MATLAB Linear Programming: In-depth Analysis and Case Applications
发布时间: 2024-09-14 20:34:52 阅读量: 46 订阅数: 32 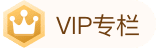
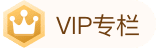
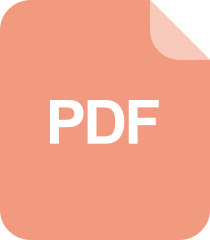
MATLAB and Simulink In-Depth
# 1. Overview of Linear Programming in MATLAB
## 1.1 What is Linear Programming?
Linear programming is a branch of mathematics that involves finding the maximum or minimum of a linear objective function subject to a series of linear inequalities or equations. In engineering, economics, and management fields, linear programming is a powerful tool used for optimal resource allocation, cost minimization, and production planning.
## 1.2 Advantages of MATLAB in Solving Linear Programming
MATLAB, as a widely used tool in the field of mathematical computation, offers robust linear programming solvers. Among them, MATLAB's `linprog` function is the main force in solving linear programming problems. It is not only easy to use but also compatible with various types of linear programming models. MATLAB's linear programming toolbox can handle various linear programming problems, including standard and general forms, and can solve large-scale and specific constraint conditions.
## 1.3 Applications of Linear Programming
In practical applications, linear programming is widely used in production scheduling, logistics distribution, supply chain management, financial portfolio optimization, and other fields. Through linear programming models, we can simplify complex systems and find optimal solutions. The next chapter will delve into the theoretical foundations of linear programming, laying a solid foundation for practical applications.
# 2. Theoretical Foundations of Linear Programming in MATLAB
Linear programming, as a mathematical optimization technique, plays a central role in computer algorithms and applied mathematics. It is widely used in economic analysis, engineering design, resource management, and logistics planning. MATLAB, as a powerful mathematical software, provides built-in linear programming functions and toolboxes for solving linear programming problems. This chapter aims to deeply explore the theoretical foundations of linear programming, laying a solid foundation for the subsequent chapters on MATLAB applications.
### 2.1 Mathematical Model of Linear Programming
#### 2.1.1 Objective Function and Constraints
The mathematical model of a linear programming problem mainly consists of an objective function and constraints. The objective function is a linear expression that we wish to maximize or minimize in linear programming, typically represented as:
\[ \text{minimize/maximize} \quad Z = c^T x \]
where \( c \) is the objective coefficient vector, \( x \) is the decision variable vector, and \( c^T \) denotes the transpose of \( c \).
Constraints describe the linear inequalities or equations that decision variables must satisfy, generally in the form:
\[ \begin{cases}
Ax \leq b \\
Ex = d \\
x \geq 0
\end{cases} \]
where \( A \) and \( E \) are coefficient matrices, \( b \) and \( d \) are constant vectors, and \( x \geq 0 \) represents the non-negativity constraint of the variables.
#### 2.1.2 Standard Form of Linear Programming Problems
The standard form of a linear programming problem can be simply described as:
\[ \text{minimize} \quad c^T x \]
\[ \text{subject to} \quad Ax = b \]
\[ \quad \quad \quad \quad x \geq 0 \]
where \( x \) represents the decision variables, \( c \) and \( b \) are constant vectors, and \( A \) is the coefficient matrix.
The standard form is the basis for linear programming problems, and other forms of linear programming problems can be transformed into the standard form through variable substitution and constraint transformation.
### 2.2 Algorithm Principles of Linear Programming
#### 2.2.1 Steps and Principles of the Simplex Method
The Simplex method is an iterative algorithm for finding the optimal solution to a linear programming problem in a multidimensional space. The basic steps include:
1. Convert the linear programming problem into an initial simplex tableau.
2. Improve the current solution through rotation operations (basis variable substitution) by finding an entering variable that can increase the objective function value.
3. Update the simplex tableau and iterate to the optimal solution.
The principle of the Simplex method is based on the geometric interpretation of linear programming problems, which is to find the optimal vertex of the objective function within the feasible solution space.
#### 2.2.2 Basic Concepts of the Interior Point Method
The Interior Point method is another algorithm for solving linear programming problems. Unlike the Simplex method, it starts iterating from an interior point within the feasible solution space and ultimately approaches the optimal solution. The advantage of this method is that it can find the optimal solution in polynomial time, especially suitable for large-scale problems.
