Solving Differential Equations with ODE45: A Secret Weapon in Finance and Economics, Mastering 5 Key Applications
发布时间: 2024-09-15 05:58:16 阅读量: 33 订阅数: 28 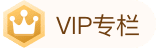
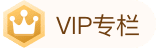
# Chapter 1: The Principle and Method of Solving Differential Equations with ode45
The ode45 solver is a numerical method for solving ordinary differential equations (ODEs). It is based on the Runge-Kutta method, a single-step method that uses the solution from the previous step to compute the current step. The ode45 solver employs the fourth-order Runge-Kutta method, which is an explicit method, meaning it does not require solving linear equation systems.
The ode45 solver uses adaptive step sizes, adjusting the step based on the local error of the solution. This feature makes the ode45 solver highly effective for solving stiff equations, which are equations with a wide range of time scales. The ode45 solver also provides estimates for local and global errors, aiding in assessing the accuracy of the solution.
# Chapter 2: Applications of Solving Differential Equations with ode45 in Finance
### 2.1 Stock Price Prediction
#### 2.1.1 Differential Equation Model of Stock Price Movement
The motion of stock prices is typically described using stochastic differential equations. One of the most common models is the Geometric Brownian Motion model, with its differential equation form being:
```
dS/dt = μS + σS * dW
```
Where:
- S is the stock price
- μ is the drift rate
- σ is the volatility
- dW is the Wiener process
#### 2.1.2 Using ode45 to Solve Stock Price Prediction Model
The ode45 solver can be used to solve the stock price prediction model. Below is the code for using ode45 in Python to solve the Geometric Brownian Motion model:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
# Define model parameters
μ = 0.05
σ = 0.2
S0 = 100
# Define time range
t = np.linspace(0, 1, 100)
# Define differential equation
def f(S, t):
return μ * S + σ * S * np.random.randn()
# Solve differential equation
S = odeint(f, S0, t)
# Plot result
plt.plot(t, S)
plt.xlabel("Time")
plt.ylabel("Stock Price")
plt.show()
```
Line-by-line code logic interpretation:
- Import necessary libraries.
- Define model parameters: drift rate, volatility, and initial stock price.
- Define time range.
- Define differential equation, where np.random.randn() generates normally distributed random numbers.
- Solve differential equation using odeint function.
- Plot results.
### 2.2 Option Pricing
#### 2.2.1 Differential Equation Form of Option Pricing Model
Option pricing models are typically described using partial differential equations. One of the most common models is the Black-Scholes model, with its differential equation form being:
```
∂V/∂t + ½σ²S²∂²V/∂S² + rSV∂V/∂S - rV = 0
```
Where:
- V is the option value
- S is the stock price
- t is time
- σ is the volatility
- r is the risk-free interest rate
#### 2.2.2 Using ode45 to Solve Option Pricing Model
The ode45 solver can also be used to solve option pricing models. Below is the code for using ode45 in Python to solve the Black-Scholes model:
```python
import numpy as np
import matplotlib.pyplot as plt
from scipy.integrate import odeint
# Define model parameters
σ = 0.2
r = 0.05
S0 = 100
T = 1
# Define time range
t = np.linspace(0, T, 100)
# Define differential equation
def f(V, t):
return -0.5 * σ**2 * S0**2 * V'' + r * S0 * V' - r * V
# Solve differential equation
V = odeint(f, V0, t)
# Plot result
plt.plot(t, V)
plt.xlabel("Time")
plt.ylabel("Option Value")
plt.show()
```
Line-by-line code logic interpretation:
- Import necessary libraries.
- Define model parameters: volatility, risk-free interest rate, initial stock price, and time to maturity.
- Define time range.
- Define differential equation, where V'' represents the second derivative and V' represents the first derivative.
- Solve differential equation using odeint function.
- Plot results.
# Chapter 3: Economic Growth Models
#### 3.1.1 Differential Equation Form of Economic Growth Model
Economic growth models are mathematical models that describe how an economic system changes over time. Differential equations are a mathematical tool that can be used to represent the rate of change of economic variables over time. Therefore, differential eq
0
0
相关推荐
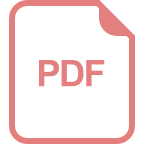
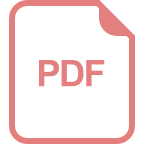
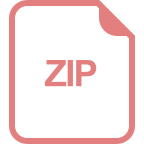
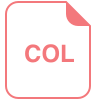
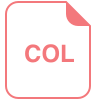
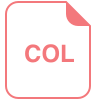
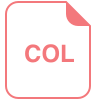
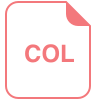
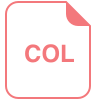
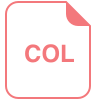