ode45 Solving Differential Equations: The Insider's Guide to Decision Making and Optimization, Mastering 5 Key Steps
发布时间: 2024-09-15 06:15:33 阅读量: 34 订阅数: 32 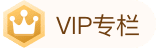
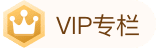
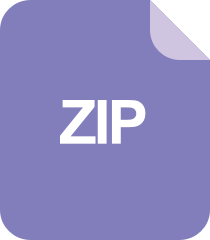
YOLO算法-城市电杆数据集-496张图像带标签-电杆.zip
# The Secret to Solving Differential Equations with ode45: Mastering 5 Key Steps
Differential equations are mathematical models that describe various processes of change in fields such as physics, chemistry, and biology. The ode45 solver in MATLAB is used for solving systems of ordinary differential equations, employing the Runge-Kutta method for its high precision and stability.
The basic syntax of the ode45 solver is as follows:
```matlab
[t, y] = ode45(@odefun, tspan, y0)
```
Where:
* `@odefun`: A function handle for the differential equation, which takes time `t` and state `y` as inputs and returns the derivatives of the differential equation.
* `tspan`: The time interval for the solution, which is a vector containing the start and end times.
* `y0`: The initial condition, a vector containing the initial values of all state variables in the system of equations.
# Theoretical Foundation of Solving Differential Equations with ode45
### 2.1 Basic Concepts of Differential Equations
A differential equation is an equation that describes the relationship between an unknown function and its derivatives. It has extensive applications in science, engineering, and finance. The general form of a differential equation is:
```
y' = f(x, y)
```
Where:
* y is the unknown function
* x is the independent variable
* f is a known function
The order of a differential equation refers to the order of the highest derivative. A first-order differential equation is the simplest type and has the form:
```
y' = f(x, y)
```
A second-order differential equation has the form:
```
y'' = f(x, y, y')
```
### 2.2 The Principle of the ode45 Solver
The ode45 solver in MATLAB is designed to solve first and second-order ordinary differential equations. It uses the Runge-Kutta method, a single-step method that approximates the solution of a differential equation.
The Runge-Kutta method follows these steps to solve a differential equation:
1. Given initial conditions y(x0) and step size h, calculate k1, k2, k3, and k4:
```
k1 = h * f(x0, y0)
k2 = h * f(x0 + h/2, y0 + k1/2)
k3 = h * f(x0 + h/2, y0 + k2/2)
k4 = h * f(x0 + h, y0 + k3)
```
2. Update y using k1, k2, k3, and k4:
```
y(x0 + h) = y0 + (k1 + 2*k2 + 2*k3 + k4) / 6
```
3. Repeat steps 1 and 2 until the final value is reached.
### 2.3 Parameter Settings of the ode45 Solver
The ode45 solver has several parameters that can be adjusted to control the solving process. These include:
***RelTol**: Relative tolerance. ode45 will attempt to keep the local error within RelTol * |y| * h, where y is the solution and h is the step size.
***AbsTol**: Absolute tolerance. ode45 will try to keep the local error below AbsTol.
***MaxStep**: The maximum step size. ode45 will not use a step size larger than MaxStep.
***InitialStep**: The initial step size. ode45 will begin solving with this step size.
The default values of these parameters usually yield good results. However, in certain cases, it may be necessary to adjust these parameters to achieve better accuracy or performance.
# Practical Application of Solving Differential Equations with ode45
### 3.1 Solving Linear Differential Equations
Linear differential equations are an important class of differential equations, having the form:
```
y' + p(x)y = q(x)
```
Where y is the unknown function and p(x) and q(x) are known functions.
The ode45 solver can easily solve linear differential equations. The following code demonstrates how to use ode45 to solve a simple linear differential equation:
```python
import numpy as np
from scipy.integrate import odeint
def f(y, x):
return -y + np.sin(x)
y0 = 1
x = np.linspace(0, 10, 100)
sol = odeint(f, y0, x)
```
Code logic analysis:
* The `f(y, x)` function defines the right-hand side of the differential equation.
* `y0` is the initial condition.
* `x` is the range of x values to solve for.
* `sol` is the result of the solution, an array of y values.
### 3.2 Solving Nonlinear Differential Equations
Nonlinear differential equations are a more complex type of differential equation, taking the form:
```
y' = f(x, y)
```
Where f(x, y) is a nonlinear function.
The ode45 solver can also solve nonlinear differential equations. The following code demonstrates how to use ode45 to solve a simple nonlinear differential equation:
```python
import numpy as np
from scipy.integrate import odeint
def f(y, x):
return y * (1 - y)
y0 = 0.5
x = np.linspace(0, 10, 100)
sol = odeint(f, y0, x)
```
Code logic analysis:
* The `f(y, x)` function defines the right-hand side of the differential equation.
* `y0` is the initial condition.
* `x` is the range of x values to solve for.
* `sol` is the result of the solution, an array of y values.
### 3.3 Solving Boundary Value Problems
Boundary value problems are a special class of differential equation problems where the unknown function has specified values at the boundaries. The ode45 solver can also solve boundary value problems. The following code demonstrates how to use ode45 to solve a simple boundary
0
0
相关推荐
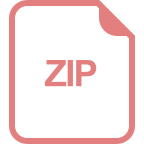
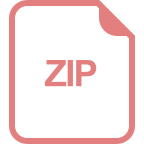
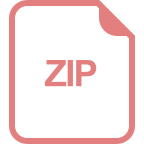
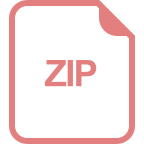