[Advanced] Source Code for Decision Tree Classification in MATLAB
发布时间: 2024-09-13 23:03:35 阅读量: 31 订阅数: 52 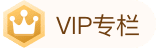
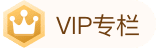
# [Advanced Series] Source Code of Decision Tree Classification in MATLAB
## 1. Overview of Decision Tree Classification
A decision tree is a machine learning algorithm used to solve classification problems. It represents the decision-making process in a tree-like structure where each internal node represents a feature, and each leaf node represents a classification outcome. The advantages of decision tree classification include:
* Strong interpretability: Decision tree models are easy to understand and can visually demonstrate the decision-making process.
* Good robustness: Decision trees are insensitive to missing and outlier values and can handle noisy data.
* Efficient computation: The training and prediction process of decision tree models is relatively efficient, making them suitable for handling large datasets.
## 2. Decision Tree Classification in MATLAB
### 2.1 Fundamental Principles of Decision Tree Models
A decision tree is a machine learning algorithm that classifies data points or predicts target variables through a series of rules. A decision tree model consists of nodes and leaf nodes, where:
- A **node** represents a decision point, dividing data points into different subsets based on a feature.
- A **leaf node** represents the termination point of the decision tree, containing the final classification or prediction result.
The construction process of a decision tree is as follows:
1. **Select the root node:** Choose a feature from the training data that best differentiates between different classes.
2. **Split the data:** Divide the data points into different subsets based on the values of the root node feature.
3. **Recursive construction:** Repeat steps 1 and 2 for each subset until all data points are classified or predicted.
### 2.2 Implementation of Decision Tree Classifiers in MATLAB
MATLAB provides the `fitctree` function for constructing decision tree classifiers. This function accepts the following parameters:
```matlab
fitctree(X, Y, 'PredictorNames', predictorNames, 'ResponseName', responseName, 'MaxNumSplits', maxNumSplits, 'MinLeafSize', minLeafSize)
```
Where:
- `X`: Feature matrix, each row represents a data point, and each column represents a feature.
- `Y`: Target variable vector, representing the class of each data point.
- `PredictorNames`: Optional cell array of feature names.
- `ResponseName`: Optional string of the target variable name.
- `MaxNumSplits`: Maximum number of splits to limit the depth of the decision tree.
- `MinLeafSize`: Minimum number of data points allowed in a leaf node.
#### 2.2.1 Usage of the `fitctree` Function
The following code demonstrates how to use the `fitctree` function to build a decision tree classifier:
```matlab
% Import data
data = readtable('data.csv');
% Feature matrix and target variable vector
X = data{:, 1:end-1};
Y = data{:, end};
% Build a decision tree classifier
tree = fitctree(X, Y);
% Predict new data
newData = [10, 20, 30];
prediction = predict(tree, newData);
```
#### 2.2.2 Optimization of Decision Tree Parameters
The `fitctree` ***mon parameter optimization methods include:
- **Cross-validation:** Divide the data into training and testing sets, construct decision trees multiple times, and evaluate their performance.
- **Grid search:** Traverse a grid of parameter values to select the best-performing combination.
- **Bayesian optimization:** Use a Bayesian optimiza
0
0
相关推荐







