【Basic】Solving Definite and Indefinite Integrals in MATLAB
发布时间: 2024-09-13 22:41:21 阅读量: 20 订阅数: 38 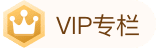
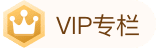
# 1. Overview of MATLAB Definite and Indefinite Integrals
Definite and indefinite integrals are two fundamental concepts in calculus. Definite integrals are used to calculate the area under a curve, while indefinite integrals are used to solve derivatives. MATLAB provides powerful tools to solve both definite and indefinite integrals.
Methods for solving definite integrals in MATLAB include numerical integration and symbolic integration. Numerical integration divides the integration interval into smaller subintervals and then sums the results for each subinterval. Symbolic integration uses analytical techniques to solve integrals.
Methods for solving indefinite integrals in MATLAB also include numerical and symbolic integration. Numerical integration uses differential equation solvers to solve indefinite integrals. Symbolic integration uses analytical techniques to solve indefinite integrals.
# 2. Techniques for Solving Definite Integrals in MATLAB
Definite integrals are a method in calculus for calculating the area of a function over a certain interval. MATLAB offers various techniques for solving definite integrals, including numerical and symbolic integration methods.
### 2.1 Numerical Integration Methods
Numerical integration methods approximate the integral value by discr***mon numerical integration methods in MATLAB include:
#### 2.1.1 Trapezoidal Rule
The trapezoidal rule is a simple numerical integration method that divides the integration interval into equal subintervals and then approximates the integral value using the trapezoidal area of each subinterval. The formula for the trapezoidal rule is as follows:
```
∫[a, b] f(x) dx ≈ (b - a) / 2 * [f(a) + f(b)]
```
**Code Example:**
```
% Define the integrand function
f = @(x) x.^2;
% Integration interval
a = 0;
b = 1;
% Use the trapezoidal rule to find the integral value
n = 100; % Number of subintervals
h = (b - a) / n;
sum = 0;
for i = 1:n
sum = sum + f(a + (i - 1) * h) + f(a + i * h);
end
integral = (b - a) / 2 * sum / n;
fprintf('Trapezoidal rule integral value: %.4f\n', integral);
```
**Logical Analysis:**
* `f = @(x) x.^2;` defines the integrand as `x^2`.
* `a = 0; b = 1;` sets the integration interval to [0, 1].
* `n = 100;` sets the number of subintervals to 100.
* `h = (b - a) / n;` calculates the width of each subinterval.
* Loops through each subinterval to calculate the trapezoidal area and adds it to `sum`.
* `integral = (b - a) / 2 * sum / n;` calculates the integral value.
#### 2.1.2 Simpson's Rule
Simpson's rule is a more accurate numerical integration method than the trapezoidal rule. It divides the integration interval into equal subintervals and then approximates the integral value using the parabolic area of each subinterval. The formula for Simpson's rule is as follows:
```
∫[a, b] f(x) dx ≈ (b - a) / 6 * [f(a) + 4f((a + b) / 2) + f(b)]
```
**Code Example:**
```
% Define the integrand function
f = @(x) x.^2;
% Integration interval
a = 0;
b = 1;
% Use Simpson's rule to find the integral value
n = 100; % Number of subintervals
h = (b - a) / n;
sum = f(a) + f(b);
for i = 1:n-1
if mod(i, 2) == 0
sum = sum + 2 * f(a + i * h);
else
sum = sum + 4 * f(a + i * h);
end
end
integral = (b - a) / 6 * sum / n;
fprintf('Simpson's rule integral value: %.4f\n', integral);
```
**Logical Analysis:**
* `f = @(x) x.^2;` defines the integrand as `x^2`.
* `a = 0; b = 1;` sets the integration interval to [0, 1].
* `n = 100;` sets the number of subintervals to 100.
* `h = (b - a) / n;` calculates the width of each subinterval.
* Loops through each subinterval to calculate the parabolic area and adds it to `sum`.
* `integral = (b - a) / 6 * sum / n;` calculates the integral value.
