[Practical Exercise] Implementation of TSP (Traveling Salesman Problem) with MATLAB Using Genetic Algorithm, Simulated Annealing Algorithm, and Lingo Dynamic Programming
发布时间: 2024-09-14 00:20:25 阅读量: 31 订阅数: 52 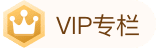
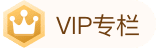
# Practical Exercise: Implementing TSP (Traveling Salesman Problem) with MATLAB
## 2.1.1 Basic Principles of Genetic Algorithm
A genetic algorithm (GA) is an optimization algorithm inspired by the process of biological evolution. It seeks the optimal solution to a problem by simulating natural selection and genetic variation. The GA process is as follows:
1. **Initialize population:** Randomly generate a set of candidate solutions, known as a population.
2. **Evaluate the population:** Calculate the fitness of each individual, i.e., how well they solve the problem.
3. **Selection:** Select better individuals in the population for reproduction based on fitness.
4. **Crossover:** Pair the selected individuals and exchange genes to produce new individuals.
5. **Mutation:** Randomly alter the genes of new individuals to introduce diversity.
6. **Repeat steps 2-5:** Until a termination condition is met (e.g., reaching the maximum number of iterations or finding a sufficiently good solution).
## 2. Implementation of TSP Algorithms in MATLAB
### 2.1 Genetic Algorithm
#### 2.1.1 Basic Principles of Genetic Algorithm
A genetic algorithm (GA) is an optimization algorithm inspired by the process of biological evolution. It iteratively generates better solutions from a set of candidate solutions (called a population) by simulating natural selection and genetic processes. The basic principles of GA are as follows:
***Initialize population:** Randomly generate a set of candidate solutions, each representing a possible TSP solution.
***Fitness evaluation:** Calculate the fitness of each solution, i.e., its ability to solve the TSP problem.
***Selection:** Select individuals in the population for reproduction based on fitness; individuals with higher fitness are more likely to be chosen.
***Crossover:** Combine the genetic information (i.e., solutions) of two selected individuals to produce new individuals.
***Mutation:** Randomly modify new individuals to introduce diversity and prevent the algorithm from converging on a local optimum.
***Iteration:** Repeat the selection, crossover, and mutation steps until a stopping condition is reached (e.g., reaching the maximum number of iterations or finding a solution that meets the requirements).
#### 2.1.2 Implementation of Genetic Algorithm in MATLAB
The `ga` function in MATLAB can be used to implement a genetic algorithm. This function accepts the following parameters:
* `FitnessFunction`: A fitness function used to calculate the fitness of each solution.
* `nvars`: The number of variables, i.e., the number of cities in the TSP.
* `options`: Algorithm options, including population size and maximum number of generations.
```matlab
% Define the fitness function
fitnessFunction = @(x) tspfun(x);
% Define algorithm options
options = gaoptimset('PopulationSize', 100, 'MaxGenerations', 100);
% Execute the genetic algorithm
[x, fval, exitflag, output] = ga(fitnessFunction, nvars, [], [], [], [], [], [], [], options);
```
### 2.2 Simulated Annealing Algorithm
#### 2.2.1 Basic Principles of Simulated Annealing Algorithm
The simulated annealing algorithm (SA) is an optimization algorithm inspired by the process of annealing in metallurgy. It gradually searches for better solutions by starting from an initial solution and gradually reducing the algorithm's temperature. The basic principles of SA are as follows:
***Initialization:** Start with a random solution and set an initial temperature.
***Perturbation:** Randomly generate a new solution and calculate its fitness.
***Acceptance criterion:** If the new solution is better than the current solution, accept it. If the new solution is worse than the current solution, accept it with a certain probability that decreases as the temperature drops.
***Temperature update:** Reduce the temperature in each iteration to decrease the probability of accepting worse solutions.
***Iteration:** Repeat the perturbation, acceptance criterion, and temperature update steps until a stopping condition is reached.
#### 2.2.2 Implementation of Simulated Annealing Algorithm in MATLAB
The `simulannealbnd` function in MATLAB can be used to implement a simulated annealing algorithm. This function accepts the following parameters:
* `fun`: The objective function used to calculate the fitness of each solution.
* `bounds`: The boundaries of variables, i.e., the range of city locations in the TSP.
* `options`: Algorithm options, including initial temperature and cooling rate.
```matlab
% Define the objective function
fun = @(x) tspfun(x);
% Define variable boundaries
bounds = [0, 100; 0, 100];
% Define algorithm options
options = simulannealbnd(fun, bounds, options);
% Execute the simulated annealing al
```
0
0
相关推荐







