Solving Differential Equations with ODE45: From Beginner to Expert, Mastering 10 Key Steps
发布时间: 2024-09-15 05:51:12 阅读量: 47 订阅数: 38 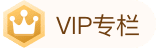
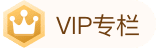
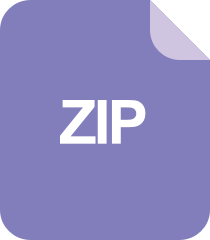
Solving Multiterm Fractional Differential equations (FDE):用一阶隐乘积梯形法则求解多项式分数微分方程-matlab开发
# 1. Introduction to Solving Differential Equations with ode45
ode45 is a solver in MATLAB used for solving systems of ordinary differential equations. It is based on the Runge-Kutta method, a numerical technique that approximates solutions to differential equations through iterative calculations. Known for its high precision, stability, and efficiency, ode45 is suitable for solving a wide range of differential equation systems.
The process of solving differential equations with ode45 involves defining the system of differential equations, setting the solving parameters and initial conditions, calling the ode45 solver, and analyzing and interpreting the results. By adjusting different solving parameters, such as step size and tolerance, the accuracy and efficiency of the solution can be controlled.
# 2. Theoretical Basis for Solving Differential Equations with ode45
### 2.1 Basic Concepts of Differential Equations
A differential equation is a mathematical expression that describes the relationship between an unknown function and its derivatives. It is widely used in science, engineering, and finance to model and analyze various dynamic systems.
The general form of a differential equation is:
```
y' = f(x, y)
```
Where:
- `y` is the unknown function
- `x` is the independent variable
- `y'` is the derivative of `y` with respect to `x`
- `f` is a function of `x` and `y`
The order of a differential equation is determined by the highest order of the derivatives of the unknown function. A first-order differential equation contains only first derivatives, a second-order differential equation includes second derivatives, and so on.
### 2.2 Principles and Methods of Numerical Solutions
Solving differential equations often requires numerical methods because analytical solutions are frequently difficult to obtain. Numerical methods discretize the differenti***
***mon numerical methods include:
- **Euler's Method:** A simple explicit method that computes the value at the next point using the derivative's approximation at the current point.
- **Improved Euler's Method:** An enhanced version of Euler's method that uses the derivative approximations at the current and previous points to calculate the next point's value.
- **Runge-Kutta Method:** A class of explicit methods that employ multiple derivative approximations to calculate the next point's value.
- **Backward Euler's Method:** An implicit method that uses the derivative approximation at the next point to calculate the current point's value.
The ode45 function in MATLAB, used for solving ordinary differential equations, employs a method called the Runge-Kutta-Fehlberg method, which features adaptive step size control. It dynamically adjusts the step size based on the solution error, thereby improving the accuracy and efficiency of the solution.
### Code Example
The following code demonstrates how to use ode45 to solve a first-order differential equation:
```
% Define the differential equation
dydt = @(t, y) t + y;
% Set initial conditions
y0 = 1;
% Set the time span for the solution
tspan = [0, 1];
% Use ode45 to solve the differential equation
[t, y] = ode45(dydt, tspan, y0);
% Plot the solution
plot(t, y);
xlabel('t');
ylabel('y');
title('Numerical Solution of a First-Order Differential Equation');
```
**Code Logic Analysis:**
- The `dydt` function defines the differential equation `y' = t + y`.
- The `y0` variable specifies the initial condition `y(0) = 1`.
- The `tspan` variable specifies the time span for the solution `[0, 1]`.
- The `ode45` function solves the differential equation using the Runge-Kutta-Fehlberg method and returns the solution for `t` and `y`.
- Finally, the plot shows how the solution `y` varies with time `t`.
# 3. Practical Steps for Solving Differential Equations with ode45
### 3.1 Determine the Type and Characteristics of the Differential Equation
Before using ode45 to solve differential equations, it is necessary to determine the type and characteristics of the equation. This will help in selecting an appropriate solution method and setting proper solving parameters.