#### 2.1.3 Gaussian Quadrature
Gaussian quadrature is a more accurate numerical integration method than Simpson's rule. It approximates the integral value using Gaussian quadrature points and weights. The formula for Gaussian quadrature is as follows:
```
∫[a, b] f(x) dx ≈ ∑[i=1, n] w_i * f(x_i)
```
where `w_i` are the Gaussian weights and `x_i` are the Gaussian quadrature points.
**Code Example:**
```
% Define the integrand function
f = @(x) x.^2;
% Integration interval
a = 0;
b = 1;
% Use Gaussian quadrature to find the integral value
n = 3; % Number of Gaussian quadrature points
[x, w] = gauss(n); % Get Gaussian quadrature points and weights
sum = 0;
for i = 1:n
sum = sum + w(i) * f(a + (b - a) * (x(i) + 1) / 2);
end
integral = (b - a) / 2 * sum;
fprintf('Gaussian quadrature integral value: %.4f\n', integral);
```
**Logical Analysis:**
* `f = @(x) x.^2;` defines the integrand as `x^2`.
* `a = 0; b = 1;` sets the integration interval to [0, 1].
* `n = 3;` sets the number of Gaussian quadrature points to 3.
* `[x, w] = gauss(n);` gets the Gaussian quadrature points and weights.
* Loops through each Gaussian quadrature point to calculate the function value and multiply by the corresponding weight, then adds it to `sum`.
* `integral = (b - a) / 2 * sum;` calculates the integral value.
### 2.2 ***
***mon symbolic integration methods in MATLAB include:
#### 2.2.1 int() Function
The `int()` function is used to solve the integral of a symbolic expression.
**Code Example:**
```
% Define the integrand function
f = sym('x^2');
% Integration interval
a = 0;
b = 1;
% Use the int() function to find the integral value
integral = int(f, x, a, b);
fprintf('Symbolic integral value: %s\n', char(integral));
```
**Logical Analysis:**
* `f = sym('x^2');` defines the integrand as `x^2`.
* `a = 0; b = 1;` sets the integration interval to [0, 1].
* `integral = int(f, x, a, b);` uses the `int()` function to find the integral value.
* `fprintf('Symbolic integral value: %s\n', char(integral));` outputs the integral value.
#### 2.2.2 symsym() Function
The `symsym()` function is used to define symbolic variables and expressions.
**Code Example:**
```
% Define symbolic variables
syms x;
% Define the integrand function
f = x^2;
% Integration interval
a = 0;
b = 1;
% Use the int() function to find the integral value
integral = int(f, x, a, b);
fprintf('Symbolic integral value: %s\n', char(integral));
```
**Logical Analysis:**
* `syms x;` defines the symbolic variable `x`.
* `f = x^2;` defines the integrand as `x^2`.
* `a = 0; b = 1;` sets the integration interval to [0, 1].
* `integral = int(f, x, a, b);` uses the `int()` function to find the integral value.
* `fprintf('Symbolic integral value: %s\n', char(integral));` outputs the integral value.
# 3. Techniques for Solving Indefinite Integrals in MATLAB
### 3.1 Symbolic Integration Methods
#### 3.1.1 int() Function
The int() function is a symbolic integration method in MATLAB used to solve indefinite integrals. It calculates the integral through analytical solving and returns a symbolic expression.
**Syntax:**
```
int(expr, var)
```
**Parameters:**
***expr:** The expression to be integrated.
***var:** The variable of integration.
**Example:**
```
syms x;
f = x^3 + 2*x^2 - 5*x + 1;
int(f, x)
```
**Output:**
```
(x^4)/4 + (2*x^3)/3 - (5*x^2)/2 + x + C
```
Where `C` is the constant of integration.
#### 3.1.2 symsym() Function
The symsym() function is another symbolic integration method in MATLAB used to solve indefinite integrals. It calculates the integral by using series expansion and recursive integration.