The key steps of the Interior Point method include:
1. Choose an initial interior point that lies within the feasible solution space.
2. Gradually iterate to the optimal solution through gradient descent or other optimization strategies.
3. Adopt a central path tracking strategy to keep the iterative point always within the feasible solution space.
#### 2.2.3 Algorithm Efficiency and Selection
The Simplex method and the Interior Point method each have their advantages. The Simplex method is more effective for small and medium-sized problems, while the Interior Point method is more efficient when dealing with large-scale problems. The choice of an appropriate algorithm usually depends on the size, complexity of the problem, and the required accuracy of the solution.
### 2.3 The Role of MATLAB in Solving Linear Programming
#### 2.3.1 Introduction to MATLAB's Linear Programming Toolbox
MATLAB provides a set of linear programming toolboxes, including various functions for building and solving linear programming models, such as the `linprog` function. These toolbox functions not only simplify the solving process but also provide a rich set of parameters to customize the algorithm's behavior.
#### 2.3.2 Comparison of MATLAB with Other Linear Programming Tools
Compared to other linear programming tools like LINDO, CPLEX, MATLAB's linear programming toolbox has the following advantages:
- Integration: MATLAB is a multifunctional platform that seamlessly integrates with the linear programming toolbox, facilitating complex data processing and result analysis.
- Scalability: MATLAB supports users in extending the functionality of the linear programming toolbox by writing custom M language functions.
- Visualization: MATLAB's powerful graphical capabilities make result visualization more convenient, aiding in the analysis and interpretation of linear programming model solutions.
In this chapter, we have gained an in-depth understanding of the mathematical model of linear programming, explored its algorithmic principles, and analyzed MATLAB's role in solving linear programming problems. The subsequent chapters will introduce how to use MATLAB for practical operations of linear programming, providing practical skills for readers to apply the theory to real-world problems.
# 3. Practical Operations of Linear Programming in MATLAB
## 3.1 Usage of MATLAB's Linear Programming Functions
### 3.1.1 Syntax Structure of the `lpolve` Function
The `lpolve` function in MATLAB is a practical tool for solving linear programming problems. Before using the `lpolve` function, it is essential to understand its syntax structure to correctly build the problem and obtain solutions. The basic calling format of the function is as follows:
```matlab
[x, fval, exitflag, output] = lpolve(f, A, b, Aeq, beq, lb, ub, xint)
```
The roles of each parameter are as follows:
- `f`: Objective function coefficient vector, representing the objective function in the optimization problem.
- `A` and `b`: Coefficient matrix and constant vector of the linear inequality constraints, i.e., `Ax <= b`.
- `Aeq` and `beq`: Coefficient matrix and constant vector of the linear equality constraints, i.e., `Aeq*x = beq`.
- `lb` and `ub`: Lower and upper bound vectors for the variables, i.e., `lb <= x <= ub`.
- `xint`: Integer attribute vector for variables, specifying which variables must be integers.
- `x`: Obtained optimal solution vector.
- `fval`: Optimal value of the objective function.
- `exitflag`: Indicator of the function's exit status.
- `output`: Contains detailed information about the algorithm's execution.
### 3.1.2 Detailed Usage of the `linprog` Function
`linprog` is another commonly used linear programming solving function in MATLAB, and compared to `lpolve`, `linprog` is more intuitive and feature-rich. Here is the common calling format of the `linprog` function:
```matlab
x = linprog(f, A, b, Aeq, beq, lb, ub)
```
In this calling format:
- The meanings of `f`, `A`, `b`, `Aeq`, `beq`, `lb`, and `ub` are the same as in the `lpolve` function.
- `x`: Returns the optimal solution vector of the problem.
When `f` is a vector and negative, it solves a minimization problem; when `f` is positive, it solves a maximization problem.
In addition to the basic usage, the `linprog` function also provides other optional parameters, such as:
- `options`: A structure that sets solver parameters, controls output information, and the algorithm's convergence.
- `x0`: A vector for specifying an initial guess solution.
- `'Algorithm'`: Specifies the algorithm for solving the linear programming problem, with options including `'dual-simplex'`, `'primal-simplex'`, or `'interior-point'`.
How to set these optional parameters will be detailed in the next section.
