[Advanced] MATLAB Interpolation and Fitting Methods
发布时间: 2024-09-13 23:45:51 阅读量: 25 订阅数: 38 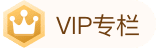
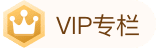
# 1. Overview of MATLAB Interpolation and Fitting
MATLAB interpolation and fitting are two powerful tools for dealing with irregularly sampled data. Interpolation is used to estimate values between data points, while fitting is used to find the best curve or surface to represent the data. MATLAB provides a wide range of interpolation and fitting functions, allowing engineers and scientists to handle complex datasets with ease and efficiency.
This chapter will introduce the basic concepts of interpolation and fitting, including their types, advantages and disadvantages, and implementation in MATLAB. We will focus on linear interpolation, polynomial interpolation, and spline interpolation, as well as fitting methods such as least squares, weighted least squares, and regularization methods.
# 2. Interpolation Methods
Interpolation is a technique for estimating unknown values between given data points. It is useful in many applications, such as signal processing, image processing, and data analysis. MATLAB offers various interpolation methods, including linear interpolation, polynomial interpolation, and spline interpolation.
### 2.1 Linear Interpolation
Linear interpolation is the simplest form of interpolation. It assumes a straight line between data points and uses this line to estimate the unknown values.
#### 2.1.1 One-dimensional Linear Interpolation
One-dimensional linear interpolation is used for interpolating one-dimensional data. It uses the following formula:
```matlab
y = y1 + (y2 - y1) * (x - x1) / (x2 - x1)
```
Where:
* `y` is the interpolated value
* `y1` and `y2` are the known values at data points `x1` and `x2`
* `x` is the value to be interpolated
**Code Block:**
```matlab
% One-dimensional linear interpolation
x = [0, 1, 2, 3];
y = [0, 2, 4, 6];
x_interp = 1.5;
y_interp = interp1(x, y, x_interp);
disp(y_interp); % Output the interpolated value
```
**Logical Analysis:**
This code block uses the `interp1` function to perform one-dimensional linear interpolation. The `x` and `y` arrays represent the data points and known values, respectively. `x_interp` is the value to be interpolated. The `y_interp` variable stores the interpolated value.
#### 2.1.2 Multidimensional Linear Interpolation
Multidimensional linear interpolation is used for interpolating multidimensional data. It uses the following formula:
```matlab
y = y1 + (y2 - y1) * (x - x1) / (x2 - x1) + (y3 - y1) * (z - z1) / (z2 - z1)
```
Where:
* `y` is the interpolated value
* `y1`, `y2`, `y3` are the known values at data points `(x1, z1)`, `(x2, z1)`, `(x1, z2)`
* `x` and `z` are the values to be interpolated
**Code Block:**
```matlab
% Multidimensional linear interpolation
x = [0, 1, 2];
y = [0, 1, 2];
z = [0, 2, 4];
data = [0, 2, 4; 1, 3, 5; 2, 4, 6];
x_interp = 1.5;
y_interp = 1.5;
z_interp = 1.5;
y_interp = interp2(x, y, z, data, x_interp, y_interp, z_interp);
disp(y_interp); % Output the interpolated value
```
**Logical Analysis:**
This code block uses the `interp2` function to perform multidimensional linear interpolation. The `x`, `y`, `z`, and `data` arrays represent the data points, known values, and multidimensional data, respectively. `x_interp`, `y_interp`, and `z_interp` are the values to be interpolated. The `y_interp` variable stores the interpolated value.
### 2.2 Polynomial Interpolation
Polynomial interpolation uses polynomials to estimate unknown values. It is more accurate than linear interpolation but comes with a higher computational cost.
#### 2.2.1 Lagrange Interpolation
Lagrange interpolation uses the following formula:
```matlab
y = Σ[i=1:n] y_i * L_i(x)
```
Where:
* `y` is the interpolated value
* `y_i` is the known value at data point `x_i`
* `L_i(x)` is the Lagrange basis function, defined as:
```matlab
L_i(x) = Π[j=1, j!=i] (x - x_j) / (x_i - x_j)
```
**Code Block:**
```matlab
% Lagrange interpolation
x = [0, 1, 2, 3];
y = [0, 2, 4, 6];
x_interp = 1.5;
y_interp = lagrange(x, y, x_interp);
disp(y_interp); % Output the interpolated value
```
**Logical Analysis:**
This code block uses the `lagrange` function to perform Lagrange interpolation. The `x` and `y` arrays represent the data points and known values, respectively. `x_interp` is the value to be interpolated. The `y
0
0
相关推荐
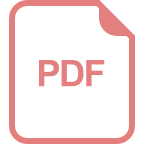
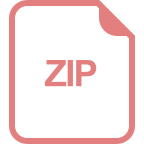
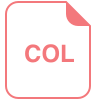
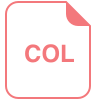
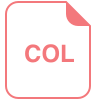
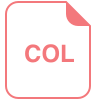
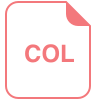
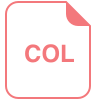