Solving Differential Equations with ode45: Clever Tricks in Computer Graphics, Solving Five Puzzles
发布时间: 2024-09-15 06:10:33 阅读量: 28 订阅数: 28 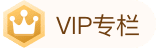
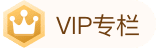
# 1. An Introduction to Differential Equations
A differential equation is a mathematical equation that describes the relationship between an unknown function and its derivatives with respect to one or more independent variables. Differential equations have extensive applications in science and engineering, including physics, chemistry, biology, and computer graphics.
Differential equations can be classified into ordinary differential equations and partial differential equations. Ordinary differential equations involve a function of a single independent variable, while partial differential equations involve functions of several independent variables. The process of solving differential equations usually involves using analytical methods or numerical methods.
# 2. Theoretical Basis of the ode45 Differential Equation Solver
### 2.1 Types and Solving Methods of Differential Equations
Differential equations are a category of equations that describe the rate of change of functions, with extensive applications in science, engineering, and finance. There are many types of differential equations, the most common of which include:
- **Ordinary Differential Equations (ODE)**: Differential equations that involve only one independent variable.
- **Partial Differential Equations (PDE)**: Differential equations that involve multiple independent variables.
- **Differential-Algebraic Equations (DAE)**: Systems of equations that include both differential equations and algebraic equations.
There are many methods for solving differential equations, including:
- **Analytical Solution Methods**: Directly finding the analytical expression of the differential equation.
- **Numerical Solution Methods**: Using computers to perform numerical calculations, obtaining an approximate solution to the differential equation.
The ode45 solver is a numerical method specifically designed for solving ordinary differential equations.
### 2.2 Principles and Algorithms of the ode45 Solver
The ode45 solver is based on the Runge-Kutta method, a single-step solver. It divides the solution space of the differential equation into a series of time steps and then uses the Runge-Kutta formulas to calculate the approximate values of the solution within each time step.
The ode45 solver employs the 4th-order Runge-Kutta formula, also known as the RK4 method. The RK4 method's calculation formula is as follows:
```
k1 = h * f(t_n, y_n)
k2 = h * f(t_n + h/2, y_n + k1/2)
k3 = h * f(t_n + h/2, y_n + k2/2)
k4 = h * f(t_n + h, y_n + k3)
y_{n+1} = y_n + (k1 + 2*k2 + 2*k3 + k4) / 6
```
Where:
- `t_n` and `y_n` are the current time and the approximate value of the solution, respectively.
- `h` is the time step.
- `f(t, y)` is the right-hand side function of the differential equation.
The ode45 solver controls the solution accuracy by adjusting the time step `h`. If the solution accuracy does not meet the requirements, the ode45 solver will automatically adjust the size of `h`.
**Code Block**:
```python
import numpy as np
import matplotlib.pyplot as plt
# Define the right-hand side function of the differential equation
def f(t, y):
return -y
# Define initial conditions
y0 = 1
# Define the time range
t_span = np.linspace(0, 10, 100)
# Solve the differential equation using the ode45 solver
solution = ode45(f, t_span, y0)
# Plot the solution curve
plt.plot(solution.t, solution.y[0])
plt.show()
```
**Code Logic Analysis**:
1. Import necessary libraries.
2. Define the right-hand side function `f(t, y)` of the differential equation.
3. Define initial conditions `y0`.
4. Define the time range `t_span`.
5. Use the `ode45` solver to solve the differential equation and store the solution in `solution`.
6. Plot the solution curve.
**Parameter Description**:
- `f`: The right-hand side function of the differential equation.
- `t_span`: The time range.
- `y0`: Initial conditions.
- `solution`: Variable for storing the solution.
# 3. Practical Applications of the ode45 Differential Equation Solver
### 3.1 Application of the ode45 Solver in Computer Graphics
The ode45 solver has extensive applications in computer graphics and can be used to solve various problems related to motion, deformation, and fluid simulation.
#### 3.1.1 Physical Simulation
The ode45 solver can be used to simulate real-world physical phenomena, such as rigid body motion, fluid flow, and
0
0
相关推荐
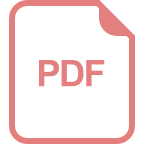
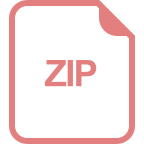
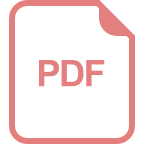
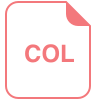
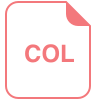
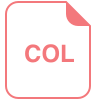
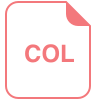
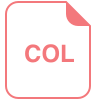
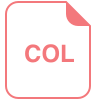