## 3.2 Case Analysis of Linear Programming
### 3.2.1 Resource Allocation Problem in Economics
The resource allocation problem is a typical example of linear programming. Suppose there are multiple products that need to be produced under limited resources, aiming to achieve maximum profit while satisfying certain constraints. Here is a specific case:
Suppose there are two products, A and B. Producing A consumes resources 1 and 2, while producing B consumes resources 2 and 3. There are 100, 200, and 150 units of resources available, respectively. The profit for product A is $100, and for product B is $200. How should resources be allocated to maximize total profit?
First, set decision variables `x1` and `x2` to represent the production quantities of products A and B, respectively. The objective function is to maximize profit:
```
maximize Z = 100x1 + 200x2
```
Then, set the constraints according to the resource limits:
```
x1 + 2x2 <= 100 (Resource 1 limit)
2x1 + x2 <= 200 (Resource 2 limit)
x2 <= 150 (Resource 3 limit)
x1, x2 >= 0 (Non-negativity condition)
```
Next, implement the model solution through MATLAB code:
```matlab
f = [-100; -200]; % Objective function coefficient vector, note that we need to maximize, so negative values are used
A = [1 2; 2 1; 0 1];
b = [100; 200; 150];
Aeq = [];
beq = [];
lb = [0; 0];
ub = [];
[x, fval] = linprog(f, A, b, Aeq, beq, lb, ub);
```
This code will obtain the optimal quantities for producing products A and B, as well as the maximum profit achieved.
### 3.2.2 Engineering Optimization Design Case
In engineering design, linear programming can be used to optimize combinations of factors such as cost, weight, size, etc. For example, consider a simple material cost minimization problem.
Assume there are three materials (M1, M2, and M3) available for constructing a structure. Both the strength and cost of the structure are the primary considerations in the design. We need to build a structure with sufficient strength without exceeding the budget. The unit cost and required minimum strength for each material are as follows:
- M1: $10/unit, minimum strength 5 units
- M2: $15/unit, minimum strength 6 units
- M3: $20/unit, minimum strength 10 units
Assuming the total strength of the structure needs to be at least 100 units, and the total cost does not exceed $1500. We will use linear programming to determine the optimal material purchasing strategy.
By following a similar method to the resource allocation problem above, we can establish the objective function and constraints and use MATLAB to solve them. The specific MATLAB code implementation will involve constructing a cost minimization objective function and constraints for cost and strength.
## 3.3 Establishment and Solution of Linear Programming Models
### 3.3.1 How to Establish Mathematical Models
Establishing a linear programming model is the process of converting a real-world problem into a mathematical form. Generally, a linear programming problem can be divided into the following steps:
1. Determine decision variables: These are the unknowns in the problem that need to be solved.
2. Construct the objective function: Expressed as a linear combination of decision variables, usually to maximize or minimize total cost, total profit, etc.
3. Specify constraints: These limit the range of values or the relationship between decision variables, and these constraints must be linear.
4. Determine the boundary conditions of variables: Decision variables generally have upper and lower bounds, indicating the range of values that variables can take.
### 3.3.2 Steps and Result Analysis of Model Solution
Once a linear programming model is established, the next step is to use MATLAB to solve the model. The steps for solving the model generally are as follows:
1. Clearly define the parameters of the linear programming model using MATLAB syntax.
2. Use MATLAB-provided functions (e.g., `linprog`) to solve the problem.
3. Analyze the results and verify the feasibility of the results.
For example, when using the `linprog` function to solve, first define the objective function coefficient vector `f`, the inequality constraint matrix `A` and vector `b`, the equality constraint matrix `Aeq` and vector `beq`, as well as the lower and upper bounds of variables `lb` and `ub`. Then call the `linprog` function to solve and store the results in variables.
After solving, analyzing the results is an important step. It is necessary to check whether the results satisfy all constraints and achieve the desired objectives. Additionally, sensitivity analysis can be performed to understand the impact of parameter changes on the objective function.
The code example for result analysis is as follows:
```matlab
[x, fval, exitflag, output] = linprog(f, A, b, Aeq, beq, lb, ub);
if exitflag > 0
fprintf('The optimal solution is x = [%f, %f] with an objective value of %f\n', x(1), x(2), fval);
else
fprintf('Problem did not converge, exitflag = %d\n', exitflag);
end
```
After executing the code, the optimal solution and the corresponding optimal objective function value will be output. If `exitflag` is a positive number, it indicates successful solving; if zero or negative, it indicates that the solution did not converge, and typically, it is necessary to check whether the model settings are correct or whether the solver parameters need adjustment.
