Truth Table Generator: Quickly Generate Truth Tables, Simplify Logical Analysis (Free Tool Recommendation)
发布时间: 2024-09-15 09:09:42 阅读量: 24 订阅数: 20 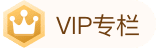
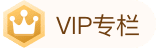
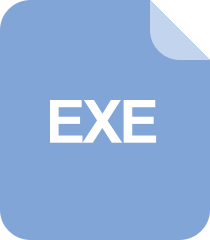
真值表生成器

# Truth Table Generator: Quickly Generate Truth Tables to Simplify Logical Analysis (Free Tool Recommendations)
## 1. Introduction to Truth Tables**
A truth table is a logical tool used to represent all possible combinations of inputs and outputs for a logical expression. It is a table where each row represents a possible combination of inputs, and each column represents a variable within a logical expression. The value in each cell of the truth table indicates the output value of the logical expression for that combination of inputs.
Truth tables are incredibly useful for understanding and analyzing logical expressions. They can help visualize how an expression changes with different inputs and determine the true or false values of the expression. Additionally, truth tables can be used to simplify logical expressions and find equivalent expressions.
## 2. Theoretical Foundations of the Truth Table Generator
### 2.1 Basic Concepts of Boolean Algebra
Boolean algebra was established by George Boole in the 19th century and provides the theoretical foundation for the truth table generator. The basic concepts of Boolean algebra include:
- **Boolean Values:** There are only two possible Boolean values: True and False.
- **Boolean Operations:** Boolean operations act on Boolean values, including:
- **AND:** The result is True if both Boolean values are True; otherwise, it is False.
- **OR:** The result is True if either of the Boolean values is True; otherwise, it is False.
- **NOT:** Inverts the Boolean value, changing True to False and False to True.
- **Boolean Expressions:** Boolean expressions consist of Boolean values, Boolean operators, and parentheses.
### 2.2 Definition and Properties of Truth Tables
A truth table is a tabular representation of a Boolean expression that shows the output values of the expression for all possible combinations of input values. Truth tables have the following properties:
- **Number of Rows:** The number of rows in a truth table equals the number of input variables.
- **Number of Columns:** The number of columns in a truth table equals the number of possible combinations of input values.
- **Output Values:** Each cell in a truth table contains a Boolean value indicating the output of the expression for that combination of input values.
### 2.3 Truth Table Generation Algorithm
The truth table generation algorithm is a systematic process used to generate the truth table of a given Boolean expression. The algorithm follows these steps:
1. **List All Possible Combinations of Input Values:** For n input variables, there are 2^n possible combinations of input values.
2. **Calculate the Output Value for Each Input Combination:** Evaluate the Boolean expression using Boolean operators to obtain the output value for each combination of inputs.
3. **Organize the Results into a Truth Table:** Organize the input combinations and output values into a table, i.e., the truth table.
**Code Block:**
```python
def generate_truth_table(expression):
"""Generate the truth table for a given Boolean expression.
Parameters:
expression: The Boolean expression for which the truth table is to be generated.
Returns:
A truth table where rows represent input combinations and columns represent output values.
"""
# List all possible combinations of input values
input_combinations = []
for i in range(2**len(expression.variables)):
input_combination = []
for variable in expression.variables:
input_combination.append(i & (1 << expression.variables.index(variable)) != 0)
input_combinations.append(input_combination)
# Calculate the output values for each input combination
output_values = []
for input_combination in input_combinations:
output_values.append(expression.evaluate(input_combination))
# Organize the res
```
0
0
相关推荐
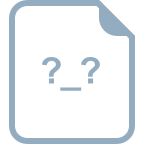
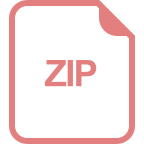
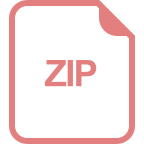
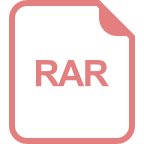
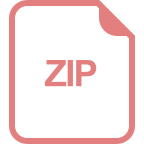
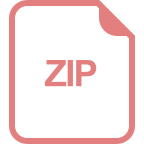
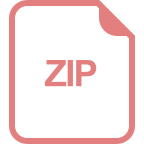