【Advanced】GAN Adversarial Network Simulation for Generating Gaussian Distribution in Matlab
发布时间: 2024-09-13 23:14:17 阅读量: 29 订阅数: 52 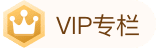
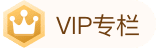
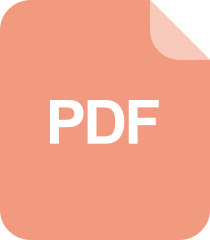
Generative Adversarial Network (GAN)
# **【Advanced篇】GAN Adversarial Network Simulation for Generating Gaussian Distribution in Matlab**
# 1. Introduction to Gaussian Distribution and Generation Methods**
The Gaussian distribution, also known as the normal distribution, is a common continuous probability distribution characterized by its probability density function:
```
f(x) = (1 / (σ√(2π))) * e^(-(x - μ)² / (2σ²))
```
Where μ represents the mean, and σ represents the standard deviation.
There are various methods to generate Gaussian distribution data, one of which is using a normal distribution random number generator. In Python, the `numpy.random.normal()` function can be used to generate normal distribution random numbers:
```python
import numpy as np
# Generate normal distribution random numbers with a mean of 0 and a standard deviation of 1
data = np.random.normal(0, 1, 1000)
```
# 2. Principles and Applications of Generative Adversarial Networks (GAN)
### 2.1 Architecture and Working Principles of GAN
A Generative Adversarial Network (GAN) is a type of generative adversarial network composed of a generator network and a discriminator network. The generator network is responsible for producing data, while the discriminator network distinguishes between generated data and real data. The training process for a GAN is adversarial; the generator network continuously improves the quality of the data it generates, while the discriminator network continuously improves its ability to distinguish.
**Generator Network:** The generator network is a neural network that generates data from noise or other random inputs. The goal of the generator network is to produce fake data that is similar to the real data distribution.
**Discriminator Network:** The discriminator network is also a neural network that distinguishes between data from the real data and generated data. The goal of the discriminator network is to maximize the accuracy of identifying real and generated data.
The GAN training process is as follows:
1. **Initialization:** Initialize the generator network and discriminator network.
2. **Training:** Alternately train the generator network and the discriminator network.
3. **Generator Network Training:** Fix the generator network and train the discriminator network to distinguish between real and generated data.
4. **Discriminator Network Training:** Fix the discriminator network and train the generator network to produce fake data that is similar to the real data distribution.
5. **Repeat Steps 3 and 4:** Repeat steps 3 and 4 until the generator network can produce fake data that is similar to the real data distribution.
### 2.2 GAN Training Methods and Optimization Strategies
GAN training is a challenging process because it involves training two adversarial networks. Here are some common GAN training methods and optimization strategies:
**Generator Network Loss Function:** The generator network's loss function is usually the output of the discriminator network. The generator network's goal is to maximize the probability that the discriminator network mistakes generated data for real data.
**Discriminator Network Loss Function:** The discriminator network's loss function is usually the cross-entropy loss between real and generated data. The discriminator network's goal is to maximize the accuracy of distinguishing between real and generated data.
**Optimization Algorithm:** GAN training typically uses optimization algorithms such as Adam or RMSProp. These algorithms can effectively handle the adversarial training process of GANs.
**Hyperparameter Tuning:** GAN training requires careful adjustment of hyperparameters, such as learning rate, batch size, and the number of training iterations. Hyperparameter tuning can significantly affect the training results of GANs.
**Code Block:**
```python
import torch
import torch.nn as nn
import torch.optim as optim
# Generator Network
class Generator(nn.Module):
def __init__(self):
super(Generator, self).__init__()
# ...
def forward(self, z):
# ...
# Discriminator Network
class Discriminator(nn.Module):
def __init__(self):
super(Discriminator, self).__init__()
# ...
def forward(self, x):
# ...
# Loss Functions
generator_loss = nn.BCELoss()
discriminator_loss = nn.BCELoss()
# Optimizers
generator_optimizer = optim.Adam(generator.parameters(), lr=0.0002)
discriminator_optimizer = optim.Adam(discriminator.parameters(), lr=0.0002)
# Training
for epoch in range(100):
# ...
```
**Logical Analysis:**
This code implements the GAN training pr
0
0
相关推荐






