MATLAB State-Space Control Design: In-depth Understanding and Applications
发布时间: 2024-09-15 00:39:04 阅读量: 29 订阅数: 30 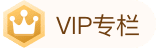
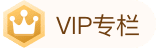
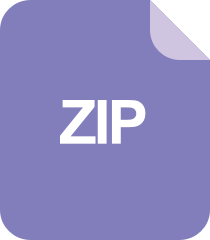
java+sql server项目之科帮网计算机配件报价系统源代码.zip
# 1. Basic Concepts of State Space Models
In modern control theory, state space models are essential tools for describing the behavior of dynamic systems. State space models provide a mathematical framework for representing the relationship between a system's internal state and external inputs, allowing for systematic and comprehensive analysis and design of controllers. This chapter aims to introduce the foundational knowledge of state space models and lay the groundwork for constructing and analyzing state space models using MATLAB in subsequent chapters.
## 1.1 Definition of State Space Models
State space models consist of a set of first-order differential equations typically used for representing continuous-time systems. The model defines the time evolution of the system's state vector and describes how the state changes over time and the relationship between the state, inputs, and outputs.
- State equations: Describe how the system's internal state evolves over time.
- Output equations: Describe how the system's output is determined by the current state and inputs.
## 1.2 The Role of System Matrices
The core of the state space model is the system matrices, including the state matrix A, input matrix B, output matrix C, and direct transmission matrix D. These matrices describe the dynamic relationships between the system's state, inputs, and outputs and are defined and manipulated using specific functions and methods in MATLAB.
- State matrix A: Defines how the state changes over time.
- Input matrix B: Defines how inputs affect the change in state.
- Output matrix C: Defines how to calculate the system output from the current state.
- Direct transmission matrix D: Defines how inputs directly influence the output.
With these matrices, we can perform simulations, stability analyses, and controller design in MATLAB. Next, we will delve into the definitions of these matrices and their representations in MATLAB.
# 2. The Application of MATLAB in State Space Model Construction
### 2.1 Basic Operations and Matrix Processing in MATLAB
#### 2.1.1 Introduction to the MATLAB Environment
MATLAB (an abbreviation for Matrix Laboratory) is an advanced language and interactive environment for numerical computation, visualization, and programming. In constructing state space models, MATLAB provides a powerful platform to simplify complex mathematical operations and model analysis. The MATLAB environment includes multiple toolboxes, among which the Control System Toolbox provides various functions and commands necessary for building and analyzing state space models.
#### 2.1.2 Matrix Operations and Function Application
Matrices are at the core of MATLAB, and almost all computations can be translated into matrix operations. In state space models, the system matrices A, B, C, and D constitute the matrix equations describing system dynamics. In MATLAB, we can utilize its rich matrix operation commands, such as creating, modifying, solving, and plotting matrices.
Here is a simple example demonstrating how to create a state space model matrix in MATLAB:
```matlab
A = [0 1; -2 -3];
B = [0; 1];
C = [1 0];
D = 0;
```
This code defines a simple state space model with a 2x2 system matrix A, a 2x1 input matrix B, a 1x2 output matrix C, and a scalar transmission matrix D.
### 2.2 MATLAB Representation of State Space Models
#### 2.2.1 Definition and Input of System Matrices
The key to state space models lies in correctly defining the system matrices. In MATLAB, we can use the `ss` function to create a state space model object. The `ss` function accepts four matrices as parameters representing the system matrix A, input matrix B, output matrix C, and transmission matrix D.
```matlab
sys = ss(A, B, C, D);
```
#### 2.2.2 Visualization of State Space Models
After creating a model, we can use various MATLAB commands to visualize the model's characteristics. For example, the `step` function can be used to plot the system's step response, while the `bode` function can plot the system's frequency response.
```matlab
step(sys);
bode(sys);
```
### 2.3 Model Transformation and Simplification
#### 2.3.1 Conversion Between Continuous and Discrete Time Models
In practical applications, depending on different needs, we may need to convert between continuous-time models and discrete-time models. MATLAB provides `c2d` and `d2c` functions to perform such conversions.
