MATLAB Simulated Annealing Algorithm: Solutions for Complex Optimization Problems
发布时间: 2024-09-14 20:49:00 阅读量: 43 订阅数: 32 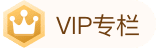
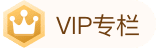
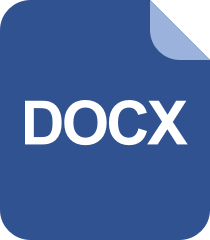
MATLAB中退火算法(Simulated Annealing)实现与应用教程
# 1. An Overview of the Simulated Annealing Algorithm
In exploring the solution space of optimization problems, the Simulated Annealing (SA) algorithm has proven its worth across various fields due to its powerful global search capabilities. Inspired by the physical annealing process, this algorithm simulates the heating and cooling crystallization process of solid matter, accepting solutions in a probabilistic manner. SA can escape local optima in the solution space, increasing the likelihood of finding the global optimum. In this chapter, we will introduce the basic concepts, origins, and application prospects of the simulated annealing algorithm in various optimization problems. By understanding this algorithm, we can provide new perspectives and solutions for solving complex engineering optimization problems.
# 2. Implementing Simulated Annealing in MATLAB
## 2.1 MATLAB Basics and Data Structures
### 2.1.1 Introduction to the MATLAB Environment
MATLAB (an abbreviation for Matrix Laboratory) is a high-performance numerical computing and visualization software developed by MathWorks. It is widely used in fields such as engineering computation, data analysis, algorithm development, and simulation. MATLAB provides an interactive environment with a vast number of built-in functions and toolboxes that cover areas ranging from signal processing, image processing to deep learning.
The fundamental unit in MATLAB is the matrix, making it highly efficient for dealing with linear algebra and multi-dimensional data structures. Users can perform complex mathematical operations and data analysis tasks through concise commands and function calls. MATLAB scripts and function files are denoted by the `.m` extension and can be organized into larger project structures such as project files (`*.prj`) or simulation models (`*.slx`).
### 2.1.2 Data Types and Matrix Operations
MATLAB supports various data types, including integers, floating-point numbers, characters, strings, logical values, as well as more complex structures like structs and cell arrays. These data types allow users to flexibly process different types of data and information. The matrix is the core data structure in MATLAB, and almost all operations can be represented as matrix operations, greatly simplifying the code.
Matrix operations in MATLAB are very intuitive. For instance, matrices can be created using square brackets, and matrix operations follow the rules of linear algebra. MATLAB also offers numerous built-in functions to handle matrices, including but not limited to matrix multiplication (`*`), matrix division (`\`), transpose (`'`), inverse (`inv`), determinant (`det`), as well as eigenvalues and eigenvectors (`eig`).
## 2.2 Principles of the Simulated Annealing Algorithm
### 2.2.1 Basic Concepts of the Algorithm
Simulated Annealing (SA) is a stochastic search algorithm used to find the optimal solution in a given large search space. Its inspiration comes from the annealing process of solids, where a solid is heated and then slowly cooled. During this process, the atoms in the solid will gradually reach the lowest energy state, that is, the most stable structure, as the temperature decreases.
In the algorithm, the system is simulated as an energy state, and the search process attempts to find the state with the least energy (i.e., the lowest cost). At the beginning of the algorithm, a high "temperature" parameter is set, and random perturbations (i.e., the probability of accepting new solutions) are used to explore the solution space. As the temperature gradually decreases, the probability of accepting new solutions also decreases, thus causing the system to gradually trend towards a stable state, which is a local or global minimum.
### 2.2.2 Temperature Scheduling Strategies
Temperature scheduling strategy is one of the most crucial parts of the simulated annealing algorithm, as it determines the performance and convergence speed of the algorithm. Temperature scheduling typically includes setting the initial temperature, determining the cooling rate, and setting the final temperature.
The initial temperature must be high enough to ensure that the system can escape local minima at the beginning and explore a sufficiently large solution space. The cooling rate determines the rate at which temperature drops; too fast may cause the algorithm to converge prematurely to a local minimum, while too slow may increase the running time of the algorithm. The final temperature is usually set to a very small positive number, and the algorithm terminates when the temperature drops below this value.
### 2.2.3 Acceptance Criteria
The acceptance criteria define the rules for accepting a new solution as the current solution when the current solution is not optimal. In the simulated annealing algorithm, the most commonly used acceptance criterion is the Metropolis criterion, which is expressed as:
\[ P(\Delta E, T) =
\begin{cases}
1 & \text{if } \Delta E < 0 \\
e^{\frac{-\Delta E}{T}} & \text{if } \Delta E \geq 0
\end{cases}
\]
where, \(\Delta E\) is the energy difference (cost difference) between the new solution and the current solution, and \(T\) is the current temperature parameter. According to the Metropolis criterion, a new solution is unconditionally accepted only if its energy is lower (i.e., the cost is lower). When the new solution has a higher energy (i.e., the cost is higher), the new solution is accepted with a certain probability, which increases with the temperature.
