Truth Tables and Logic Circuits: Unveiling the Mysteries Behind Digital Circuits (Authoritative Analysis)
发布时间: 2024-09-15 08:57:36 阅读量: 22 订阅数: 25 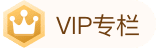
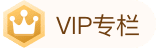
# 1. Truth Tables: The Foundation of Logic Circuits
The truth table is the bedrock of logic circuits, detailing the output of logic gates across various input combinations. A truth table is a two-dimensional chart, where:
- Rows represent all possible input combinations.
- Columns represent the outputs of the logic gate.
- Cell values are the outputs of the gate, either 0 (False) or 1 (True).
Through truth tables, we can understand the behavior of logic gates and design more complex logic circuits. For instance, the truth table for an AND gate is as follows:
| A | B | A AND B |
|---|---|---|
| 0 | 0 | 0 |
| 0 | 1 | 0 |
| 1 | 0 | 0 |
| 1 | 1 | 1 |
# 2. Implementation of Truth Tables
### 2.1 Basic Logic Gates
Logic gates are electronic circuits that implement truth tables, producing output signals based on combinations of input signals. Basic logic gates include AND, OR, and NOT gates.
#### 2.1.1 AND Gate (AND)
The AND gate has two inputs and one output. The output is true only when both inputs are true. The truth table is:
| A | B | AND |
|---|---|---|
| 0 | 0 | 0 |
| 0 | 1 | 0 |
| 1 | 0 | 0 |
| 1 | 1 | 1 |
**Code Example:**
```python
def and_gate(a, b):
"""
AND gate function
Parameters:
a (bool): Input A
b (bool): Input B
Returns:
bool: Output
"""
return a and b
**Logical Analysis:**
The `and_gate` function takes two boolean inputs `a` and `b`, and returns a boolean output. The output is true if both `a` and `b` are true; otherwise, it's false.
#### 2.1.2 OR Gate (OR)
The OR gate has two inputs and one output. The output is true when at least one input is true. The truth table is:
| A | B | OR |
|---|---|---|
| 0 | 0 | 0 |
| 0 | 1 | 1 |
| 1 | 0 | 1 |
| 1 | 1 | 1 |
**Code Example:**
```python
def or_gate(a, b):
"""
OR gate function
Parameters:
a (bool): Input A
b (bool): Input B
Returns:
bool: Output
"""
return a or b
**Logical Analysis:**
The `or_gate` function takes two boolean inputs `a` and `b`, and returns a boolean output. The output is true if at least one of `a` or `b` is true; otherwise, it's false.
#### 2.1.3 NOT Gate (NOT)
The NOT gate has one input and one output. The output is the inverse of the input. The truth table is:
| A | NOT |
|---|---|
| 0 | 1 |
| 1 | 0 |
**Code Example:**
```python
def not_gate(a):
"""
NOT gate function
Parameters:
a (bool): Input
Returns:
bool: Output
"""
return not a
**Logical Analysis:**
The `not_gate` function takes a boolean input `a`, and returns the inverse as a boolean output. The output is false if `a` is true, and true if `a` is false.
# 3.1 Combinational Logic Circuits
A combinational logic circuit is one made up of logic gates where the output is solely dependent on the current inputs, and not on the circuit'***binational logic circuits are widely used in computer systems, communication systems, and control systems.
#### 3.1.1 Adders
An adder is a combinational logic circuit used to perform addition on two or more binary numbers. The simplest adder is the half-adder, which considers only two input bits and produces a sum bit and a carry bit. A full-adder is an extension of the half-adder, considering three input bits and producing a sum bit, carry bit, and overflow bit.
**Code Block:**
```python
def half_adder(a, b):
"""
Half-adder: computes the sum and carry of two binary numbers.
Parameters:
a: First binary number
b: Second binary number
Returns:
Sum bit
Carry bit
"""
sum = a ^ b
carry = a & b
return sum, carry
def full_adder(a, b, cin):
"""
Full-adder: computes the sum, carry, and overflow of three binary numbers.
Parameters:
a: First binary number
b: Second binary number
cin: Carry input
Returns:
Sum bit
Carry bit
Overflow bit
"""
sum, carry = half_adder(a, b)
sum, overflow = half_adder(sum, cin)
carry = carry | overflow
return sum, carry, overflow
```
**Logical Analysis:**
* The `half_adder` function computes the sum and carry of two binary numbers. It uses the XOR operator (^) to compute the sum bit and the AND operator (&) to compute the carry bit.
* The `full_adder` function computes the sum, carry, and overflow of three binary numbers. It first calls the `half_adder` function to compute the sum and carry of the first two binary numbers. Then, it combines the carry result with the third binary number using a half-adder, yielding the final sum bit and carry bit. The overflow bit is the result of the AND operation between the carry bit and the final sum bit.