```matlab
sysd = c2d(sys, T, 'zoh'); % Convert continuous model to discrete model
sysh = d2c(sysd); % Convert discrete model back to continuous model
```
#### 2.3.2 Model Reduction Techniques
Model reduction helps to reduce computational complexity and improve the efficiency of simulation and control. MATLAB's `balred` function can help us reduce the model using the balanced truncation method.
```matlab
[sysr, g] = balred(sys, r); % where r is the order of the new model, g is the error bound of reduction
```
The reduction operation can be detailed in the following table:
| Function | Purpose |
| --- | --- |
| `balred` | Model reduction through balanced truncation |
| `r = 5` | Specify the target order of the new model |
| `g` | Error bound of the reduced model |
This demonstrates how to construct and perform basic operations on state space models in MATLAB. The next section will explore model visualization and conversion and how to further simplify models to meet specific application requirements.
# 3. Analysis of State Space Control Systems
## 3.1 System Stability Analysis
### 3.1.1 Criteria for Linear System Stability
Analyzing the stability of a linear system is a fundamental step in control system design. A system is considered stable if it produces bounded outputs for any bounded input. In state space models, the stability of a linear time-invariant (LTI) system can be judged by the eigenvalues of its state matrix A. If all the real parts of the eigenvalues of matrix A are less than zero, then the system is asymptotically stable.
In MATLAB, we can use the `eig` function to calculate the eigenvalues and determine whether they satisfy the stability condition. Here is a simple code example for calculating eigenvalues and judging stability:
```matlab
A = [...]; % Define state matrix A
eigenvalues = eig(A); % Calculate eigenvalues
if all(real(eigenvalues) < 0)
disp('The system is stable');
else
disp('The system is unstable');
end
```
### 3.1.2 Stability Analysis Tools in MATLAB
MATLAB provides a rich set of tools for analyzing system stability, including but not limited to the `lyapunov` function, which can be used to solve Lyapunov equations and analyze system stability. For linear systems, Lyapunov's first method tells us that if there exists a symmetric positive definite matrix P such that the Lyapunov equation `A'*P + P*A = -Q` has a unique solution, then the system is stable.
The following code shows how to use the `lyapunov` function:
```matlab
Q = eye(size(A)); % Define a positive definite matrix Q
P = lyap(A, -Q); % Calculate the solution to the Lyapunov equation
if all(min(real(eig(P))) > 0)
disp('The system is stable');
else
disp('The system is unstable');
end
```
## 3.2 System Performance Evaluation
### 3.2.1 Step Response and Frequency Response Analysis
Evaluating system performance is a complex process involving both time and frequency domains. In the time domain, the step response is a critical indicator reflecting the system's response to a step input. Ideally, a system should have a fast rise time and minimal overshoot. MATLAB provides the `step` function to simulate the system's step response.
```matlab
sys = ss(A, B, C, D); % Define state space model
step(sys); % Plot step response
title('System Step Response');
```
In the frequency domain, the Bode plot is a common tool for evaluating system performance, providing information on how system gain and phase angle change with frequency. MATLAB's `bode` function can be used to plot the Bode diagram.
```matlab
bode(sys); % Plot Bode diagram
title('System Bode Diagram');
```
### 3.2.2 Effect of Pole Placement and Damping Ratio
The pole placement of a system has a decisive impact on system performance. By changing the positions of the poles, the system's response characteristics to specific inputs can be improved, such as accelerating the response speed or increasing system stability. MATLAB's `place` function can be used to design state feedback control laws to achieve the desired pole placement.
The following code shows how to use the `place` function:
```matlab
K = place(A, B, poles); % poles are the desired pole positions
sys_cl = ss(A-B*K, B, eye(size(B)), zeros(size(B)));
step(sys_cl); % Plot the step response of the closed-loop system
title('Step Response of the Closed-Loop System');
```
The damping ratio is another important parameter that describe
0
0
相关推荐
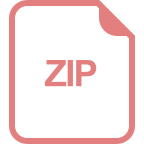
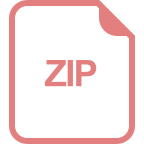
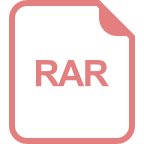
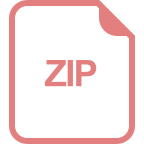
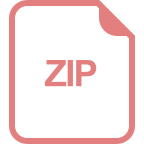