MATLAB Normal Distribution Fitting: Exploring the Best Fit for Data Distribution
发布时间: 2024-09-14 15:17:02 阅读量: 58 订阅数: 37 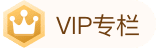
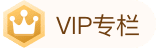
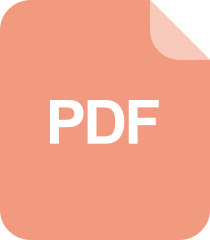
Matlab Curve Fitting Toolbox工具箱使用说明手册

# 1. Basics of Normal Distribution**
The normal distribution, also known as the Gaussian distribution, is a continuous probability distribution characterized by a bell-shaped probability density function. It is widely used in nature and statistics to describe various phenomena such as height, weight, and measurement errors.
The mathematical formula for the normal distribution is:
```
f(x) = (1 / (σ√(2π))) * e^(-(x - μ)² / (2σ²))
```
Where:
* μ is the mean of the normal distribution, indicating the center of the distribution.
* σ is the standard deviation of the normal distribution, indicating the dispersion of the distribution.
# 2. Fitting Normal Distribution in MATLAB
### 2.1 Normal Distribution Fitting Functions
MATLAB provides two functions for normal distribution fitting: `normfit` and `fitdist`.
#### 2.1.1 normfit Function
The `normfit` function is used for fitting a normal distribution and returns the mean and standard deviation. Its syntax is as follows:
```
[mu, sigma] = normfit(data)
```
Where:
- `data`: Input data vector or matrix.
- `mu`: The mean of the fitted normal distribution.
- `sigma`: The standard deviation of the fitted normal distribution.
#### 2.1.2 fitdist Function
The `fitdist` function is used for fitting various distributions, including the normal distribution. Its syntax is as follows:
```
pd = fitdist(data, 'Normal')
```
Where:
- `data`: Input data vector or matrix.
- `pd`: The fitted probability distribution object, which contains parameters such as mean and standard deviation.
### 2.2 Interpretation of Fitting Parameters
#### 2.2.1 Mean and Standard Deviation
The mean (`mu`) represents the central location of the normal distribution, while the standard deviation (`sigma`) represents the dispersion of the distribution.
#### 2.2.2 Confidence Intervals
Both `normfit` and `fitdist` functions also return confidence intervals, indicating the uncertainty range of the estimated mean and standard deviation at a given confidence level.
### 2.3 Fitting Quality Assessment
#### 2.3.1 Residual Analysis
Residual analysis is used to evaluate the difference between the fitted normal distribution and the actual data. The residual is the difference between each data point and the expected value of the fitted distribution.
#### 2.3.2 Chi-Square Test
The Chi-Square test is a statistical test used to assess the goodness of fit between the fitted normal distribution and the actual data. The Chi-Square statistic measures the difference between observed and expected values.
### Code Example
```
% Generate normal distribution data
data = normrnd(0, 1, 100);
% Fit normal distribution using normfit function
[mu, sigma] = normfit(data);
% Fit normal distribution using fitdist function
pd = fitdist(data, 'Normal');
% Print fitting parameters
fprintf('Mean: %.2f\n', mu);
fprintf('Standard Deviation: %.2f\n', sigma);
```
**Code Logic Analysis:**
1. The `normrnd` function generates normal distribution data.
2. The `normfit` function fits the normal distribution and returns the mean and standard deviation.
3. The `fitdist` function fits the normal distribution and returns a probability distribution object.
4. The `fprintf` function prints the fitting parameters.
### Table: Comparison of Fitting Functions
| Function | Fitting Parameters | Confidence Intervals | Residual Analysis | Chi-Square Test |
|---|---|---|---|---|
| `normfit` | Mean, Standard Deviation | Yes | Yes | No |
| `fitdist` | Mean, Standard Deviation, Skewness, Kurtosis | Yes | Yes | Yes |
### Mermaid Flowchart: Normal Distribution Fitting Process
```mermaid
sequenceDiagram
participant User
participant MATLAB
User->MATLAB: Generate data
MATLAB->User: Data generated
User->MATLAB: Fit distribution
MATLAB->User: Distribution fitted
User->MATLAB: Evaluate fit
MATLAB
```
0
0
相关推荐