**Types of Differential Equations**
Differential equations can be categorized into several types:
- **Ordinary Differential Equations (ODE)**: Equations that involve one independent variable and one or more dependent variables and their derivatives.
- **Partial Differential Equations (PDE)**: Equations that include multiple independent variables and dependent variables and their partial derivatives.
- **Integral Differential Equations (IDE)**: Equations that involve both integration and differentiation operators.
**Characteristics of Differential Equations**
The characteristics of a differential equation include:
- **Order**: The order of the highest derivative.
- **Linearity**: Whether the equation can be expressed as a linear combination of the dependent variable and its derivatives.
- **Homogeneity**: Whether the equation does not contain constant terms of the dependent variable or its derivatives.
- **Self-adjointness**: Whether the equation satisfies certain symmetry conditions.
### 3.2 Set Solving Parameters and Initial Conditions
After determining the type and characteristics of the differential equation, you need to set the solving parameters and initial conditions.
**Solving Parameters**
Solving parameters for ode45 include:
- **RelTol**: Relative error tolerance.
- **AbsTol**: Absolute error tolerance.
- **MaxStep**: Maximum step size.
- **InitialStep**: Initial step size.
- **Events**: Event functions for handling discrete events within the equation.
**Initial Conditions**
Initial conditions specify the values of the dependent variables at the beginning of the solution. They must be consistent with the type and characteristics of the differential equation.
### 3.3 Use ode45 to Solve Differential Equations
Once the solving parameters and initial conditions are set, you can use ode45 to solve the differential equation. The syntax for the ode45 function is as follows:
```matlab
[t, y] = ode45(@ode_func, tspan, y0, options)
```
Where:
- `ode_func`: The function representing the right-hand side of the differential equation.
- `tspan`: The time span for the solution.
- `y0`: The initial condition.
- `options`: Solving parameters.
The ode45 function returns the solution results:
- `t`: The time points of the solution.
- `y`: The values of the dependent variable during the solution.
**Code Block: Solving a First-Order ODE with ode45**
```matlab
% Define the right-hand side function of the differential equation
ode_func = @(t, y) -y + 1;
% Set solving parameters and initial conditions
tspan = [0, 10];
y0 = 1;
options = odeset('RelTol', 1e-6, 'AbsTol', 1e-9);
% Solve the differential equation
[t, y] = ode45(ode_func, tspan, y0, options);
% Plot the solution results
plot(t, y);
xlabel('Time');
ylabel('y');
title('Solution of the ODE');
```
**Code Logic Analysis**
This code uses ode45 to solve the first-order ordinary differential equation `y' = -y + 1`.
- The `ode_func` function defines the right-hand side of the differential equation, `-y + 1`.
- `tspan` sets the time span for the solution to `[0, 10]`.
- `y0` sets the initial condition to `1`.
- `options` sets the solving parameters, including a relative error tolerance of `1e-6` and an absolute error tolerance of `1e-9`.
- The `ode45` function solves the differential equation and returns the time points `t` and the values of the dependent variable `y`.
- Finally, the `plot` function is used to visualize the solution results.
### 3.4 Analyze and Interpret the Solution Results
After solving the differential equation, it is necessary to analyze and interpret the results. This includes:
- **Checking Errors**: Compare the solution results with known solutions or other numerical methods to assess the error.
- **Analyzing the Solution's Properties**: Determine the stability, periodicity, or other characteristics of the solution.
- **Interpreting the Physical Meaning**: If the differential equation describes a physical system, explain the physical significance of the solution.
# ***mon Issues and Solutions When Solving Differential Equations with ode45
### 4.1 Sources of Error in Numerical Methods
When using ode45 to solve differential equations, errors are inevitable due to the nature of numerical methods. These errors can come from several aspects:
- **Truncation Error**: This arises from using a finite step size for numerical integration of the differential equation. The smaller the step size, the smaller the truncation error.
- **Rounding Error**: This is the error produced by a computer during floating-point operations.
- **Rounding Error**: This is the error produced by a computer during floating-point operations.
- **Modeling Error**: This is due to the approximation or simplification of the differential equation model itself.
