MATLAB Normal Distribution in Machine Learning: Unveiling the Role of Normal Distribution in Machine Learning
发布时间: 2024-09-14 15:34:26 阅读量: 22 订阅数: 29 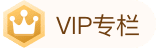
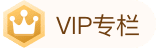
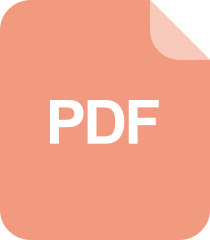
WebFace260M A Benchmark Unveiling the Power of Million-Scale.pdf
# MATLAB Normal Distribution in Machine Learning: Unveiling the Role of the Normal Distribution in Machine Learning
## 1. Theoretical Foundations of the Normal Distribution**
The normal distribution, also known as the Gaussian distribution, is a continuous probability distribution that takes the shape of a bell curve. It is extensively used in statistics and machine learning because it can describe numerous natural phenomena and datasets.
The mathematical expression of the normal distribution is:
```
f(x) = (1 / (σ√(2π))) * e^(-(x - μ)² / (2σ²))
```
Where:
* μ is the mean of the normal distribution, representing the central position of the data.
* σ is the standard deviation of the normal distribution, indicating the dispersion of the data.
## 2. Applications of the Normal Distribution in Machine Learning
### 2.1 Application of the Normal Distribution in Classification
#### 2.1.1 Naive Bayes Classifier
The naive Bayes classifier is a probabilistic classifier based on Bayes' theorem. It assumes that the features are mutually independent; in other words, the value of one feature does not affect the value of other features.
**Code Block:**
```python
import numpy as np
import pandas as pd
from sklearn.naive_bayes import GaussianNB
# Import data
data = pd.read_csv('data.csv')
# Separate features and labels
X = data.drop('label', axis=1)
y = data['label']
# Train the naive Bayes classifier
model = GaussianNB()
model.fit(X, y)
# Predict new data
new_data = pd.DataFrame({'feature1': [1, 2, 3], 'feature2': [4, 5, 6]})
predictions = model.predict(new_data)
```
**Logical Analysis:**
* `GaussianNB()`: Create a naive Bayes classifier.
* `fit(X, y)`: Train the classifier, where X is the features and y is the labels.
* `predict(new_data)`: Use the trained classifier to predict new data.
#### 2.1.2 Support Vector Machines
Support vector machines are a classification algorithm that map data points to a high-dimensional space and find a hyperplane to separate the data points into different categories.
**Code Block:**
```python
import numpy as np
import pandas as pd
from sklearn.svm import SVC
# Import data
data = pd.read_csv('data.csv')
# Separate features and labels
X = data.drop('label', axis=1)
y = data['label']
# Train the support vector machine classifier
model = SVC()
model.fit(X, y)
# Predict new data
new_data = pd.DataFrame({'feature1': [1, 2, 3], 'feature2': [4, 5, 6]})
predictions = model.predict(new_data)
```
**Logical Analysis:**
* `SVC()`: Create a support vector machine classifier.
* `fit(X, y)`: Train the classifier, where X is the features and y is the labels.
* `predict(new_data)`: Use the trained classifier to predict new data.
### 2.2 Application of the Normal Distribution in Regression
#### 2.2.1 Linear Regression
Linear regression is a regression algorithm used for predicting continuous target variables. It assumes a linear relationship between the target variable and the feature variables.
**Code Block:**
```python
import numpy as np
import pandas as pd
from sklearn.linear_model import LinearRegression
# Import data
data = pd.read_csv('data.csv')
# Separate features and labels
X = data.drop('label', axis=1)
y = data['label']
# Train the linear regression model
model = LinearRegression()
model.fit(X, y)
# Predict new data
new_data = pd.DataFrame({'feature1': [1, 2, 3], 'feature2': [4, 5, 6]})
predictions = model.predict(new_data)
```
**Logical Analysis:**
* `LinearRegression()`: Create a linear regression model.
* `fit(X, y)`: Train the model, where X is the features and y is the labels.
* `predict(new_data)`: Use the trained model to predict new data.
#### 2.2.2 Logistic Regression
Logistic regression is a regression algorithm used for binary classification problems. It maps input data to a probability value, representing the probability of belonging to a certain class.
**Code Block:**
```python
import numpy as np
import pandas as pd
from sklearn.linear_model import LogisticRegression
# Import data
data = pd.read_csv('data.csv')
# Separate features and labels
X = data.drop('label', axis=1)
y = data['label']
# Train the logistic regression model
model = LogisticRegression()
model.fit(X, y)
# Predict new data
new_data = pd.DataFrame({'feature1': [1, 2, 3], 'feature2': [4, 5, 6]})
predictions = model.predict(new_data)
```
**Logical Analysis:**
* `LogisticRegression()`: Create a logistic regression model.
* `fit(X, y)`: Train the model, where X is the features and y is the labels.
* `predict(new_data)`: Use the trained model to predict new data.
# 3.1 Applications of the Normal Distribution in Data Analysis
The normal distribution plays a crucial role in data analysis, providing a solid foundation for data exploration
0
0