【Practical Exercise】Genetic Algorithm MATLAB Program for Multivariate Functions
发布时间: 2024-09-14 00:31:32 阅读量: 32 订阅数: 52 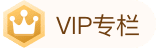
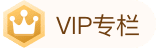
# 2.1 Fundamental Concepts of Genetic Algorithms
A Genetic Algorithm (GA) is an optimization algorithm inspired by the process of biological evolution. It tackles complex optimization problems by simulating natural selection and genetic mechanisms. The basic concepts of GA include:
- **Population:** A group of possible solutions, referred to as individuals.
- **Individual:** A potential solution represented by a set of genes.
- **Gene:** A single value representing a specific feature within an individual.
- **Fitness Function:** A function that evaluates the quality of an individual, where higher fitness indicates a better solution.
- **Selection:** Choosing individuals from the population for reproduction, with higher fitness individuals being more likely to be selected.
- **Crossover:** Combining the genes of two or more selected individuals to produce a new individual.
- **Mutation:** Randomly altering the genes of an individual to introduce diversity.
# 2. Genetic Algorithm Principles and MATLAB Implementation
## 2.1 Fundamental Concepts of Genetic Algorithms
Genetic Algorithms (GAs) are heuristic algorithms inspired by the theory of biological evolution, which simulate natural selection and genetic mechanisms to solve optimization problems. GAs iteratively search for the optimal solution through the following steps:
1. **Initialize Population:** Randomly generate a set of candidate solutions, known as a population.
2. **Evaluate Fitness:** Calculate the fitness of each solution, which is its degree of adaptation to the objective function.
3. **Selection:** Select the fittest individuals for reproduction based on their fitness.
4. **Crossover:** Combine two or more selected individuals to produce new individuals.
5. **Mutation:** Randomly modify certain features of the new individuals at a certain probability.
6. **Repeat Steps 2-5:** Until a termination condition is met (e.g., maximum iterations or fitness reaches a target value).
## 2.2 Genetic Algorithm MATLAB Implementation
### 2.2.1 Encoding and Decoding
Encoding represents solutions in the problem space as binary strings or other data structures. Decoding converts the encoded solutions back into actual solutions in the problem space.
**Code Block:**
```matlab
% Encoding function
function chromosome = encode(x)
% Convert real-number solutions to binary strings
chromosome = dec2bin(x);
end
% Decoding function
function x = decode(chromosome)
% Convert binary strings back to real-number solutions
x = bin2dec(chromosome);
end
```
### 2.2.2 Fitness Function Design
The fitness function measures the degree of adaptation of an individual, typically the negative value of the objective function.
**Code Block:**
```matlab
% Fitness function
function fitness = evaluate(x)
% Objective function
f = @(x) x^2;
% Calculate fitness
fitness = -f(x);
end
```
### 2.2.3 Selection Operator
The selection operator chooses the fittest individuals from the population for reproduction.
**Code Block:**
```matlab
% Roulette Wheel Selection
function selected = roulette_wheel_selection(population, fitness)
% Normalize fitness
fitness = fitness / sum(fitness);
% Randomly select an individual
r = rand();
for i = 1:length(population)
if r < sum(fitness(1:i))
selected = population(i);
break;
end
end
end
```
### 2.2.4 Crossover Operator
The crossover operator combines two or more selected individuals to produce new individuals.
**Code Block:**
```matlab
% Single-point Crossover
function offspring = single_point_crossover(parent1, parent2)
% Randomly select a crossover point
crossover_point = randi([1, length(parent1)]);
% Swap genes after the crossover point
offspring = [parent1(1:crossover_point), parent2(crossover_point+1:end)];
end
```
### 2.2.5 Mutation Operator
The mutation operator randomly modifie
0
0
相关推荐






