【Advanced】Machine Learning Implementation of Bayes Optimization for Machine Learning Models in MATLAB
发布时间: 2024-09-13 23:22:57 阅读量: 36 订阅数: 52 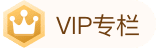
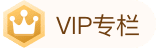
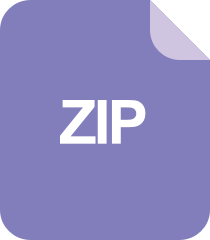
Machine Learning A Bayesian and Optimization Perspective
# 2.1 Implementing Bayesian Optimization in MATLAB
Within MATLAB, we can leverage the bayesopt function to implement the Bayesian optimization algorithm. This function accepts an objective function as input, which computes the target value to be optimized. The bayesopt function uses a Gaussian process as a surrogate model and employs sampling and optimization techniques to iteratively update the surrogate model and find the optimal value.
Parameters for the bayesopt function in MATLAB include:
- `AcquisitionFunctionName`: Specifies the acquisition function used to select the next sampling point.
- `MaxObjectiveEvaluations`: Specifies the maximum number of evaluations for the algorithm.
- `InitialPoints`: Specifies the initial sampling points for the algorithm.
- `AcquisitionParameters`: Specifies parameters for the acquisition function.
- `Verbose`: Specifies whether to display the algorithm's progress information.
# 2. Implementing Bayesian Optimization in MATLAB
### 2.1 Implementing Bayesian Optimization in MATLAB
In MATLAB, we can utilize the Bayesian Optimization Toolbox (bayesopt) to implement the Bayesian optimization algorithm. This toolbox provides various functions that enable us to easily set up, run, and evaluate Bayesian optimization algorithms.
The following code demonstrates how to implement the Bayesian optimization algorithm using the bayesopt toolbox:
```
% Define the objective function
objective = @(x) -x^2 + 10*sin(x);
% Define the search space
lb = -5;
ub = 5;
% Define parameters for the Bayesian optimization algorithm
acq_fn = 'ucb';
n_init_points = 5;
max_iter = 10;
% Create a Bayesian optimizer
optimizer = bayesopt(objective, lb, ub, acq_fn, n_init_points, max_iter);
% Run the Bayesian optimization algorithm
[x_opt, f_opt, ~, ~] = optimizer.optimize();
% Output the optimal solution
fprintf('Optimal solution: x = %.4f, f(x) = %.4f\n', x_opt, f_opt);
```
### 2.2 Setting Parameters for Bayesian Optimization
The performance of the Bayesian optimization algorithm largely depends on its parameter settings. The bayesopt toolbox allows us to customize the following parameters:
- **acq_fn:** The acquisition function used to choose the next point to evaluate.
- **n_init_points:** The number of initial sampling points.
- **max_iter:** The maximum number of iterations for the algorithm.
- **bounds:** The boundaries of the search space.
- **optimizer:** The optimizer used to optimize the acquisition function.
### 2.3 Evaluating the Performance of Bayesian Optimization
To evaluate the performance of the Bayesian optimization algorithm, we can use the following metrics:
- **Optimal solution:** The optimal solution found by the algorithm.
- **Convergence speed:** The time it takes for the algorithm to reach the optimal solution.
- **Robustness:** The algorithm's adaptability to different objective functions and search spaces.
We can assess these metrics by running the algorithm multiple times and comparing their performance.
# 3.1 Steps and Methods for Bayesian Optimization Hyperparameter Tuning
### Steps
Bayesian optimization hyperparameter tuning typically follows these steps:
1. **Define the objective function:** Clearly define the objective function to be optimized, which represents the performance metric of the model.
2. **Select the hyperparameter space:** Determine which hyperparameters of the model need to be optimized and their value ranges.
3. **Initialize the optimization process:** Specify the initial hyperparameter values and the parameters for the Bayesian optimization algorithm (such as acquisition functions, surrogate models, etc.).
4. **Iterative optimization:** In each iteration, the acquisition function selects the next hyperparameter combination to evaluate. Then, use the surrogate model to predict the performance of this combination and update the surrogate model.
5. **Termination condition:** Stop the optimization process when a predefined termination condition is met (such as the maximum number of iterations or convergence of the objective function).
### Methods
Bayesian optimization hyperparameter tuning involves the following key methods:
**Acquisition function:***mo
0
0
相关推荐







