Solving Differential Equations with ode45: The Key to Simulation and Modeling, Mastering 5 Critical Applications
发布时间: 2024-09-15 06:12:15 阅读量: 38 订阅数: 40 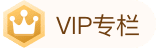
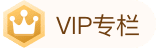
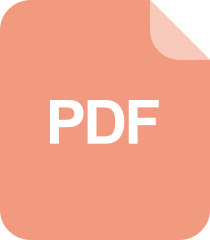
Ordinary differential equations and dynamical systems-G. Teschl
# 1. The Principle and Implementation of Solving Differential Equations with ode45: A Secret Weapon in Simulation and Modeling, Mastering 5 Key Applications
## 1.1 Introduction to Differential Equations
Differential equations describe the relationship between unknown functions and their derivatives. They are widely used in science, engineering, and mathematics to model various physical phenomena, such as motion, heat transfer, and chemical reactions.
## 1.2 The ode45 Solver
ode45 is a solver in MATLAB for solving systems of ordinary differential equations. It is based on the explicit Runge-Kutta method, a single-step solver that approximates solutions through iterative updates. ode45 balances accuracy and efficiency by controlling step size and order, providing good performance in most cases.
# 2. Applications of ode45 in Simulation
## 2.1 Mechanical System Simulation
### 2.1.1 Spring Oscillator Simulation
The spring oscillator is a classic mechanical system consisting of a spring and a mass attached to it. When the mass is stretched or compressed, the spring applies a restoring force, causing the mass to oscillate.
ode45 can simulate its behavior by solving the motion equations of the spring oscillator. The motion equations are:
```python
def spring_oscillator(t, y):
"""Spring oscillator motion equations.
Args:
t (float): Time.
y (list): State variables [position, velocity].
Returns:
list: Derivatives of state variables [velocity, acceleration].
"""
m = 1 # mass
k = 1 # spring constant
dydt = [y[1], -k/m * y[0]]
return dydt
```
Where `t` is time, `y` is the state variable, including position and velocity. `m` is the mass, and `k` is the spring constant.
```python
import numpy as np
import matplotlib.pyplot as plt
# Initial conditions
y0 = [0.5, 0] # Initial position and velocity
# Solve motion equations
t_span = np.linspace(0, 10, 1000) # Time range
sol = ode45(spring_oscillator, t_span, y0)
# Plot results
plt.plot(sol.t, sol.y[0, :])
plt.xlabel('Time (s)')
plt.ylabel('Position (m)')
plt.show()
```
### 2.1.2 Particle Motion Simulation
Particle motion simulation involves solving the motion trajectory of a particle under a given force field. ode45 can simulate its behavior by solving the motion equations of the particle. The motion equations are:
```python
def particle_motion(t, y):
"""Particle motion equations.
Args:
t (float): Time.
y (list): State variables [position, velocity].
Returns:
list: Derivatives of state variables [velocity, acceleration].
"""
g = 9.81 # Gravitational acceleration
dydt = [y[1], -g]
return dydt
```
Where `t` is time, `y` is the state variable, including position and velocity. `g` is the gravitational acceleration.
```python
import numpy as np
import matplotlib.pyplot as plt
# Initial conditions
y0 = [0, 0] # Initial position and velocity
# Solve motion equations
t_span = np.linspace(0, 10, 1000) # Time range
sol = ode45(particle_motion, t_span, y0)
# Plot results
plt.plot(sol.t, sol.y[0, :])
plt.xlabel('Time (s)')
plt.ylabel('Height (m)')
plt.show()
```
## 2.2 Circuit System Simulation
### 2.2.1 Capacitor Discharge Simulation
Capacitor discharge simulation involves solving for current and voltage in a capacitor discharge circuit. ode45 can simulate its behavior by solving the differential equations of the circuit. The differential equations are:
```python
def capacitor_discharge(t, y):
"""Capacitor discharge circuit differential equations.
Args:
t (float): Time.
y (list): State variables [voltage, current].
Returns:
list: Derivatives of state variables [current derivative, voltage derivative].
"""
C = 1e-6 # Capacitance
R = 1e3 # Resistance
dydt = [-(y[1]/C), -y[0]/R]
return dydt
```
Where `t` is time, `y` is the state variable, including voltage and current. `C` is the capacitance, and `R` is the resistance.
```python
import numpy as np
import matplotlib.pyplot as plt
# Initial conditions
y0 = [10, 0] # Initial voltage and current
# Solve differential equations
t_span = np.linspace(0, 0.01, 1000) # Time range
sol = ode45(capacitor_discharge, t_span, y0)
# Plot results
plt.plot(sol.t, sol.y[0, :])
plt.xlabel('Time (s)')
plt.ylabel('Voltage (V)')
plt.show()
```
### 2.2.2 Inductor Charge and Discharge Simulation
Inductor charge and discharge simulation involves solving for current and voltage in an inductor charge and discharge circuit. ode45 can simulate its behavior by solving the differential equations of the circuit. The differential equations are:
```python
def inductor_charge_discharge(t, y):
"""Inductor charge and discharge circuit differential equations.
Args:
t (float): Time.
y (list): State variables [current, voltage].
Returns:
list: Derivatives of state variables [voltage derivative, current derivative].
"""
L = 1e-3 # Inductance
R = 1e3 # Resistance
E = 10 # Power supply voltage
dydt = [-(R/L)*y[1] + (E/L), -(y[0]/L)]
return dydt
```
Where `t` is time, `y` is the state variable, including current and voltage. `L` is the inductance, `R` is the resistance, and `E` is the power supply voltage.
```python
import numpy as np
import matplotlib.pyplot as plt
# Initial conditions
y0 = [0, 0] # Initial current and voltage
# Solve differential equations
t_span = np.linspace(0, 0.01, 1000) # Time range
sol = ode45(inductor_ch
```
0
0
相关推荐








