Application of Truth Tables in Computer Systems: From CPU to Memory (An Authoritative Revelation)
发布时间: 2024-09-15 09:02:41 阅读量: 20 订阅数: 20 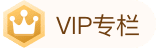
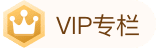
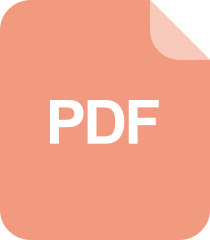
The_Computer_from_Pascal_to_von_Neumann.pdf
# Introduction to Truth Tables
A truth table is a table that describes the output values of logical operations. It lists all possible combinations of inputs and their corresponding output values. The truth table is the foundation for understanding and designing logical circuits and is widely used in computer systems.
Each row in a truth table represents a combination of inputs, while each column represents an output. For a logical operation with n inputs, the truth table will have 2^n rows. For example, a logical operation with two inputs (like AND) will have a truth table with 4 rows, as shown below:
| A | B | AND |
|---|---|---|
| 0 | 0 | 0 |
| 0 | 1 | 0 |
| 1 | 0 | 0 |
| 1 | 1 | 1 |
# Application of Truth Tables in CPUs
### 2.1 The CPU's Arithmetic Logic Unit (ALU)
A core component of the CPU is the Arithmetic Logic Unit (ALU), responsible for executing arithmetic and logical operations. The ALU uses truth tables to guide its operations. Truth tables define the output for each operation (such as AND, OR, NOT) under all possible combinations of inputs.
### 2.2 Guiding Logical Operations with Truth Tables
When the ALU executes an operation, it compares input values with the truth table to determine the output. For instance, consider a 2-input AND gate, with the following truth table:
| A | B | Output |
|---|---|---|
| 0 | 0 | 0 |
| 0 | 1 | 0 |
| 1 | 0 | 0 |
| 1 | 1 | 1 |
If the ALU receives input A=1 and B=0, it will determine the output as 0 based on the truth table.
### 2.3 Optimizing CPU Performance with Truth Tables
Truth tables can also be used to optimize CPU performance. By analyzing truth tables, operations that can be simplified or parallelized can be identified. For example, consider the following expression:
```
(A AND B) OR (NOT A AND C)
```
Using a truth table, this expression can be simplified to:
```
B OR C
```
This reduces the number of logical operations required, thereby improving CPU performance.
**Code Block:**
```python
def optimize_expression(expression):
"""Optimizes a given boolean expression.
Uses a truth table to identify operations that can be simplified.
Parameters:
expression: The boolean expression to be optimized.
Returns:
The optimized boolean expression.
"""
# Create a truth table
truth_table = truth_table_generator(expression)
# Identify operations that can be simplified
simplified_expression = ""
for row in truth_table:
if row[-1] == 1:
simplified_expression += " | " + " & ".join([var for var, value in zip(expression.split(), row[:-1]) if value == 1])
return simplified_expression[3:]
```
**Logical Analysis:**
This code block uses a truth table to optimize boolean expressions. It first creates a truth table, then iterates over each row to identify operations that can be simplified. Finally, it returns the optimized expression.
**Parameter Explanation:**
* `expression`: The boolean expression to be optimized.
# Application of Truth Tables in Memory
### 3.1 Storage Principle of Memory Units
Memory units are the basic units of data storage in computer systems. Each memory unit consists of a storage element and an address bus. The storage element
0
0
相关推荐
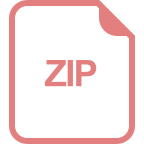
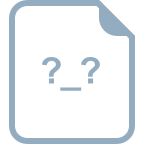
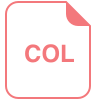
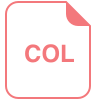
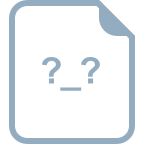
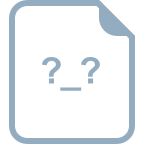
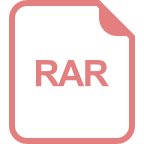
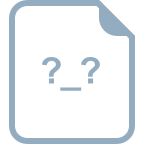