[Advanced Chapter] Solving Nonlinear Equations: Broyden and Secant Methods in MATLAB
发布时间: 2024-09-13 23:52:49 阅读量: 32 订阅数: 38 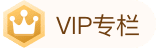
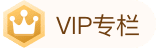
# **Advanced篇** Nonlinear Equation Solving: Broyden and Secant Methods in MATLAB
# 1. Overview of Nonlinear Equation Solving**
A nonlinear equation is one that takes the form of f(x) = 0, where f(x) is nonlinear. Solving nonlinear equations is a fundamental problem in scientific computing and has wide-ranging applications in fields such as engineering, finance, and physics.
The methods for solving nonlinear equations can be broadly classified into two categories: direct methods and iterative methods. Direct methods yield the solution of the equation in one step but are often impractical for high-dimensional nonlinear equations. Iterative methods, which involve approximating the solution iteratively, exhibit better convergence properties and are the primary means for solving nonlinear equations.
# 2. Broyden Method
### 2.1 Principle of the Broyden Method
The Broyden method is a quasi-Newton method used for solving systems of nonlinear equations. It accelerates convergence by approximating the Hessian matrix.
#### 2.1.1 Broyden Update Formula
The Broyden method employs the following update formula to refresh the approximate Hessian matrix B:
```
B_{k+1} = B_k - \frac{B_k s_k y_k^T B_k}{s_k^T B_k s_k} + \frac{y_k y_k^T}{s_k^T y_k}
```
Where:
* B_k is the approximate Hessian matrix at the k-th iteration.
* s_k = x_{k+1} - x_k is the step size at the k-th iteration.
* y_k = f(x_{k+1}) - f(x_k) is the difference in function values at the k-th iteration.
#### 2.1.2 Convergence Analysis
The Broyden method exhibits quadratic convergence under the following conditions:
* The objective function f(x) is continuously differentiable.
* The initial approximate Hessian matrix B_0 is positive definite.
* The step size s_k satisfies the Wolfe conditions.
### 2.2 Implementation of the Broyden Method in MATLAB
#### 2.2.1 MATLAB Code for the Broyden Method
```matlab
function [x, iter] = broyden(f, x0, tol, max_iter)
% Initialization
x = x0;
B = eye(length(x0));
iter = 0;
% Iterative solution
while norm(f(x)) > tol && iter < max_iter
% Compute gradient and approximate Hessian matrix
g = grad(f, x);
s = -B \ g;
% Update x and B
x_next = x + s;
y = f(x_next) - f(x);
B = B - (B * s * y' * B) / (s' * B * s) + (y * y') / (s' * y);
% Update iteration count
iter = iter + 1;
% Update x
x = x_next;
end
end
```
#### 2.2.2 Application Example of the Broyden Method
```matlab
% Define the objective function
f = @(x) x^3 - 2*x^2 + 1;
% Initial point
x0 = 1;
% Tolerance
tol = 1e-6;
% Maximum number of iterations
max_iter = 100;
% Solve
[x, iter] = broyden(f, x0, tol, max_iter);
% Output results
fprintf('Solution is: %.6f\n', x);
fprintf('Number of iterations: %d\n', iter);
```
# 3. Secant Method
### 3.1 Principle of the Secant Method
The Secant method is a one-dimensional method for solving nonlinear equations. It is based on the idea of Newton's method but, unlike Newton's method, does not require the computation of derivatives. The Secant method uses the function values at the last two iteration points to approximate the derivative, then uses this approximate derivative for iterative solution.
#### 3.1.1 Secant Method Iteration Formula
The Secant method iteration formula is as follows:
```
x_{n+1} = x_n - f(x_n) * (x_n - x_{n-1}) / (f(x_n) - f(x_{n-1}))
```
Where:
* $x_n$ is the approximate solution at the n-th iteration.
* $f(x)$ is the objective function.
#### 3.1.2 Convergence Analysis
The convergence properties of the Secant method are similar to Newton's method and can converge quadratically under certain conditions. Specifically, when the objective function $f(x)$ satisfies the Lipschitz continuity condition near the approximate solution $x_n$, the Secant method can achieve quadratic convergence.
### 3.2 Implementation of the Secant Method in MATLAB
#### 3.2.1 MATLAB Code for the Secant Method
```matlab
function root = secant(f, x0, x1, tol, max_iter)
% Secant method for finding the root of a nonlinear equation
%
% Input:
% f: the nonlinear function
% x0, x1: initial guesses
% tol: tolerance for
```
0
0
相关推荐
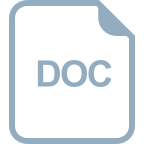
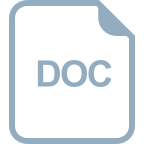
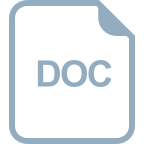
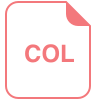
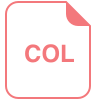
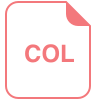
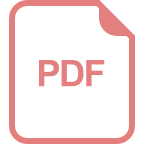
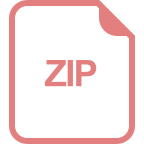
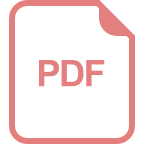