【Advanced Chapter】Finite Element Method: Theory and MATLAB Implementation
发布时间: 2024-09-13 23:41:12 阅读量: 21 订阅数: 38 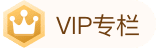
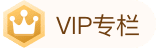
# Advanced Chapter: Finite Element Method: Theory and MATLAB Implementation
## 2.1 Solving Finite Element Equations
The solution of finite element equations is a critical step in the finite element method, ***mon solution methods include direct and iterative methods.
### 2.1.1 Direct Metho*
***mon direct methods include Gaussian elimination and LU decomposition. The advantage of direct methods is high solution accuracy and fast convergence. However, for large-scale finite element models, direct methods require substantial computational resources, and the computational complexity increases sharply with the size of the model.
### 2.1.***
***mon iterative methods include the Jacobi method, Gauss-Seidel method, and Conjugate Gradient method. The advantage of iterative methods is that they require fewer computational resources, and the convergence speed for large models is unaffected. However, the accuracy and convergence speed of iterative methods depend on the number of iterations and the choice of iterative algorithms.
## 2. Implementation of the Finite Element Method in MATLAB
### 2.1 Solving Finite Element Equations
The core of the finite element method lies in solving the partial differential equations that describe physical problems. In MATLAB, direct or iterative methods can be used to solve finite element equations.
#### ***
***mon direct methods in MATLAB include Gaussian elimination and Cholesky decomposition.
**Gaussian Elimination**
```matlab
% Stiffness matrix
K = [2 -1 0; -1 2 -1; 0 -1 1];
% Load vector
F = [1; 0; 0];
% Solve for unknown displacements
U = K \ F;
```
**Logical Analysis:**
* Gaussian elimination converts the stiffness matrix K into an upper triangular matrix, and then the unknown displacements U are solved by back substitution.
* In MATLAB, the backslash operator (\) is used to solve linear systems, where K\F represents solving the system K*U = F.
**Cholesky Decomposition**
```matlab
% Stiffness matrix
K = [2 -1 0; -1 2 -1; 0 -1 1];
% Cholesky decomposition
[L, U] = chol(K);
% Solve for unknown displacements
Y = L \ F;
X = U \ Y;
```
**Logical Analysis:**
* Cholesky decomposition decomposes the stiffness matrix K into the product of a lower triangular matrix L and an upper triangular matrix U.
* To solve for the unknown displacements, the load vector F is first decomposed into Y, and then X is solved.
* Cholesky decomposition is more stable than Gaussian elimination but more computationally intensive.
#### ***
***mon iterative methods in MATLAB include the Jacobi method and Conjugate Gradient method.
**Jacobi Iterative Method**
```matlab
% Stiffness matrix
K = [2 -1 0; -1 2 -1; 0 -1 1];
% Load vector
F = [1; 0; 0];
% Initial guess
U0 = zeros(size(F));
% Number of iterations
maxIter = 100;
% Iterative solution
for i = 1:maxIter
for j = 1:size(K, 1)
U0(j) = (F(j) - K(j, :) * U0) / K(j, j);
end
end
```
**Logical Analysis:**
* The Jacobi iterative method successively updates the unknown displacements row by row until convergence conditions are met.
* MATLAB uses a for loop to implement the iteration process.
**Conjugate Gradient Method**
```matlab
% Stiffness matrix
K = [2 -1 0; -1 2 -1; 0 -1 1];
% Load vector
F = [1; 0; 0];
% Initial guess
U0 = zeros(size(F));
% Number of iterations
maxIter = 100;
% Conjugate gradient method solution
[U, flag] = pcg(K, F, 1e-6, maxIter);
```
**Logical Analysis:**
* The Conjugate Gradient method is a more efficient iterative method, which uses conjugate gradient directions to accelerate convergence.
* In MATLAB, the pcg function is used to solve the conjugate gradient system, where K is the stiffness matrix, F is the load vector, 1e-6 is the convergence tolerance, and maxIter is the maximum number of iterations.
## 3.1 Structural Mechanics Analysis
The finite element method is widely used in structural mechanics analysis and can solve various complex structural problems, such as the bending of beams and the vibration of plates.
#### 3.1.1 Bending of Beams
Beam bending analysis is a common problem in structural mechanics. The finite element method can accurately calculate the deformation and internal forces of beams.
```matlab
% Beam geometric parameters
L = 1; % Beam length
b = 0.1; % Beam width
h = 0.2; % Beam height
E = 200e9; % Young's modulus
I = b*h^3/12; % Moment of inertia
% Apply boundary conditions
fixed_end = 1; % Fixed end
free_end = 2; % Free end
u_fixed = 0; % Displacement at the fixed end
theta_fixed = 0; % Rotation at the fixed end
P = 1000; % Concentrated force magnitude
% Mesh generation
n_elements = 10; % Number of elements
x = linspace(0, L, n_elements+1); % Node coordinates
% Assemble stiffness matrix and load vector
K = zeros(2*(n_elements+1)); % Stiffness matrix
F = zeros(2*(n_elements+1), 1); % Load vector
for i = 1:n_elements
% Element stiffness matrix
k = [E*I/L^3, -E*I/L^2, -E*I/L^3, E*I/L^2;
-E*I/L^2, 4*E*I/L^3, E*I/L^2, -2*E*I/L^3;
-E*I/L^3, E*I/L^2, E*I/L^3, -E*I/L^2;
```
0
0
相关推荐
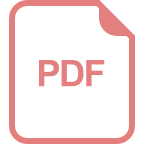
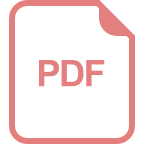
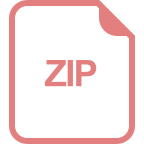





