[Advanced] Implementation of Hidden Markov Model (HMM) in MATLAB
发布时间: 2024-09-13 23:25:40 阅读量: 37 订阅数: 52 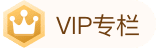
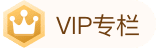
# 2.1 Creation and Initialization of the HMM Model
### 2.1.1 Definition of State Transition and Observation Matrices
The Hidden Markov Model (HMM) is defined by two key matrices: the state transition matrix and the observation matrix.
The state transition matrix `A` defines the probability of the system transitioning from one state to another. It is an `N x N` matrix where `N` is the number of states in the model. `A(i, j)` represents the probability of the system transitioning from state `i` to state `j`.
The observation matrix `B` defines the probability of the system producing an observation given a certain state. It is an `N x M` matrix where `M` is the number of observations. `B(i, k)` represents the probability of the system producing observation `k` while in state `i`.
### 2.1.2 Setting the Initial State Probabilities
The initial state probabilities `π` is a vector with `N` dimensions, where `π(i)` represents the probability of the system starting in state `i`. It is typically set through a uniform distribution or based on prior knowledge.
# 2. Tips for Implementing HMM in MATLAB
### 2.1 Creation and Initialization of the HMM Model
#### 2.1.1 Definition of State Transition and Observation Matrices
When creating an HMM model in MATLAB, it is first necessary to define the state transition matrix and the observation matrix. The state transition matrix specifies the probability of transitioning from one state to another, while the observation matrix specifies the probability of observing a particular symbol given a certain state.
```matlab
% Defining the state transition matrix
A = [0.5, 0.5; 0.2, 0.8];
% Defining the observation matrix
B = [0.6, 0.4; 0.3, 0.7];
```
**Parameter Description:**
* `A`: State transition matrix with size `n x n`, where `n` is the number of states.
* `B`: Observation matrix with size `n x m`, where `m` is the number of observation symbols.
**Code Logic:**
1. In matrix `A`, the first row represents the transition probabilities for state 1, and the second row represents the transition probabilities for state 2.
2. In matrix `B`, the first row represents the probability of observing symbol 1 while in state 1, and the second row represents the probability of observing symbol 2 while in state 1.
#### 2.1.2 Setting the Initial State Probabilities
In addition to the state transition matrix and the observation matrix, it is also necessary to set the initial state probabilities, which represent the probabilities of being in each state at time step 0.
```matlab
% Defining the initial state probabilities
pi = [0.5, 0.5];
```
**Parameter Description:**
* `pi`: Initial state probability vector with size `1 x n`.
**Code Logic:**
1. In the `pi` vector, the first element represents the initial probability of state 1, and the second element represents the initial probability of state 2.
### 2.2 Training and Evaluating the HMM Model
#### 2.2.1 Forward-Backward Algorithm
The forward-backward algorithm is used to calculate the probabilities of each state in the HMM model given a sequence of observations.
```matlab
% Forward-Backward algorithm
[alpha, beta] = hmmdecode(A, B, pi, obs);
```
**Parameter Description:**
* `A`: State transition matrix.
* `B`: Observation matrix.
* `pi`: Initial state probability vector.
* `obs`: Sequence of observations.
**Code Logic:**
1. The `hmmdecode` function uses the forward-backward algorithm to compute the probabilities of each state given the sequence of observations.
2. The `alpha` matrix stores forward probabilities, while the `beta` matrix stores backward probabilities.
#### 2.2.2 Baum-Welch Algorithm
The Baum-Welch algorithm is used to train the parameters of the HMM model (state transition matrix, observation matrix, and initial st
0
0
相关推荐