## 2.3 Implementation of the Algorithm in MATLAB
### 2.3.1 MATLAB Code Framework
Implementing the simulated annealing algorithm in MATLAB typically involves writing a main function that contains the core steps of the simulated annealing algorithm. Below is a simplified MATLAB code framework that demonstrates the basic structure of the algorithm:
```matlab
function [best_solution, best_cost] = simulated_annealing(initial_solution)
% Initialize parameters
current_solution = initial_solution;
best_solution = current_solution;
current_cost = cost_function(current_solution);
best_cost = current_cost;
% Set initial temperature and cooling rate
T = initial_temperature;
cooling_rate = ...;
% Start the simulated annealing process
while T > final_temperature
% Generate a new candidate solution
new_solution = perturb(current_solution);
new_cost = cost_function(new_solution);
% Decide whether to accept the new solution based on acceptance criteria
if accept_new_solution(new_cost, current_cost, T)
current_solution = new_solution;
current_cost = new_cost;
% Update the best solution
if new_cost < best_cost
best_solution = new_solution;
best_cost = new_cost;
end
end
% Decrease the temperature
T = T * (1 - cooling_rate);
end
end
```
In this framework, `cost_function` is a function used to calculate the cost of a solution, `perturb` is used to generate neighboring solutions, and `accept_new_solution` implements the acceptance criteria. This framework can be expanded and modified to suit different types of optimization problems.
### 2.3.2 Parameter Configuration and Optimization
To effectively apply the simulated annealing algorithm, careful configuration and optimization of the algorithm's parameters are required. These include initial temperature, cooling rate, final temperature, perturbation strategy, and acceptance criteria. In MATLAB, these parameters can be defined as function input parameters, allowing users to adjust them according to the specific problem at hand.
Parameter configuration typically depends on the specific problem and experience and can be determined by trial and error. In addition, adaptive methods can be used to dynamically adjust parameters, such as adjusting temperature or cooling rate based on the current search state, making the algorithm more flexible and effective.
In this process, MATLAB's plotting functions can be used to monitor the performance of the algorithm, such as plotting the relationship between cost and iteration number or cost and temperature. Such visualization can help users better understand the convergence behavior of the algorithm and adjust parameters to obtain better results.
```matlab
% Plot the cost change graph
figure;
plot(cost_history);
xlabel('Iteration');
ylabel('Cost');
title('Cost vs. Iteration');
```
This code will plot the trend of cost changes with the number of iterations, helping users assess the algorithm's performance and adjust parameters accordingly. With appropriate parameter configuration and optimization, the simulated annealing algorithm in MATLAB can effectively solve various complex optimization problems.
# 3. Applications of the Simulated Annealing Algorithm in Optimization Problems
## 3.1 Function Optimization
### 3.1.1 Single-Peak and Multi-Peak Function Optimization
Function optimization is one of the most basic applications of the simulated annealing algorithm, mainly involving the optimization of single-peak functions and multi-peak functions. A single-peak function has only one local optimum, while a multi-peak function has multiple local optima. The simulated annealing algorithm reduces the risk of falling into local optima during the search for the global optimum by controlling the "temperature" parameter.
In single-peak function optimization, the simulated annealing algorithm can quickly converge to the global optimum because the path in the solution space is relatively simple. However, in multi-peak function optimization, avoiding falling into local optima and exploring the global optimum is the key problem the algorithm needs to solve.
### 3.1.2 MATLAB Function Optimization Examples
Below is an example code for implementing single-peak and multi-peak function optimization using MATLAB:
```matlab
% Single-peak function optimization example: Rosenbrock function
x0 = [0, 0]; % Initial point
options = optimoptions('simulannealbnd','PlotFcns',@saplotbestx);
[x,fval] = simulannealbnd(@rosen,x0,options);
% Multi-peak function optimization example: Ackley function
x0 = [rand, rand]; % Random initia
```
0
0
相关推荐
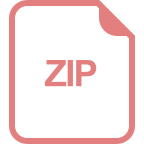
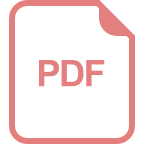
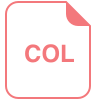
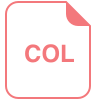
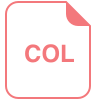
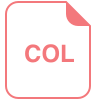
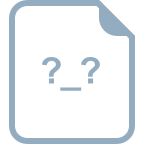
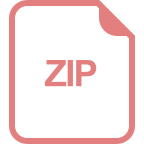