Practical Application of Truth Tables: Mastering the Essence of Logical Design from Theory to Practice (5 Real Cases)
发布时间: 2024-09-15 08:59:13 阅读量: 16 订阅数: 17 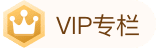
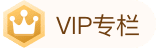
# Practical Application of Truth Tables: From Theory to Practice, Mastering the Essence of Logical Design (5 Real-World Cases)
# 1. The Basics of Truth Tables
A truth table is a tabular representation of logical operations that shows all possible combinations of inputs and their corresponding output values. Truth tables are crucial for understanding and designing logical circuits as they offer a visual representation of logical operations.
**Composition of a Truth Table:**
- **Input Variables:** The first row of a truth table represents the input variables, each of which can take a true (1) or false (0) value.
- **Output Variable:** The last column of a truth table represents the output variable, which is calculated based on the values of the input variables.
- **Truth Table Entries:** Each row in a truth table is referred to as a truth table entry, representing a specific combination of input variables and their corresponding output value.
**Uses of Truth Tables:**
Truth tables are used for:
- **Analyzing Logical Operations:** Truth tables help visualize logical operations and analyze their behavior.
- **Designing Logical Circuits:** Truth tables can serve as the foundation for designing logical circuits, as they provide a systematic method to determine the circuit's output.
- **Verifying Logical Circuits:** Truth tables can be used to verify the correctness of logical circuits by comparing actual outputs with the expected outputs listed in the table.
# 2. Practical Design of Truth Tables
### 2.1 Logical Gate Design
#### 2.1.1 AND Gates, OR Gates, NOT Gates
**AND Gate**
Truth Table:
| A | B | A AND B |
|---|---|---|
| 0 | 0 | 0 |
| 0 | 1 | 0 |
| 1 | 0 | 0 |
| 1 | 1 | 1 |
An AND gate outputs true only when all inputs are true.
**OR Gate**
Truth Table:
| A | B | A OR B |
|---|---|---|
| 0 | 0 | 0 |
| 0 | 1 | 1 |
| 1 | 0 | 1 |
| 1 | 1 | 1 |
An OR gate outputs true when at least one input is true.
**NOT Gate**
Truth Table:
| A | NOT A |
|---|---|
| 0 | 1 |
| 1 | 0 |
A NOT gate inverts the input, changing true to false and vice versa.
#### 2.1.2 XOR Gates, XNOR Gates
**XOR Gate**
Truth Table:
| A | B | A XOR B |
|---|---|---|
| 0 | 0 | 0 |
| 0 | 1 | 1 |
| 1 | 0 | 1 |
| 1 | 1 | 0 |
An XOR gate outputs true when the inputs are different.
**XNOR Gate**
Truth Table:
| A | B | A XNOR B |
|---|---|---|
| 0 | 0 | 1 |
| 0 | 1 | 0 |
| 1 | 0 | 0 |
| 1 | 1 | 1 |
An XNOR gate outputs true when the inputs are the same.
### 2.2 Design of Combinational Logic Circuits
The output of a combinational logic circuit depends solely on the current inputs and is independent of the circuit's past state.
#### 2.2.1 Half Adder Design
A half adder is a circuit that adds two binary numbers, producing a sum and a carry.
Truth Table:
| A | B | SUM | CARRY |
|---|---|---|---|
| 0 | 0 | 0 | 0 |
| 0 | 1 | 1 | 0 |
| 1 | 0 | 1 | 0 |
| 1 | 1 | 0 | 1 |
```verilog
module half_adder(
input A,
input B,
output SUM,
output CARRY
);
assign SUM = A ^ B;
assign CARRY = A & B;
endmodule
```
Logical Analysis:
* SUM is the exclusive OR (XOR) result of A and B, representing the sum.
* CARRY is the AND result of A and B, representing the carry.
#### 2.2.2 Full Adder Design
A full adder is a circuit that adds three binary numbers, producing a sum and a carry.