#### 3.1.2 Comparators
A comparator is a combinational logic circuit used to compare the magnitude of two binary numbers. The simplest comparator is an equality comparator, which only checks if the two input bits are equal. A magnitude comparator can compare the relative magnitude of two input bits and produce outputs indicating greater than, equal to, or less than.
**Code Block:**
```python
def equality_comparator(a, b):
"""
Equality comparator: checks if two binary numbers are equal.
Parameters:
a: First binary number
b: Second binary number
Returns:
True if equal, otherwise False
"""
return a == b
def magnitude_comparator(a, b):
"""
Magnitude comparator: compares the magnitude of two binary numbers.
Parameters:
a: First binary number
b: Second binary number
Returns:
1 if a > b
0 if a = b
-1 if a < b
"""
if a > b:
return 1
elif a == b:
return 0
else:
return -1
```
**Logical Analysis:**
* The `equality_comparator` function checks if two binary numbers are equal by comparing if their input bits are equal.
* The `magnitude_comparator` function compares the relative magnitude of two binary numbers by comparing their input bits. It uses conditional statements to determine the result.
# 4. Applications of Logic Circuits
Logic circuits have a wide range of applications in modern electronic systems, from computer systems to communication systems. This chapter will explore the specific applications of logic circuits in these fields.
### 4.1 Computer Systems
Logic circuits play a critical role in computer systems, responsible for processing data and controlling system operations.
#### 4.1.1 Arithmetic Logic Unit (ALU)
The Arithmetic Logic Unit (ALU) is the core component of a computer system that performs arithmetic and logical operations. It uses logic gates to implement addition, subtraction, multiplication, division, and logical operations (such as AND, OR, NOT). The ALU receives operands and an opcode, performs the corresponding operation based on the opcode, and produces a result.
```python
def alu(op, a, b):
"""
Arithmetic Logic Unit (ALU)
:param op: Opcode
:param a: Operand 1
:param b: Operand 2
:return: Result of operation
"""
if op == 'ADD':
return a + b
elif op == 'SUB':
return a - b
elif op == 'AND':
return a & b
elif op == 'OR':
return a | b
elif op == 'XOR':
return a ^ b
else:
raise ValueError("Invalid opcode")
```
**Logical Analysis:**
* The `op` parameter specifies the operation to be performed (addition, subtraction, AND, OR, XOR).
* Parameters `a` and `b` are the operands.
* The function performs the corresponding operation based on the `op` value and returns the result.
#### 4.1.2 Control Unit
The control unit is the brain of the computer system, responsible for coordinating system operations. It uses logic circuits to decode instructions, generate control signals, and manage data flow. The control unit interacts with other components (such as the ALU, registers, and memory) to implement the system's overall functionality.
### 4.2 Communication Systems
Logic circuits also play a critical role in communication systems, ensuring reliable data transmission and processing.
#### 4.2.1 Data Encoding
Data encoding is the process of converting digital data into a format suitable for transmission. Logic circuits are used to implement various encoding schemes, such as binary encoding, Manchester encoding, and NRZ encoding. These schemes use logic gates to generate specific signal patterns representing different data bits.
#### 4.2.2 Data Transmission
Data transmission involves transferring data from one location to another. Logic circuits are used to implement data transmission protocols, such as serial communication protocols and parallel communication protocols. These protocols use logic gates to control data flow, synchronize clock signals, and detect transmission errors.
**Flowchart: Serial Communication Protocol**
```mermaid
sequenceDiagram
participant Sender
participant Receiver
Sender->Receiver: Start bit
Receiver->Sender: Ack
Sender->Receiver: Data bit 1
Receiver->Sender: Ack
Sender->Receiver: Data bit 2
Receiver->Sender: Ack
Sender->Receiver: Stop bit
Receiver->Sender: Ack
```
**Logical Analysis:**
* The sender transmits a start bit, indicating the beginning of data transmission.
* The receiver sends an acknowledgment signal (Ack), indicating readiness to receive data.
* The sender transmits data bits, one at a time.
* The receiver sends an acknowledgment after receiving each data bit.
* The sender transmits a stop bit, indicating the end of data transmission.
# 5.1 Types of Failures
Logic circuit failures are typically classified into two categories:
### 5.1.1 Short-Circuit Failures
A short-circuit failure occurs when there's an unintended conductive path between two points that should not be connected, causing abnormal current flow. Short-circuit failures are usually caused by:
- Damaged wire insulation
- Solder bridges between component leads
- Internal short circuits within components
Short-circuit failures can lead to excessive current in the circuit, potentially causing component damage or circuit board failure.
### 5.1.2 Open-Circuit Failures
An open-circuit failure occurs when there's a break in the path between two points that should be connected, preventing normal current flow. Open-circuit failures are usually caused by:
- Wire breaks
- Solder joint failures on component leads
- Internal open circuits within components
Open-circuit failures result in an interruption of current in the circuit, which may cause the circuit to malfunction.
0
0
相关推荐








