【Fundamentals】In-depth Exploration of Random Number Generation and Statistical Analysis in MATLAB
发布时间: 2024-09-13 22:53:17 阅读量: 18 订阅数: 38 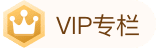
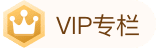
**1. Random Number Generation in MATLAB**
MATLAB offers a variety of pseudo-random number generators (PRNGs) that produce deterministic sequences of numbers that appear to be random. The most commonly used PRNG is the `rand` function, which generates uniformly distributed random numbers in the range [0,1].
```
% Generate 10 uniformly distributed random numbers
rand_numbers = rand(1, 10);
```
MATLAB also provides functions for generating random numbers with other distributions, such as `randn` (normal distribution), `randperm` (permutation), and `poissrnd` (Poisson distribution).
**2. Practical Random Number Generation**
### 2.1 Generation of Random Variables
#### 2.1.1 Discrete Random Variables
**Generating Binomial Distributed Random Variables**
```matlab
n = 10; % Number of trials
p = 0.5; % Probability of success
x = binornd(n, p, 1000); % Generate 1000 binomially distributed random variables
```
**Logical Analysis:**
The `binornd` function is used to generate binomially distributed random variables. It accepts three parameters:
* `n`: Number of trials
* `p`: Probability of success
* `size`: Number of random variables to generate
The function returns a vector of random variables of the specified size.
**Generating Poisson Distributed Random Variables**
```matlab
lambda = 5; % Average rate of occurrence
x = poissrnd(lambda, 1000); % Generate 1000 Poisson distributed random variables
```
**Logical Analysis:**
The `poissrnd` function is used to generate Poisson distributed random variables. It accepts two parameters:
* `lambda`: Average rate of occurrence
* `size`: Number of random variables to generate
The function returns a vector of random variables of the specified size.
#### 2.1.2 Continuous Random Variables
**Generating Uniformly Distributed Random Variables**
```matlab
a = 0; % Lower bound
b = 10; % Upper bound
x = unifrnd(a, b, 1000); % Generate 1000 uniformly distributed random variables
```
**Logical Analysis:**
The `unifrnd` function is used to generate uniformly distributed random variables. It accepts three parameters:
* `a`: Lower bound
* `b`: Upper bound
* `size`: Number of random variables to generate
The function returns a vector of random variables of the specified size.
**Generating Normally Distributed Random Variables**
```matlab
mu = 0; % Mean
sigma = 1; % Standard deviation
x = normrnd(mu, sigma, 1000); % Generate 1000 normally distributed random variables
```
**Logical Analysis:**
The `normrnd` function is used to generate normally distributed random variables. It accepts three parameters:
* `mu`: Mean
* `sigma`: Standard deviation
* `size`: Number of random variables to generate
The function returns a vector of random variables of the specified size.
### 2.2 Generation of Random Sequences
#### 2.2.1 White Noise
**Generating White Noise Sequence**
```matlab
N = 1000; % Sequence length
x = randn(1, N); % Generate 1000 white noise samples
```
**Logical Analysis:**
The `randn` function is used to generate normally distributed random numbers. It accepts one parameter:
* `size`: Dimensionality of the generated random numbers
The function returns a matrix of random numbers of the specified size.
#### 2.2.2 Brownian Motion
**Generating Brownian Motion Sequence**
```matlab
N = 1000; % Sequence length
dt = 0.01; % Time step
sigma = 0.1; % Standard deviation
x = zeros(1, N);
for i = 2:N
x(i) = x(i-1) + sqrt(dt) * normrnd(0, sigma);
end
```
**Logical Analysis:**
Brownian motion is a stochastic process where the increments are normally distributed. This code uses the Euler-Maruyama method to generate the sequence of Brownian motion.
* `zeros(1, N)` creates a zero vector of length `N`.
* The loop starts at `i = 2` since the initial value of Brownian motion is usually zero.
* In each iteration, `x(i)` is updated to the previous value `x(i-1)` plus the square root of the time step `dt` multiplied by a normally distributed random number `normrnd(0, sigma)`.
The code generates a Brownian motion sequence of length `N`, stored in the vector `x`.
**3. Descriptive Statistics**
#### 3.1 Me***
***mon measures of central tendency include:
***Mean (Average):** The sum of all data values di
0
0
相关推荐
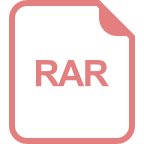
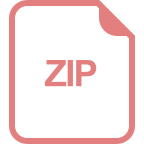
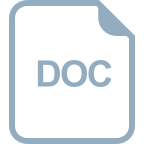
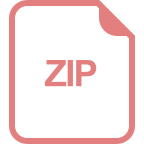
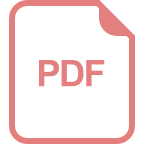
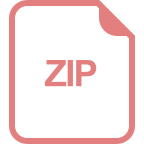
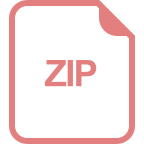
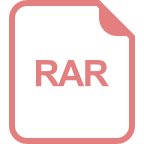