【Advanced】Implementing PSO Algorithm with Matlab
发布时间: 2024-09-13 23:18:15 阅读量: 40 订阅数: 38 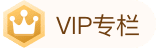
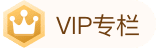
# Advanced篇: Implementing PSO Algorithm in Matlab
## 2.1 Mathematical Model of PSO Algorithm in Matlab
### 2.1.1 Algorithm Principle
Particle Swarm Optimization (PSO) is a heuristic optimization technique inspired by the social behavior of birds. In PSO, each particle represents a potential solution, and the swarm represents a set of all potential solutions. Particles search for the optimal solution by continuously updating their position and velocity.
The formulae for updating the velocity and position of particles are as follows:
```matlab
v(i+1) = w * v(i) + c1 * r1 * (pBest(i) - x(i)) + c2 * r2 * (gBest - x(i))
x(i+1) = x(i) + v(i+1)
```
Where:
- `v(i)` denotes the velocity of the `i`-th particle.
- `x(i)` denotes the position of the `i`-th particle.
- `w` denotes the inertia weight.
- `c1` and `c2` denote the cognitive and social coefficients, respectively.
- `r1` and `r2` denote random numbers in the range [0, 1].
- `pBest(i)` denotes the personal best position of the `i`-th particle.
- `gBest` denotes the global best position among the entire swarm.
## 2. Implementation Fundamentals of PSO Algorithm in Matlab
### 2.1 Mathematical Model of PSO Algorithm in Matlab
#### 2.1.1 Algorithm Principle
Particle Swarm Optimization (PSO) is a heuristic optimization technique that is inspired by the social behavior of birds. In PSO, each particle represents a potential solution, and the swarm collectively searches for the optimal solution.
The mathematical model of the PSO algorithm is as follows:
```
v_i(t+1) = w * v_i(t) + c1 * r1 * (pbest_i(t) - x_i(t)) + c2 * r2 * (gbest(t) - x_i(t))
x_i(t+1) = x_i(t) + v_i(t+1)
```
Where:
* `v_i(t)` is the velocity of particle `i` at time `t`
* `w` is the inertia weight, controlling the variation of particle velocity
* `c1` and `c2` are the cognitive and social coefficients, respectively, controlling the extent of learning from the particle's own best solution and the swarm's best solution
* `r1` and `r2` are uniformly distributed random numbers in the range [0, 1]
* `pbest_i(t)` is the personal best solution of particle `i` at time `t`
* `gbest(t)` is the global best solution of the swarm at time `t`
* `x_i(t)` is the position of particle `i` at time `t`
#### 2.1.2 Algorithm Parameters
The key parameters of the PSO algorithm include:
| Parameter | Meaning | Range |
|---|---|---|
| `w` | Inertia weight | [0, 1] |
| `c1` | Cognitive factor | [0, 2] |
| `c2` | Social factor | [0, 2] |
| `r1` and `r2` | Random numbers | [0, 1] |
| `max_iter` | Maximum number of iterations | Positive integer |
| `pop_size` | Swarm size | Positive integer |
### 2.2 Code Framework of PSO Algorithm in Matlab
#### 2.2.1 Algorithm Initialization
```matlab
% Initialize parameters
w = 0.729;
c1 = 1.49445;
c2 = 1.49445;
max_iter = 100;
pop_size = 30;
% Initialize the swarm
particles = zeros(pop_size, d);
for i = 1:pop_size
particles(i, :) = lb + (ub - lb) .* rand(1, d);
end
% Initialize the personal bests and global best
pbest = particles;
gbest = min(particles);
```
#### 2.2.2 Algorithm Iteration
```matlab
% Iterative optimization
for iter = 1:max_iter
% Update particle velocity and position
for i = 1:pop_size
v_i = w * v_i + c1 * r1 * (pbest_i - x_i) + c2 * r2 * (gbest - x_i);
x_i = x_i + v_i;
end
% Update personal bests and global best
for i = 1:pop_size
if f(x_i) < f(pbest_i)
pbest_i = x_i;
end
end
gbest = min(pbest);
end
```
#### 2.2.3 Convergence Judgment
```matlab
% Determine if the algorithm has converged
if abs(gbest(iter) - gbest(iter-1)) < tol
break;
end
```
# 3. Practical Application of PSO Algorithm in Matlab
### 3.1 Solving Classic Functions with PSO Algorithm in Matlab
In this section, we will use the PSO algorithm in Matlab to solve two classic functions: the Rosenbrock function and the Rastrigin function. These functions are typically used to test the performance of optimization algorithms.
#### 3.1.1 Rosenbrock Function
The Rosenbrock function is a non-convex function with the following mathematical expression:
```
f(x, y) = 100 * (y - x^2)^2 + (1 - x)^2
```
Where `x` and `y` are the independent variables.
**Code Block:**
```matlab
% Parameter settings
num_particles = 100; % Number of particles
max_iter = 100; % Maximum number of iterations
c1 = 2; % Cognitive factor
c2 = 2; % Social factor
w = 0.5; % Inertia weight
% Initialize the swarm
particles = rand(num_particles, 2) * 10 - 5; % Particle positions range [-5, 5]
velocities = zeros(num_particles, 2); % Particle velocities
% Iterative optimization
for iter = 1:max_iter
% Calculate fitness values
fitness = 100 * (particles(:, 2) - particles(:, 1).^2).^2 + (1 - particles(:, 1)).^2;
% Update personal best positions and velocities
for i = 1:num_particles
if fitness(i) < particles(i, 3)
particles(i, 3:4) = particles(i, 1:2);
end
end
% Update global best position
[~, best_idx] = min(fitness);
gbest = particles(best_idx, :);
% Update particle velocities and positions
for i = 1:num_particles
velocities(i, :) = w * velocities(i, :) + c1 * rand(1, 2) .* (particles(i, 3:4) - particles(i, 1:2)) + c2 * rand(1, 2) .* (gbest(1:2) - particles(i, 1:2));
particles(i, 1:2) = particles(i, 1:2) + velocities(i, :);
end
end
% Output the optimal solution
disp(['Optimal solution: ', num2str(gbest(1:2))]);
disp(['Optimal fitness value: ', num2str(gbest(3))]);
```
**Logical Analysis:**
* Initialize the swarm, including particle positions and velocities.
* Iterative optimization, including calculating fitness values, updating personal best positions and velocities, updating the global best position, and updating particle velocities and positions.
* Output the optimal solution and the optimal fitness
0
0
相关推荐
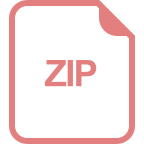
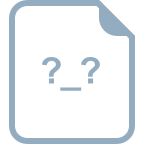
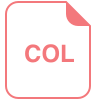
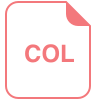
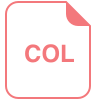
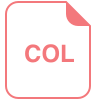
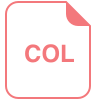
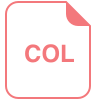