【Advanced Chapter】Advanced Optimization Algorithms: Interior Point Method and Duality Method in MATLAB
发布时间: 2024-09-14 00:01:36 阅读量: 21 订阅数: 38 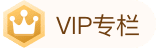
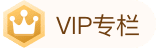
# [Advanced篇] Advanced Optimization Algorithms: Interior Point Method and Duality Method in MATLAB
## 2.1 Fundamental Principles of the Interior Point Method
The interior point method is an optimization algorithm used to solve optimization problems such as linear programming, quadratic programming, and nonlinear programming. Its basic principle involves a series of iterative steps that continuously narrow down the feasible region until the optimal solution is approximated.
The interior point method transforms the optimization problem into a set of equivalent barrier problems, where the barrier function is a convex function. As the number of iterations increases, the barrier function gradually diminishes, thereby approximating the optimal solution. The introduction of the barrier function ensures that the algorithm iterates within the feasible region, avoiding the pitfall of infeasible solutions.
## 2. Interior Point Method Theory and Practice
### 2.1 Fundamental Principles of the Interior Point Method
The interior point method is an iterative algorithm for solving linear programming problems, with the basic idea of iterating within the feasible region to gradually approach the optimal solution. The core of the interior point method is to maintain a central point within the feasible region and, through a series of iterative steps, move the central point closer to the optimal solution.
The main steps of the interior point method are as follows:
1. **Initialization:** Choose an initial central point within the feasible region and set an initial step size.
2. **Direction Search:** Calculate a search direction that reduces the objective function value when moved along.
3. **Step Size Selection:** Determine an appropriate step size to ensure that moving along the search direction still maintains feasibility.
4. **Central Point Update:** Move the central point along the search direction and update the step size.
5. **Termination Condition:** When the central point satisfies a certain termination condition, stop the iteration and output the optimal solution.
### 2.2 Algorithm Flow of the Interior Point Method
The algorithm flow of the interior point method is as follows:
```mermaid
graph LR
subgraph Interior Point Method Algorithm Flow
init[Initialization] --> dir[Direction Search] --> step[Step Size Selection] --> update[Central Point Update]
update --> dir
dir --> step
step --> update
update --> stop[Termination Condition]
end
```
### 2.3 MATLAB Implementation of the Interior Point Method
```matlab
% Interior Point Method for Solving Linear Programming Problems
function [x, fval] = interior_point(f, A, b, lb, ub)
% Initialization
n = size(A, 2);
x0 = (lb + ub) / 2;
t0 = 1;
mu = 1e-6;
% Iteration
while true
% Direction Search
d = -inv(A' * A + mu * diag(x0)) * (A' * f + mu * (x0 - lb) - mu * (ub - x0));
% Step Size Selection
alpha = 1;
while (x0 + alpha * d) >= lb & (x0 + alpha * d) <= ub
alpha = alpha * 2;
end
alpha = alpha / 2;
% Central Point Update
x0 = x0 + alpha * d;
t0 = t0 / alpha;
mu = mu * t0;
% Termination Condition
if norm(d) < 1e-6
break;
end
end
% Output the Optimal Solution
x = x0;
fval = f' * x;
end
```
0
0
相关推荐
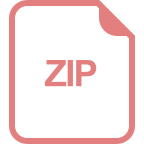
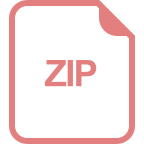
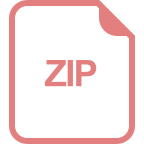
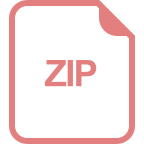
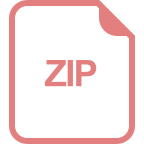
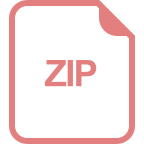
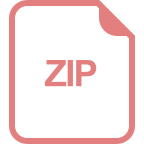
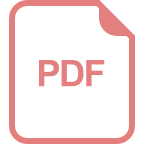
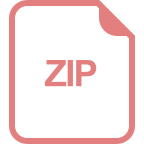