### 3.3.3 Flowchart of Model Solution and Result Analysis
To more intuitively illustrate the process of solving and analyzing linear programming models, the following is a simplified flowchart representation:
```mermaid
graph LR
A[Start] --> B[Define Decision Variables]
B --> C[Construct Objective Function]
C --> D[Set Constraints]
D --> E[Set Variable Boundaries]
E --> F[Solve Linear Programming with MATLAB]
F --> G[Check if Solution is Successful]
G -->|Successful| H[Output Optimal Solution and Objective Function Value]
G -->|Unsuccessful| I[Adjust Model Parameters or Check Model]
H --> J[End]
I --> F
```
With this flowchart, the entire process from establishing to solving and analyzing linear programming models can be clearly understood at a glance.
# 4. Advanced Applications of Linear Programming in MATLAB
## 4.1 Multi-Objective Linear Programming
Multi-objective linear programming is an extension of linear programming that involves multiple objective functions rather than a single objective function. In real-world problems, decision-makers often need to consider multiple factors; thus, multi-objective programming can more comprehensively describe problems and provide more reasonable solutions.
### 4.1.1 Definition of Multi-Objective Linear Programming
In multi-objective linear programming, we have multiple linear objective functions and a set of linear constraints. Usually, these objectives may be in competition with each other, and it is impossible to maximize or minimize all objectives simultaneously. Therefore, decision-makers need to find a compromise solution, also known as the Pareto optimal solution, so that no objective can be improved without at least one other objective becoming worse.
### 4.1.2 Methods for Solving Multi-Objective Linear Programming in MATLAB
MATLAB provides several methods to handle multi-objective linear programming problems, among which the most commonly used is the `fmincon` function combined with objective weights. In addition, the `gamultiobj` function can be used, which is specifically designed for solving multi-objective optimization problems.
```matlab
function [x, fval, exitflag, output, lambda] = multi_objective_optimization(A, b, Aeq, beq, lb, ub, nonlcon, objectives)
% The definitions of A, b, Aeq, beq, lb, ub, and nonlcon are similar to those of the lpolve function
% objectives is an array of function handles, each representing an objective function
% Convert the multi-objective optimization problem into a single-objective optimization problem, here using a simple weighted sum method
weights = [0.5, 0.5]; % Weights need to be adjusted according to the actual problem
% Define the composite objective function
obj = @(x) weights(1) * objectives{1}(x) + weights(2) * objectives{2}(x);
% Use the fmincon function to solve
options = optimoptions('fmincon', 'Display', 'iter', 'Algorithm', 'sqp');
[x, fval] = fmincon(obj, x0, A, b, Aeq, beq, lb, ub, nonlcon, options);
% Analyze the function's return parameters
exitflag = ... % Indicator of whether the result converges
output = ... % Detailed information about the optimization process
lambda = ... % Lagrange multipliers
end
```
When using `fmincon` for multi-objective optimization, the key lies in how to set the weights of the objective functions. Different weight combinations will yield different Pareto optimal solutions. In practical applications, it may be necessary to run the optimization process multiple times to explore the solution space and find a series of Pareto optimal solutions.
## 4.2 Integer Linear Programming
Integer linear programming (ILP) is a type of linear programming in which decision variables are restricted to integer values. This type of problem is very common in resource allocation, scheduling, network design, and financial models.
### 4.2.1 Characteristics and Applications of Integer Linear Programming
The characteristic of integer linear programming is that decision variables must be integers, making the problem more complex. Due to the introduction of integer constraints, ILP is typically an NP-hard problem, meaning that finding the optimal solution requires a significant amount of computational time in most cases. However, for small or medium-sized problems, both the commercial and academic worlds have developed many efficient algorithms and tools to solve such problems.
A classic application of integer linear programming is the Traveling Salesman Problem (TSP), where the shortest path visiting a series of cities and returning to the starting point needs to be found. This problem has extensive applications in logistics, manufacturing, and computer science.
### 4.2.2 Application of MATLAB Integer Programming Functions
The `intlinprog` function in MATLAB is a specialized tool for solving integer linear programming problems. It can solve integer programming problems by setting the variable type to `'integer'`.
