【Advanced Level】Implementing Genetic Algorithms with Matlab
发布时间: 2024-09-13 23:15:37 阅读量: 24 订阅数: 52 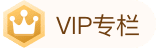
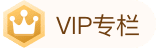
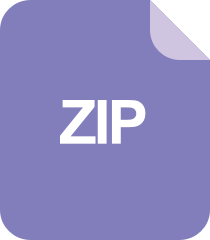
java计算器源码.zip
# [Advanced篇] Implementing Genetic Algorithms in Matlab
# 1. Theoretical Foundation of Genetic Algorithms
Genetic algorithms are heuristic search algorithms that mimic the biological evolution process to iteratively optimize complex problems. The fundamental principles include:
- **Population Initialization:** Randomly generate a set of candidate solutions, known as a population.
- **Fitness Function:** Evaluate the quality of each candidate solution and assign a fitness value.
- **Selection Operation:** Select superior candidate solutions for reproduction based on their fitness values.
# 2. Programming Implementation of Genetic Algorithms
### 2.1 Basic Process of Genetic Algorithms
The basic process of genetic algorithms includes the following steps:
#### 2.1.1 Initialize the Population
The population is a set of candidate solutions in genetic algorithms. During the initialization phase, each individual in the population is a randomly generated solution. The size of the population is usually determined by the size and complexity of the problem.
#### 2.1.2 Design of the Fitness Function
The fitness function is the standard for measuring the quality of individuals. It converts individuals into a numerical value, with higher values indicating better individuals. The design of the fitness function depends on the specific problem.
#### 2.1.3 Selection Operation
The selecti***mon selection methods include roulette wheel selection, tournament selection, and elitism selection.
### 2.2 Mutation and Crossover in Genetic Algorithms
#### 2.2.1 Mutation Operation
The mutation operation rand***mon mutation methods include gene mutation, gene swapping, and gene insertion.
#### 2.2.2 Cr***
***mon crossover methods include single-point crossover, two-point crossover, and uniform crossover.
### 2.3 Termination Conditions of Gen***
***mon termination conditions include:
#### 2.3.1 Reaching the Maximum Number of Generations
When the algorithm reaches the pre-set maximum number of generations, it stops.
#### 2.3.2 Achieving the Optimal Solution
When the algorithm finds a satisfactory optimal solution, it stops.
```
% Basic process of genetic algorithms
function [best_individual, best_fitness] = genetic_algorithm(problem, options)
% Initialize the population
population = initialize_population(problem, options.population_size);
% Evaluate population fitness
fitness = evaluate_fitness(population, problem);
% Iterative evolution
for generation = 1:options.max_generations
% Selection operation
selected_individuals = select_individuals(population, fitness, options.selection_method);
% Mutation operation
mutated_individuals = mutate_individuals(selected_individuals, options.mutation_rate);
% Crossover operation
crossed_individuals = crossover_individuals(selected_individuals, options.crossover_rate);
% New population
new_population = [mutated_individuals; crossed_individuals];
% Evaluate the fitness of the new population
new_fitness = evaluate_fitness(new_population, problem);
% Update the population
population = [population; new_population];
fitness = [fitness; new_fitness];
% Find the best individual
[best_fitness, best_index] = max(fitness);
best_individual = population(best_index, :);
% Termination condition
if best_fitness >= options.target_fitness || generation >= options.max_generations
break;
end
end
end
```
# 3. Practicing Genetic Algorithms in Matlab
### 3.1 Matlab Genetic Algorithm Toolbox
Matlab provides a powerful genetic algorithm toolbox that includes functions and classes for implementing gen
0
0