【Practical Exercise】MATLAB Simulated Annealing Algorithm for Multi-objective Optimization
发布时间: 2024-09-14 00:24:32 阅读量: 39 订阅数: 38 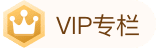
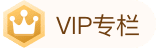
# 1. Overview of MATLAB Simulated Annealing Algorithm
Simulated annealing is a global optimization algorithm based on the annealing principle from statistical mechanics. It simulates the physical annealing process, where the system gradually evolves from a high-energy state to a low-energy state by continuously reducing the temperature, ultimately reaching the optimal solution. The simulated annealing algorithm has strong global search capabilities and can effectively solve complex optimization problems.
In MATLAB, the simulated annealing algorithm can be implemented through the following steps:
1. **Define the objective function:** Define the objective function to be optimized, which accepts input parameters and returns a scalar value.
2. **Initialize parameters:** Set algorithm parameters, including initial temperature, cooling rate, and termination conditions.
3. **Generate an initial solution:** Create a random initial solution.
4. **Iterative optimization:** In each iteration, generate a new solution and calculate its objective function value. If the new solution is better than the current one, accept it; otherwise, accept it based on probability.
5. **Cooling:** Gradually reduce the temperature as iterations proceed, causing the algorithm to converge to the optimal solution.
6. **Termination:** When the termination conditions are met, the algorithm stops and returns the optimal solution.
# 2. Principles and Implementation of Simulated Annealing Algorithm
### 2.1 Theoretical Foundations
#### 2.1.1 Concepts and Principles of Simulated Annealing Algorithm
Simulated annealing (SA) is a global optimization algorithm based on statistical principles, inspired by the annealing process of metals. In metal annealing, the metal is heated to a certain temperature and then cooled slowly to reach an optimal internal structure. The SA algorithm simulates this process by continuously adjusting algorithm parameters (temperature) to perform random exploration in the search space, finding the optimal solution.
The basic principles of the SA algorithm are as follows:
- **Initialization:** Set algorithm parameters (temperature, initial solution, termination conditions, etc.).
- **Iteration:** Generate a new candidate solution at the current temperature and calculate its objective function value.
- **Acceptance Criteria:** Accept or reject the candidate solution based on the Metropolis criterion. If the candidate solution is better than the current one, accept it directly; if it is worse, accept it with a certain probability.
- **Temperature Update:** Gradually lower the temperature as iterations proceed to reduce the range of random exploration and improve the convergence of the algorithm.
- **Termination:** When termination conditions (reaching the maximum number of iterations or temperature dropping to a sufficiently low level) are met, the algorithm stops and returns the current optimal solution.
#### 2.1.2 Algorithm Flow and Parameter Settings
The flow of the SA algorithm is as follows:
```mermaid
graph LR
subgraph Initialization
A[Initialize Parameters] --> B[Generate Initial Solution]
end
subgraph Iteration
C[Generate Candidate Solution] --> D[Calculate Objective Function Value] --> E[Accept or Reject Candidate Solution]
end
subgraph Termination
F[Update Temperature] --> G[Termination Conditions] --> H[Return Optimal Solution]
end
A --> C
D --> E
E --> F
F --> C
F --> G
```
The settings of SA algorithm parameters greatly affect the performance of the algorithm. The main parameters include:
- **Initial Temperature:** The initial temperature determines the exploration capability of the algorithm; the higher the temperature, the broader the exploration range.
- **Cooling Rate:** The cooling rate controls the speed of temperature reduction, affecting the convergence speed and quality of the solution.
- **Termination Conditions:** Termination conditions determine the running time of the algorithm and can be set to the maximum number of iterations or a temperature threshold.
### 2.2 Practical Application
#### 2.2.1 Implementation of Simulated Annealing in MATLAB
MATLAB provides an optimization toolbox that includes the implementation of the simulated annealing algorithm. The following code can be used to implement the SA algorithm:
```matlab
% Define the objective function
objectiveFunction = @(x) sum(x.^2);
% Set algorithm parameters
initialTemperature = 100;
coolingRate = 0.95;
maxIterations = 1000;
% Initialize the algorithm
sa = simulannealbnd(@objectiveFunction, [-10, 10], [-10, 10], ...
'Temperature', initialTemperature, 'CoolingRate', coolingRate, ...
'MaxIterations', maxIterations);
% Solve for the optimal solution
[x, fval] = sa.BestPoint;
% Output the optimal solution
disp(['Optimal solution: ', num2str(x)]);
disp(['Objective function value: ', num2str(fval)]);
```
#### 2.2.2 Parameter Tuning and Algorithm Performance Analysis
The performance of the SA algorithm is influenced by parameter settings. Parameters can be optimized using methods such as grid search or adaptive adjustment. The following table summarizes the impact of different parameter settings on algorithm performance:
| Parameter | Effect |
|---|---|
| Initial Temperature | Exploration Capability |
| Cooling Rate | Convergence Speed and Solution Quality |
| Termination Conditions | Running Time |
By comparing algorithm performance under different parameter settings, the optimal combination of parameters can be found.
# 3. Multi-Objective Optimization with MATLAB Simulated Annealing
### 3.1 Introduction to Multi-Objective Optimization Problems
#### 3.1.1 Definition and Characteristics of Multi-Objective Optimization Problems
A multi-objective optimization problem involves optimizing multiple objective functions simultaneously, where each objective function represents a different optimization goal. Unlike single-objective optimization problems, there is no single best solution in multi-objective optimization problems, but rather a set of solutions known as Pareto optimal solutions.
A Pareto optimal solution is one where no objective function can be improved without simultaneously worsening another objective function. In other words, for a Pareto optimal solution, if you want to improve one objective function, you must sacrifice another or others.
Multi-objective optimization problems have the following characteristics:
- **Objective Conflict:** Different objective functions typically conflict with each
0
0