### 4.2 Convergence Problems and Optimization Strategies
During the use of ode45 to solve differential equations, convergence problems may sometimes occur. This may be caused by the following reasons:
- **Inappropriate Step Size Selection**: A step size that is too large can lead to significant truncation errors, while a step size that is too small increases computational time.
- **Inappropriate Initial Conditions**: If the initial conditions are too far from the true solution, it can cause the solution process to diverge.
- **High Stiffness of Differential Equations**: Stiff differential equations are highly sensitive to step size, and small mistakes can lead to convergence failure.
To address convergence problems, the following optimization strategies can be employed:
- **Adaptive Step Size**: ode45 can automatically adjust the step size to control truncation errors and thus improve convergence.
- **Change Initial Conditions**: If the initial conditions are too far from the true solution, try adjusting them to be closer to the actual solution.
- **Use Less Stiff Solving Methods**: For highly stiff differential equations, use solving methods specifically designed for stiff equations, such as BDF methods or Rosenbrock methods.
### 4.3 Handling Special Cases and Nonlinear Equations
When using ode45 to solve differential equations, one may encounter special cases and nonlinear equations that require special treatment.
**Special Cases:**
- **Singular Points**: Singular points are points in the differential equation where the derivative does not exist or is infinite. Around singular points, ode45 may not converge.
- **Boundary Conditions**: Boundary conditions are constraints on the differential equation solution at specific boundaries. ode45 cannot directly handle boundary conditions and requires users to code solutions themselves.
**Nonlinear Equations:**
- **Nonlinear Differential Equations**: Nonlinear differential equations are systems of nonlinear equations that are more complex to solve than linear differential equations. ode45 can solve nonlinear differential equations, but the process may be more time-consuming.
- **Algebraic Equations**: Algebraic equations are a special type of nonlinear equation system where the number of unknowns equals the number of equations. ode45 can convert algebraic equation systems into differential equation systems and then solve them.
When handling special cases and nonlinear equations, different strategies need to be adopted based on the specific situation. For instance, for singular points, one might try using adaptive step size or changing initial conditions to bypass the singular points; for boundary conditions, the user must write their own code to handle them; for nonlinear differential equations, iterative methods or other nonlinear equation solving methods can be used to find solutions.
# 5. Application Examples of Solving Differential Equations with ode45
The use of ode45 to solve differential equations has broad applications in science, engineering, and finance. Here are a few common application examples:
### 5.1 Solving Motion Equations in Physics
In physics, Newton's second law describes the motion of an object under the action of forces. This law can be represented by the following second-order differential equation:
```
m * d^2x/dt^2 = F(t)
```
Where m is the mass of the object, x is the displacement of the object, t is time, and F(t) is the force acting on the object.
Using ode45, this differential equation can be solved to obtain the curves of the object's displacement and velocity over time. This has significant applications in motion analysis, ballistics, and celestial mechanics.
### 5.2 Chemical Reaction Kinetic Models
In chemical reactions, the concentration of reactants changes over time according to specific differential equations. These differential equations describe the generation and consumption rates of reactants.
For example, a simple second-order reaction kinetic model can be represented by the following differential equation system:
```
dA/dt = -k * A^2
dB/dt = 2 * k * A^2
```
Where A and B are the concentrations of the reactants, and k is the rate constant of the reaction.
Using ode45, these differential equations can be solved to obtain the curves of the reactant concentrations over time. This has important applications in chemical reaction engineering, pharmacokinetics, and environmental modeling.
### 5.3 Modeling and Simulation of Biological Systems
In biological systems, many processes can be described by differential equations, such as population growth, disease spread, and ecosystem dynamics.
For instance, a population growth model can be represented by the following differential equation:
```
dN/dt = r * N * (1 - N/K)
```
Where N is the population size, r is the growth rate, and K is the environmental carrying capacity.
Using ode45, this differential equation can be solved to obtain the curve of population size over time. This has significant applications in ecology, epidemiology, and biotechnology.
0
0
相关推荐