```matlab
function [x, fval, exitflag, output] = integer_linear_programming(f, A, b, Aeq, beq, lb, ub, intcon)
% The definitions of f, A, b, Aeq, beq, lb, and ub are similar to those of linear programming
% intcon is a vector containing the indices of variables that need to be forced to be integers
% Define the integer programming problem
intcon = [1, 3]; % Assuming the first and third variables need to be integers
% Call the intlinprog function to solve
options = optimoptions('intlinprog', 'Display', 'iter');
[x, fval, exitflag, output] = intlinprog(f, intcon, A, b, Aeq, beq, lb, ub, options);
% Analyze the function's return parameters
exitflag = ... % Indicator of whether the result converges
output = ... % Detailed information about the optimization process
end
```
When using the `intlinprog` function, we need to pay attention to its limitations on problem size and solution time. For large-scale problems, it may be necessary to adopt branching and bounding methods or other strategies to accelerate the solution process.
## 4.3 Sensitivity Analysis of Constrained Linear Programming
Sensitivity analysis is an important step in optimization problems, involving checking the impact of small changes in decision variables, objective functions, or constraints on the optimal solution or optimal value.
### 4.3.1 Concepts and Methods of Sensitivity Analysis
In MATLAB, sensitivity analysis can be performed by analyzing the output of the `linprog` function. For each constraint condition of a linear programming problem, we can observe changes in the solution by altering the coefficients of the constraints.
### 4.3.2 MATLAB Tools for Sensitivity Analysis
MATLAB provides specialized tools to help users perform sensitivity analysis, such as the `lower` and `upper` fields in the `lambda` structure returned by the `linprog` function. These fields represent the dual variables for each constraint, which are the shadow prices of the objective function for these constraints.
```matlab
% Assuming A, b, f, lb, ub are defined
[x, fval, exitflag, output, lambda] = linprog(f, A, b, [], [], lb, ub);
% Sensitivity analysis
% Analyze the sensitivity of the ith constraint
i = 1;
dual_price = lambda.lower(i); % The dual variable (shadow price) for the ith constraint
```
In the above code, the dual variable (shadow price) can help us understand the sensitivity of the objective function to the ith constraint. If the dual variable for the ith constraint is very large, then small changes in this constraint will have a significant impact on the objective function value.
Sensitivity analysis is not limited to constraint conditions; it can also be used to analyze changes in objective function coefficients. In this way, decision-makers can gain a deeper understanding of the problem structure and make better decisions.
# 5. In-Depth Case Analysis of Linear Programming in MATLAB
After delving into the theory and practical operations of linear programming, this chapter will take you into the world of in-depth case analysis. Case analysis is a bridge that applies theory to practice and is an important way to understand the practical application value of linear programming in real work. We will analyze methods for dealing with large-scale linear programming problems and combine linear programming with practical application cases in various industries, revealing the tremendous potential of linear programming in solving complex problems.
## 5.1 Handling Large-Scale Linear Programming Problems
In real life, we often encounter large-scale linear programming problems with many variables and complex constraints. These problems often cannot be solved directly by simple optimization algorithms, so special techniques are needed to handle them.
### 5.1.1 Decomposition Techniques for Large Models
For large-scale linear programming problems, decomposition techniques are usually adopted to simplify the model and the solving process. For example, one of the decomposition techniques is Benders decomposition, which decomposes the original problem into a master problem and subproblems. Optimal solutions are approximated by iteratively solving these subproblems.
#### Code Block Display and Analysis
The following is a simple example of implementing Benders decomposition using MATLAB:
```matlab
function [x, fval] = benders_decomposition(A, b, c, max_iter)
% Input parameters:
% A - constraint matrix
% b - constraint vector
% c - objective function coefficients
% max_iter - maximum number of iterations
% Output parameters:
% x - optimal solution
% fval - optimal objective function value
% Initialization
x = zeros(length(c), 1);
x_bar = x;
lambda = 1;
fval = inf;
for k = 1:max_iter
% Solve the subproblem
[lambda_bar, s] = solve_subproblem(A, b, c, x_bar);
% Check the feasibility of the solution
if isinf(lambda_bar)
error('Subproblem is infeasible');
end
% Update the Lagrange multiplier
lambda = lambda + lambda_bar;
% Solve the master problem
x_bar = solve_masterproblem(A, b, lambda);
% Check convergence
if norm(x_bar - x) < 1e-4
break;
end
x = x_bar;
end
% Calculate the optimal value
fval = c' * x + lambda;
end
```
This code segment defines a basic framework for Benders decomposition. `A`, `b`, `c` are the constraint matrix, constraint vector, and objective function coefficients of the original linear programming problem, respectively. `max_iter` is the upper limit for the number of iterations. The function `solve_subproblem` is used to solve the subproblem, and `solve_masterproblem` is used to solve the master problem. Each iteration updates the Lagrange multiplier and checks the convergence of the solution.
