[Practical Exercise] Improving Particle Swarm Optimization with Genetic Algorithm in MATLAB (GA-PSO Algorithm)
发布时间: 2024-09-14 00:23:03 阅读量: 20 订阅数: 33 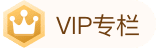
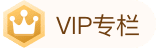
# 2.1 Principles and Implementation of Genetic Algorithms
Genetic Algorithms (GAs) are optimization algorithms inspired by the process of biological evolution. They search for optimal solutions by simulating natural selection and genetic mechanisms.
**2.1.1 Encoding and Decoding in Genetic Algorithms**
Encoding is the process of representing solutions in the problem space as binary strings or other data stru***mon encoding methods include binary encoding, real-number encoding, and tree encoding.
**2.1.2 Crossover and Mutation in Genetic Algorithms**
Crossover is the process of exchanging genetic segments between two parent individuals to produce new offspring. Mutation is a probabilistic event that randomly changes individual genes, ***mon crossover operators include single-point crossover, two-point crossover, ***mon mutation operators include bit-flip, Gaussian mutation, and uniform mutation.
# 2. Theoretical Foundations of the GA-PSO Algorithm
### 2.1 Principles and Implementation of Genetic Algorithms
#### 2.1.1 Encoding and Decoding in Genetic Algorithms
Genetic Algorithms (GA) are optimization algorithms inspired by biological evolution. They utilize a set of candidate solutions (called chromosomes) to search for optimal solutions. Each chromosome consists of a set of genes, where each gene represents a specific characteristic of the solution.
**Encoding** refers to re***mon encoding schemes include:
- **Binary encoding:** representing solutions as a string of binary bits.
- **Real-number encoding:** representing solutions as a set of real numbers.
- **Symbolic encoding:** representing solutions as a set of symbols or characters.
**Decoding** refers to converting chromosomes into problem solutions. The decoding scheme corresponds to the encoding scheme. For example, for binary encoding, the decoder converts binary bits into real numbers or other required data types.
#### 2.1.2 Crossover and Mutation in Genetic Algorithms
**Crossover** is an important operation in genetic algorithms that allows chromosomes to exchange genes. Cro***
***mon crossover operations include:
- **Single-point crossover:** Randomly select a point on the chromosome and swap the genes after that point.
- **Two-point crossover:** Randomly select two points on the chromosome and swap the genes between those points.
- **Uniform crossover:** Swap each gene on the chromosome with a certain probability.
**Mutation** is another crucial operation in genetic algorithms that allows chromosomes to rand***
***mon mutation operations include:
- **Bit-flip:** For binary encoding, randomly flip one or more binary bits.
- **Gaussian mutation:** For real-number encoding, add a random number that follows a normal distribution to the gene.
- **Swap mutation:** Randomly select two genes on the chromosome and swap their positions.
### 2.2 Principles and Implementation of Particle Swarm Optimization Algorithms
#### 2.2.1 Initialization of Particle Swarm Optimization Algorithms
Particle Swarm Optimization (PSO) is an optimization algorithm inspired by the behavior of groups such as bird flocks or fish schools. It uses a set of particles to search for optimal solutions. Each particle represents a potential solution and has a position and velocity.
The initialization of PSO algorithms includes:
- **Initialization of particle positions:** Randomly initialize the positions of each particle, where the position represents the characteristics of the solution.
- **Initialization of particle velocities:** Randomly initialize the velocities of each particle, where the velocity represents the direction and magnitude of the particle's movement.
#### 2.2.2 Updating of Particle Swarm Optimization Algorithms
The updating process of PSO algorithms is as follows:
- **Updating particle velocities:** The velocity of each particle is updated based on its current velocity, its personal best position, and the global best position.
- **Updating particle positions:** The position of each particle is updated based on its current position and the updated velocity.
The updating formulas for PSO algorithms are as follows:
```
v_i(t+1) = w * v_i(t) + c1 * r1 * (pbest_i - x_i(t)) + c2 * r2 * (gbest - x_i(t))
x_i(t+1) = x_i(t) + v_i(t+1)
```
Where:
- `v_i(t)`: The velocity of particle `i` at time `t`
- `v_i(t+1)`: The velocity of particle `i` at time `t+1`
- `w`: Inertia weight, used to balance exploration and exploitation
- `c1`: Cognitive learning factor, used to control the degree to which particles move towards their personal best positions
- `r1`: Random number, uniformly distributed
- `pbest_i`: The personal best position of particle `i`
- `x_i(t)`: The position of particle `i` at time `t`
- `x_i(t+1)`: The position of particle `i` at time `t+1`
- `c2`: Social learning factor, used to control the degree to which particles move towards the global best position
- `r2`: Random number, uniformly distributed
- `gbest`: The global best position
# 3.1 Function Design of the GA-PSO Algorithm
#### 3.1.1 Algorithm Initialization Function
```matlab
function [pop, params] = init_ga_pso(problem, params)
% Initialize GA-PSO algorithm
% Inputs:
% problem: Optimization problem
% params: Algorithm parameters
% Outputs:
% pop: Initialized population
% params: Updated algorithm parameters
% 1. Initialize population
pop = init_population(problem, params.pop_size);
% 2. Initialize particle swarm
params.particles = init_particles(problem, params.pop_size);
% 3. Update algorithm parameters
params.best_pos = get_best_pos(pop);
params.best_fit = get_best_fit(pop);
end
```
**Logical Analysis:**
* Initialize population: Calls the `init_population` function to initialize the population based on the problem and population size.
* Initialize particle swarm: Calls the `init_particles` function to initialize the particle swarm based on the problem and population size.
* Update algorithm parameters: Updates the best position and best fitness.
**Parameter Explanation:**
* `problem`: Optimization problem structure, containing problem information.
* `params`: Algorithm parameter structure, containing algorithm parameters.
* `pop`: Initialized population, each individual containing decision variables and fitness information.
* `particles`: Initialized particle swarm, each particle containing position, velocity, and fitness information.
* `best_pos`: Current best position.
* `best_fit`: Current best fitness.
#### 3.1.2 Algorithm Iteration Function
```matlab
function [pop, particles, params] = iterate_ga_pso(pop, particles, params)
% Iterate GA-PSO algorithm
% Inputs:
% pop: Current population
% particles: Current particle s
```
0
0
相关推荐
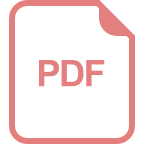
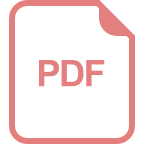
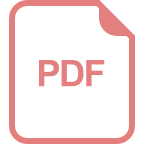
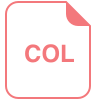
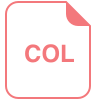
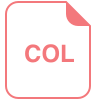
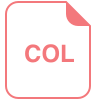
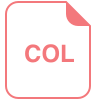
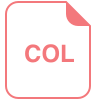
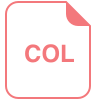