Truth Table:
| A | B | CIN | SUM | CARRY |
|---|---|---|---|---|
| 0 | 0 | 0 | 0 | 0 |
| 0 | 0 | 1 | 1 | 0 |
| 0 | 1 | 0 | 1 | 0 |
| 0 | 1 | 1 | 0 | 1 |
| 1 | 0 | 0 | 1 | 0 |
| 1 | 0 | 1 | 0 | 1 |
| 1 | 1 | 0 | 0 | 1 |
| 1 | 1 | 1 | 1 | 1 |
```verilog
module full_adder(
input A,
input B,
input CIN,
output SUM,
output CARRY
);
assign SUM = A ^ B ^ CIN;
assign CARRY = (A & B) | (B & CIN) | (A & CIN);
endmodule
```
Logical Analysis:
* SUM is the XOR result of A, B, and CIN, representing the sum.
* CARRY is the OR result of A and B with CIN, representing the carry.
### 2.3 Design of Sequential Logic Circuits
The output of a sequential logic circuit depends not only on the current inputs but also on the past state of the circuit.
#### 2.3.1 RS Flip-Flop Design
An RS flip-flop is a sequential logic circuit with two inputs (R and S) and two outputs (Q and Q').
Truth Table:
| R | S | Q | Q' |
|---|---|---|---|
| 0 | 0 | Hold | Hold |
| 0 | 1 | 0 | 1 |
| 1 | 0 | 1 | 0 |
| 1 | 1 | X | X |
```verilog
module rs_latch(
input R,
input S,
output Q,
output Q_NOT
);
always @(R, S) begin
if (R == 1) begin
Q <= 0;
Q_NOT <= 1;
end else if (S == 1) begin
Q <= 1;
Q_NOT <= 0;
end
end
endmodule
```
Logical Analysis:
* When R is 1, Q outputs 0, Q' outputs 1, resetting the flip-flop.
* When S is 1, Q outputs 1, Q' outputs 0, setting the flip-flop.
* When both R and S are 0, the flip-flop holds its current state.
* When both R and S are 1, the flip-flop enters an unstable state with unpredictable outputs.
#### 2.3.2 JK Flip-Flop Design
A JK flip-flop is a sequential logic circuit with two inputs (J and K) and two outputs (Q and Q').
Truth Table:
| J | K | Q | Q' |
|---|---|---|---|
| 0 | 0 | Hold | Hold |
| 0 | 1 | 0 | 1 |
| 1 | 0 | 1 | 0 |
| 1 | 1 | Q' | Q |
```verilog
module jk_flipflop(
input J,
input K,
input CLK,
output Q,
output Q_NOT
);
always @(posedge CLK) begin
if (J == 1 && K == 0) begin
Q <= 1;
Q_NOT <= 0;
end else if (J == 0 && K == 1) begin
Q <= 0;
Q_NOT <= 1;
end else if (J == 1 && K == 1) begin
Q <= ~Q;
Q_NOT <= ~Q_NOT;
end
end
endmodule
```
Logical Analysis:
* When J is 1 and K is 0, Q outputs 1, Q' outputs 0, setting the flip-flop.
* When J is 0 and K is 1, Q outputs 0, Q' outputs 1, re
0
0
相关推荐
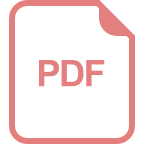
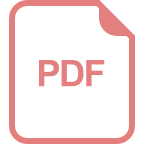
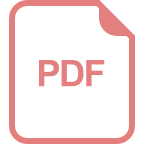
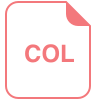
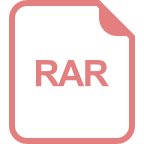
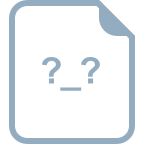
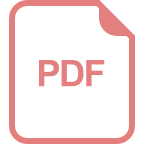
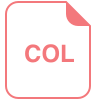
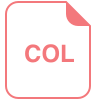
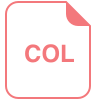