【Introduction to Newton's Method and Its Implementation in Matlab】
发布时间: 2024-09-13 22:51:44 阅读量: 37 订阅数: 52 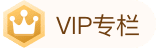
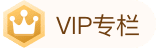
# Introduction to Newton's Method and Its Implementation in Matlab
## 1. Theoretical Basis of Newton's Method
Newton's method, also known as the tangent method, is an iterative technique for solving nonlinear equations. The fundamental idea is to draw the tangent to the function f(x) at a given point x0, and find the point of intersection x1, where the tangent intersects the x-axis. Subsequently, using x1 as the new starting point, the process is repeated until a termination condition is met.
The iterative formula for Newton's method is given by:
```
x_{n+1} = x_n - f(x_n) / f'(x_n)
```
Where:
* x_n is the approximation at the nth iteration
* f(x_n) is the value of the function at x_n
* f'(x_n) is the derivative of the function at x_n
## 2. Implementation of Newton's Method in Matlab
### 2.1 Matlab Code for Newton's Method Algorithm
The Matlab code for Newton's method algorithm mainly consists of two parts: solving the Newton's equation and computing the derivatives.
#### 2.1.1 Solving Newton's Equation
```matlab
function x = newton_method(f, df, x0, tol, max_iter)
% Newton's method for equation solving
% Input:
% f: Equation function
% df: Derivative function of the equation
% x0: Initial guess value
% tol: Tolerance
% max_iter: Maximum number of iterations
% Output:
% x: Obtained root
% Initialization
x = x0;
iter = 0;
% Iterative solution
while iter < max_iter && abs(f(x)) > tol
x = x - f(x) / df(x);
iter = iter + 1;
end
% Check for convergence
if iter == max_iter
warning('Newton method did not converge, reached maximum iterations.');
end
end
```
**Code Logic Analysis:**
* The `newton_method` function accepts the equation function `f`, derivative function `df`, initial guess value `x0`, tolerance `tol`, and maximum number of iterations `max_iter` as inputs and returns the obtained root `x`.
* The function initializes `x` to `x0` and sets the iteration counter `iter` to zero.
* It enters an iterative loop where the Newton's iteration formula is used to update the value of `x` and increment `iter`.
* The loop condition is that `iter` is less than `max_iter` and the absolute value of the equation value `f(x)` is greater than `tol`.
* If the number of iterations reaches `max_iter`, a warning is issued indicating that Newton's method has not converged.
#### 2.1.2 Computing Derivatives
```matlab
function df = derivative(f, x)
% Calculate the derivative of a function
% Input:
% f: Function
% x: Independent variable
% Output:
% df: Derivative value
% Numerical differentiation
h = 1e-6;
df = (f(x + h) - f(x)) / h;
end
```
**Code Logic Analysis:**
* The `derivative` function takes the function `f` and the independent variable `x` as inputs and returns the derivative value `df`.
* The function uses numerical differentiation to compute the derivative by approximating the difference in function values at `x` and a small increment `h` nearby.
* The numerical differentiation method is simple and easy to use but has limited precision, especially when the function has drastic changes or noise.
### 2.2 Analysis of Newton's Method Convergence
#### 2.2.1 Convergence Conditions
The sufficient conditions for Newton's method to converge are that, in the neighborhood of the root, the equation satisfies:
* The second derivative of the equation is not zero.
* The third derivative of the equation exists and is bounded.
#### 2.2.2 Convergence Speed
The convergence speed of Newton's method is related to the second derivative of the equation. If the second derivative remains positive (negative) in the neighborhood of the root, then Newton's method will converge at a quadratic (super-quadratic) speed.
## 3. Application of Newton's Method in Matlab**
**3.1 Solving Polynomial Equations**
Newton's method is widely used in solving polynomial equations. For a general polynomial equation:
```
P(x) = a_n*x^n + a_{n-1}*x^{n-1} + ... + a_1*x + a_0 = 0
```
We can iteratively solve for the roots of the equation using Newton's method.
**3.1.1 Specific Implementation Steps**
The specific steps for implementing Newton's method to sol
0
0
相关推荐