Please note that this example code is intended to demonstrate the logic of implementing decomposition techniques and is not a complete implementation of the Benders decomposition algorithm. In practical applications, it is necessary to develop specific subproblem and master problem solvers according to the characteristics of the problem.
### 5.1.2 Application of MATLAB in Large-Scale Problems
MATLAB provides a series of efficient tools to handle large-scale linear programming problems, such as the `intlinprog` function, which is specifically designed for solving integer linear programming problems and supports large-scale models. In addition, MATLAB's Parallel Computing Toolbox allows linear programming algorithms to run on multi-core processors, significantly reducing solution time.
#### Code Block Display and Analysis
```matlab
% Using the intlinprog function to solve an integer linear programming problem
f = [-1; -1]; % Objective function coefficients
intcon = [1, 2]; % Specify the indices of integer variables
A = [3, 2; 2, 3; -1, 1];
b = [3; 5; 1];
lb = zeros(2, 1);
ub = [Inf, Inf];
[x, fval] = intlinprog(f, intcon, A, b, [], [], lb, ub);
% fval is the optimal objective function value, x is the optimal solution vector
```
The `intlinprog` function is used to solve integer linear programming problems of the form `min f'*x`, where `f` is the objective function coefficient vector, `intcon` is the index vector of integer variables, `A` and `b` define the linear constraint conditions, `lb` and `ub` define the lower and upper bounds of the variables, respectively. `x` and `fval` are the obtained optimal solution vector and objective function value, respectively.
Through these methods, MATLAB is capable of handling complex, large-scale linear programming problems, even in environments with large amounts of data, many variables, and complex constraint conditions, quickly finding the optimal or satisfactory solution.
## 5.2 Combining Linear Programming with Practical Applications
Applying the theory of linear programming to real-world problems can help us solve various engineering, economic, and management issues more effectively.
### 5.2.1 Industry Case Analysis
In many industries, including transportation planning, logistics management, production scheduling, and more, linear programming is widely used to optimize resource allocation, reduce costs, and improve efficiency.
#### Table Display and Analysis
| Industry | Application Scenario | Role of Linear Programming |
|-----------------|-----------------------------------------|---------------------------------------------------------------|
| Transportation | Path optimization, traffic control | Reduce transportation time, decrease congestion, improve system efficiency |
| Logistics Management | Goods scheduling, inventory control | Reduce storage costs, improve goods delivery speed and accuracy |
| Production Scheduling | Task sorting, equipment utilization | Improve production efficiency, reduce idle time and overtime |
These cases demonstrate the significant role of linear programming in optimizing decision-making processes. Each scenario requires the establishment of precise mathematical models, which are then solved using MATLAB's linear programming toolbox.
### 5.2.2 Application of Linear Programming in Project Management
In project management, linear programming can be used for resource allocation, project schedule planning, risk assessment, and more, helping project managers efficiently allocate limited resources and develop optimal project schedules.
#### Logical Flowchart Display
The following mermaid format flowchart shows a decision-making process that applies linear programming in project management:
```mermaid
graph TD
A[Project Resource Allocation Requirement Analysis] --> B[Establish Linear Programming Model]
B --> C[Solve Linear Programming Problem]
C --> D[Analyze Linear Programming Solution Results]
D --> E{Do the results meet the constraints?}
E -- Yes --> F[Generate Resource Allocation Plan]
E -- No --> G[Adjust Model Parameters]
G --> B
F --> H[Implement Resource Allocation Plan]
H --> I[Project Progress Monitoring and Evaluation]
```
Through such a process, project managers can systematically utilize linear programming to optimize resource allocation and achieve project goals. Any abnormalities at any step can be promptly fed back into the model for adjustments, ensuring that the project always moves towards the optimal direction.
