【Advanced】Complex Matrix Operations: Schur Decomposition and Jordan Form in MATLAB
发布时间: 2024-09-13 23:49:32 阅读量: 39 订阅数: 52 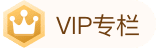
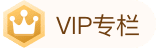
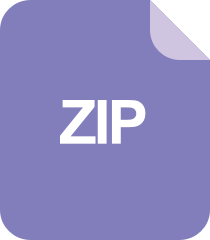
Schur_Decomposition_20:Schur_Decomposition_of_some_graphs_with_twenty_vertices
# Advanced Topic: Advanced Matrix Operations - Schur Decomposition and Jordan Form in MATLAB
## 2.1 Basic Concepts and Properties of Schur Decomposition
Schur decomposition is a matrix factorization technique that decomposes a matrix into the product of an upper triangular matrix and a unitary matrix.
**Basic Concepts:**
Given a complex matrix A, its Schur decomposition is represented as:
```
A = Q * T * Q^H
```
Where:
* Q is a unitary matrix, meaning Q^H * Q = I
* T is an upper triangular matrix, whose diagonal elements are the eigenvalues of A
**Properties:**
* Schur decomposition is unique; that is, for a given A, there exists a unique Q and T that satisfy the decomposition.
* The diagonal elements of T are the eigenvalues of A, arranged according to their algebraic multiplicity.
* The columns of Q are the eigenvectors of A, i.e., A * Q = Q * T.
## 2. Schur Decomposition Theory and Algorithms
### 2.1 Basic Concepts and Properties of Schur Decomposition
**Schur Decomposition** is a matrix factorization technique that decomposes a square matrix into the product of an upper triangular matrix and a unitary matrix. For a real symmetric matrix, its Schur decomposition form is:
```
A = Q * T * Q^T
```
Where:
***A** is the real symmetric matrix to be decomposed
***Q** is a unitary matrix, meaning its transpose is equal to its inverse
***T** is an upper triangular matrix, whose diagonal elements are the eigenvalues of A
Schur decomposition has the following properties:
***Orthogonality:** Q is a unitary matrix, thus Q^T * Q = I, where I is the identity matrix. This indicates that the columns of Q are orthogonal.
***Diagonalization:** T is a diagonal matrix, hence its diagonal elements are the eigenvalues of A.
***Uniqueness:** For a given A, its Schur decomposition is unique, except that the order of the columns of the unitary matrix Q may vary.
### 2.2 Algorithm Implementation of Schur Decomposition
#### 2.2.1 QR Algorithm
The QR algorithm is an iterative algorithm used to compute the Schur decomposition of a matrix. The algorithm decomposes A into the product of an upper triangular matrix and a unitary matrix through a series of QR decompositions.
**Algorithm Steps:**
1. Initialize A as A0.
2. For k = 1, 2, ..., n-1, perform the following steps:
* Perform a QR decomposition on A_k to obtain A_k = Q_k * R_k.
* Update A_k+1 = R_k * Q_k.
3. Let T = A_n, Q = Q_1 * Q_2 * ... * Q_n.
**Code Block:**
```python
def schur_qr(A):
"""
Computes the Schur decomposition of matrix A using the QR algorithm.
Parameters:
A: The real symmetric matrix to be decomposed
Returns:
T: An upper triangular matrix whose diagonal elements are the eigenvalues of A
Q: A unitary matrix whose columns are the eigenvectors of A
"""
n = A.shape[0]
Q = np.eye(n)
for k in range(n-1):
A, Q_k = qr(A)
Q = Q @ Q_k
T = A
return T, Q
```
**Logical Analysis:**
The code block implements the QR algorithm. It first initializes A as A0, then iteratively updates A through a series of QR decompositions. In each iteration, it decomposes A into the product of an upper triangular matrix and a unitary matrix and updates A and Q. Finally, it returns the upper triangular matrix T and the unitary matrix Q.
#### 2.2.2 Divide and Conquer Method
The divide and conquer method is a recursive algorithm used to compute the Schur decomposition of a matrix. The algorithm breaks down A into smaller blocks and recursively computes the Schur decomposition of these blocks.
**Algorithm Steps:**
1. If A is a 2x2 matrix, directly compute its eigenvalues and eigenvectors.
2. Otherwise, decompose A into the following form:
```
A = [A11 A12]
[A21 A22]
```
***
***
***bine the Schur decompositions of A11 and A22 to obtain the Schur decomposition of A.
**Code Block:**
```python
def schur_divide(A):
"""
Computes the Schur decomposition of matrix A using the divide and conquer method.
Parameters:
A: The real symmetric matrix to be decomposed
Returns:
T: An upper triangular matrix whose diagonal elements are the eigenvalues of A
Q: A unitary matrix whose columns are the eigenvectors of A
"""
n = A.shape[0]
if n == 2:
return schur_2x2(A)
else:
# Decompose A
A11 = A[:n//2, :n//2]
A12 = A[:n//2, n//2:]
A21 = A[n//2:, :n//2]
A22 = A[n//2:, n//2:]
# Recursively compute the Schur decomposition of the submatrices
T11, Q1 = schur_divide(A11)
T22, Q2 = schur_divide(A22)
# Combine the Schur decompositions of the submatrices
T = np.block([[T11, A12 @ Q2],
[Q1.T @ A21, T22]])
Q = np.block([[Q1, np.zeros((n//2, n//2))],
[np.zeros((n//2, n//2)), Q2]])
return T, Q
```
**Logical Analysis:**
The code block implements the divide and conquer method. It first checks if A is a 2x2 matrix; if so, it directly
0
0
相关推荐