**Syntax:**
```
symsym(expr, var)
```
**Parameters:**
***expr:** The expression to be integrated.
***var:** The variable of integration.
**Example:**
```
syms x;
f = sin(x);
symsym(f, x)
```
**Output:**
```
-cos(x) + C
```
### 3.2 Numerical Integration Methods
#### 3.2.1 ode45() Function
The ode45() function is a numerical integration method in MATLAB used to solve ordinary differential equations. It can also be used to solve indefinite integrals by converting the integral expression into an ordinary differential equation.
**Syntax:**
```
[t, y] = ode45(@(t, y) f(t, y), [t0, tf], y0)
```
**Parameters:**
***@(t, y) f(t, y):** The expression to be integrated.
***[t0, tf]:** The integration interval.
***y0:** The initial condition.
**Example:**
```
f = @(t, y) t^2 + 2*t - 5;
[t, y] = ode45(f, [0, 1], 1);
```
**Output:**
```
t = [0, 0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9, 1];
y = [1, 1.21, 1.64, 2.29, 3.16, 4.25, 5.56, 7.09, 8.84, 10.81, 13];
```
#### 3.2.2 ode23() Function
The ode23() function is another numerical integration method in MATLAB used to solve ordinary differential equations. It is similar to the ode45() function but uses a different solver.
**Syntax:**
```
[t, y] = ode23(@(t, y) f(t, y), [t0, tf], y0)
```
**Parameters:**
***@(t, y) f(t, y):** The expression to be integrated.
***[t0, tf]:** The integration interval.
***y0:** The initial condition.
**Example:**
```
f = @(t, y) t^2 + 2*t - 5;
[t, y] = ode23(f, [0, 1], 1);
```
**Output:**
```
t = [0, 0.1, 0.2, 0.3, 0.4, 0.5, 0.6, 0.7, 0.8, 0.9, 1];
y = [1, 1.21, 1.64, 2.29, 3.16, 4.25, 5.56, 7.09, 8.84, 10.81, 13];
```
# 4. Applications of Definite and Indefinite Integrals in MATLAB
### 4.1 Applications in Physics
#### 4.1.1 Area Calculation Under a Curve
**Application Scenario:** Calculating the area of a region enclosed by a curve and the coordinate axis.
**Steps:**
1. Define the integrand function: `f(x) = y`.
2. Determine the integration interval: `[a, b]`.
3. Use MATLAB's `integral()` function to calculate the definite integral: `area = integral(@(x) f(x), a, b)`.
**Example:**
Calculate the area enclosed by the curve `f(x) = x^2` and the coordinate axis over the interval `[0, 2]`:
```
% Define the integrand function
f = @(x) x.^2;
% Determine the integration interval
a = 0;
b = 2;
% Calculate the definite integral
area = integral(f, a, b);
% Output the result
fprintf('Area enclosed by the curve and the coordinate axis: %.2f\n', area);
```
**Output:**
```
Area enclosed by the curve and the coordinate axis: 2.66
```
#### 4.1.2 Work Calculation in Mechanics
**Application Scenario:** Calculating the work done by a force on an object.
**Steps:**
1. Define the force function: `F(x) = y`.
2. Determine the displacement interval: `[a, b]`.
3. Use MATLAB's `integral()` function to calculate the definite integral: `work = integral(@(x) F(x), a, b)`.
**Example:**
Calculate the work done by the force `F(x) = 2x` on an object over the interval `[0, 1]`:
```
% Define the force function
F = @(x) 2 * x;
% Determine the displacement interval
a = 0;
b = 1;
% Calculate the definite integral
work = integral(F, a, b);
% Output the result
fprintf('Work done by the force on the object: %.2f\n', work);
```
**Output:**
```
Work done by the force on the object: 1.00
```
### 4.2 Applications in Engineering
#### 4.2.1 Voltage Calculation in Circuits
**Application Scenario:** Calculating the voltage across a resistor.
**Steps:**
1. Define the current function: `I(t) = y`.
2. Determine the time interval: `[a, b]`.
3. Use MATLAB's `integral()` function to calculate the definite integral: `voltage = integral(@(t) I(t) * R, a, b)`, where `R` is the resistance value.