These cases and applications are based on MATLAB's powerful linear programming toolbox. It not only provides robust solving capabilities but also features a user-friendly interface and comprehensive documentation support, making linear programming no longer an unapproachable mathematical model but a decision-making tool that can be implemented and bring practical benefits to enterprises and organizations.
Through the in-depth analysis of this chapter, we can see that MATLAB linear programming is not only theoretically complete but also efficient in practical applications. It can help us solve academic problems and penetrate into various industries to solve practical problems, which is a significant advantage of MATLAB as an industry-leading mathematical computation software.
# 6. Optimization and Prospects of MATLAB Linear Programming
## 6.1 Optimization Strategies for Linear Programming Models
In dealing with linear programming problems, model optimization strategies are key to ensuring a fast and efficient solution process. Model optimization can start from various perspectives, such as reducing the problem size through preprocessing or applying heuristic algorithms to find approximate optimal solutions.
### 6.1.1 Model Simplification and Preprocessing Techniques
When building linear programming models, simplifying the model to reduce computational complexity is a common optimization method. Here are some commonly used model simplification techniques:
1. **Variable Elimination**: If a linear programming model contains variables that only appear in inequality constraints and not in the objective function, these variables can be attempted to be eliminated.
2. **Constraint Simplification**: Redundant constraint conditions can be eliminated without affecting the optimal solution of the problem, reducing the model's complexity.
3. **Preprocessing Models**: Through preprocessing, such as standardizing variables, merging similar constraints, etc., the efficiency of solvers can be improved.
### 6.1.2 Application of Heuristic Algorithms in Optimization
For large-scale or particularly complex linear programming problems, it may be difficult to find the exact solution in a reasonable time. In these cases, heuristic algorithms can provide approximate solutions. Here are two common heuristic algorithms:
1. **Greedy Algorithm**: In each step, the locally optimal choice is selected without considering the global optimal solution. For example, in resource allocation problems, a greedy algorithm might prioritize resource allocation to the project with the greatest current benefit.
2. **Simulated Annealing Algorithm**: By simulating the annealing process of materials, the algorithm allows for a certain degree of "退步" (退步) during the search for the optimal solution, thus escaping local optima and increasing the probability of finding the global optimal solution.
In MATLAB, using heuristic algorithms for optimization may require custom functions or the combination of existing toolboxes.
## 6.2 Future Development of MATLAB Linear Programming Toolbox
MATLAB's linear programming toolbox is constantly being updated and improved to meet increasingly complex application scenarios and research needs.
### 6.2.1 Introduction to New Features in New Versions
With the update of MATLAB versions, the linear programming toolbox is also introducing new features. For example:
1. **Improved Solvers**: To handle larger-scale linear programming problems, new versions may introduce more efficient solvers.
2. **Multi-thread Support**: To improve performance, new versions may provide support for multi-threaded computing.
3. **Enhanced Graphical User Interface (GUI)**: New versions of MATLAB may offer a more intuitive and user-friendly GUI to help users build and solve linear programming problems more quickly.
### 6.2.2 Prospects for Future Research
With the continuous development of computer science, operations research, and machine learning, the future development trends of the linear programming toolbox may include:
1. **Integration of Machine Learning Algorithms**: To improve solving efficiency, machine learning algorithms may be integrated into the linear programming solving process, such as guiding the search by predicting the location of the optimal value.
2. **Multi-objective Solving Optimization**: Multi-objective linear programming will be further developed, providing decision-makers with more choices and flexibility.
3. **Cloud Computing Integration**: With the maturity of cloud computing technology, future MATLAB toolboxes may support cloud computing resources, enabling parallel computing for larger-scale linear programming problems.
In the optimization and prospects chapter, we can see that MATLAB's linear programming toolbox has tremendous potential for improving algorithm efficiency, adding new features, and integrating emerging technologies. With the update of MATLAB versions, we can look forward to more improvements and innovations to solve the complex challenges of real-world problems.
0
0
相关推荐
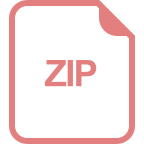
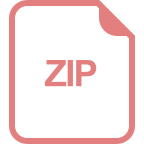
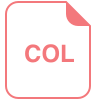
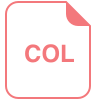
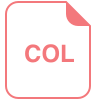
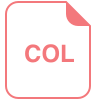
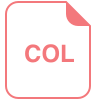
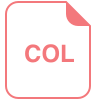