true (1) or false (0).
- **Output Variables:** Rows in a truth table represent output variables, whose values depend on the combination of input variables.
- **Logical Operators:** Symbols in a truth table represent logical operators, such as AND, OR, NOT, etc.
**Applications:**
Truth tables are widely used for:
- **Verifying Logical Expressions:** By checking all possible input combinations, the correctness of logical expressions can be verified.
- **Simplifying Logical Expressions:** By analyzing the truth table, redundant input variables can be identified and eliminated, thus simplifying the logical expression.
- **Designing Logical Circuits:** Truth tables can serve as the basis for designing logical circuits, by determining the types and connections of logic gates needed in the circuit.
### 2.2 Simplification Methods of Truth Tables
**Karnaugh Map:**
A Karnaugh map is a graphical tool for representing truth tables that can simplify the process of logical expression. The Karnaugh map groups adjacent rows and columns in the truth table to form a grid, where adjacent cells represent input combinations with the same variable values. By analyzing the Karnaugh map, adjacent cells can be identified and combined, thus simplifying the logical expression.
**Code Block:**
```python
def simplify_with_karnaugh_map(truth_table):
"""
Use Karnaugh map to simplify truth table.
Parameters:
truth_table: Truth table, represented as a list.
Returns:
Simplified logical expression.
"""
# Create Karnaugh map
karnaugh_map = create_karnaugh_map(truth_table)
# Identify and combine adjacent cells
groups = find_groups(karnaugh_map)
# Generate simplified logical expression
simplified_expression = generate_expression(groups)
return simplified_expression
```
**Logical Analysis:**
This code block implements the process of using a Karnaugh map to simplify a truth table. It first creates a Karnaugh map, then identifies and combines adjacent cells, and finally generates a simplified logical expression.
**Parameter Description:**
- `truth_table`: Truth table, represented as a list, where each row represents a combination of input variables, and each column represents an output variable.
**Returns:**
- `simplified_expression`: Simplified logical expression, represented as a string.
# 3. The Principle of the Quine-McCluskey Method
### 3.1 Basic Steps of the Quine-McCluskey Method
The Quine-McCluskey method is a logical simplification method that gradually obtains a simpler logical expression by merging rows or columns in the truth table. Its basic steps are as follows:
1. **Create Truth Table:** First, create a truth table based on the given logic function, where columns
0
0
相关推荐
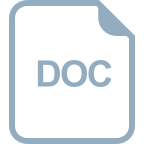
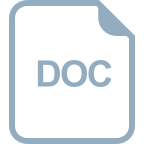
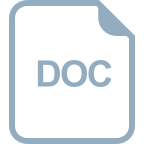
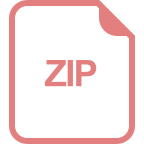
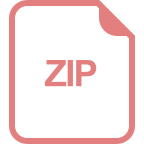
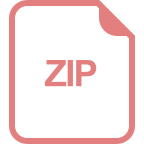
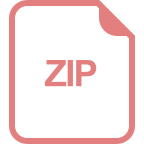
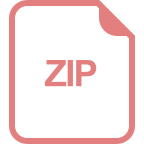
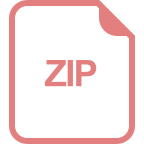