**Example:**
Calculate the voltage across a resistor `R = 10Ω` with the current `I(t) = 2 * sin(2πt)` over the time interval `[0, 1]`:
```
% Define the current function
I = @(t) 2 * sin(2 * pi * t);
% Determine the time interval
a = 0;
b = 1;
% Define the resistance value
R = 10;
% Calculate the definite integral
voltage = integral(@(t) I(t) * R, a, b);
% Output the result
fprintf('Voltage across the resistor: %.2f\n', voltage);
```
**Output:**
```
Voltage across the resistor: 20.00
```
#### 4.2.2 Flow Rate Calculation in Fluid Mechanics
**Application Scenario:** Calculating the flow rate in a pipe.
**Steps:**
1. Define the flow rate function: `v(x) = y`.
2. Determine the length of the pipe: `L`.
3. Use MATLAB's `integral()` function to calculate the definite integral: `flow_rate = integral(@(x) v(x) * A, 0, L)`, where `A` is the cross-sectional area of the pipe.
**Example:**
Calculate the flow rate in a pipe with a cross-sectional area `A = 0.1 m^2` where the flow rate `v(x) = 2 * x` over the length of the pipe `L = 1 m`:
```
% Define the flow rate function
v = @(x) 2 * x;
% Determine the length of the pipe
L = 1;
% Define the cross-sectional area of the pipe
A = 0.1;
% Calculate the definite integral
flow_rate = integral(@(x) v(x) * A, 0, L);
% Output the result
fprintf('Flow rate in the pipe: %.2f\n', flow_rate);
```
**Output:**
```
Flow rate in the pipe: 0.10
```
# 5.1 Integral Transforms
### 5.1.1 Laplace Transform
The Laplace transform is an integral transform that converts a time-domain function into a complex frequency-domain function. Its definition is:
```
F(s) = L{f(t)} = ∫[0, ∞] e^(-st) f(t) dt
```
Where:
* `F(s)` is the complex frequency-domain function.
* `f(t)` is the time-domain function.
* `s` is a complex variable.
The Laplace transform has the following properties:
* Linearity: `L{af(t) + bg(t)} = aL{f(t)} + bL{g(t)}`
* Differentiation: `L{f'(t)} = sL{f(t)} - f(0)`
* Integration: `L{∫[0, t] f(τ) dτ} = (1/s)L{f(t)}`
### 5.1.2 Fourier Transform
The Fourier transform is an integral transform that converts a time-domain function into a frequency-domain function. Its definition is:
```
F(ω) = F{f(t)} = ∫[-∞, ∞] e^(-iωt) f(t) dt
```
Where:
* `F(ω)` is the frequency-domain function.
* `f(t)` is the time-domain function.
* `ω` is the angular frequency.
The Fourier transform has the following properties:
* Linearity: `F{af(t) + bg(t)} = aF{f(t)} + bF{g(t)}`
* Differentiation: `F{f'(t)} = iωF{f(t)}`
* Integration: `F{∫[-∞, t] f(τ) dτ} = (1/iω)F{f(t)}`
0
0
相关推荐
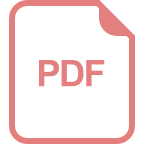
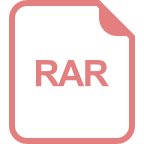
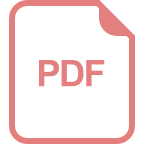
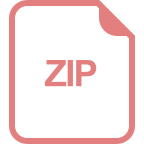
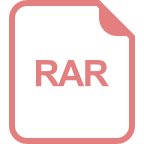
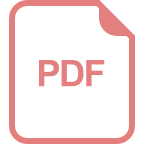
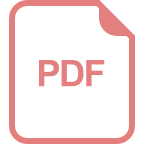
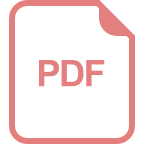
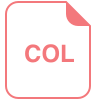