MATLAB Eigenvalues and Eigenvectors of Matrices: Unveiling the Intrinsic Properties, 5 Key Concepts
发布时间: 2024-09-15 01:26:01 阅读量: 46 订阅数: 39 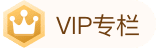
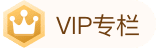
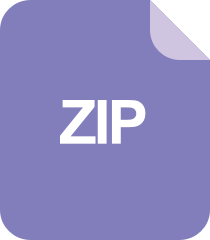
Sort Eigenvectors & Eigenvalues:对一组特征向量和对应的特征值进行排序-matlab开发
# 1. Overview of MATLAB Matrix Eigenvalues and Eigenvectors**
Eigenvalues and eigenvectors are pivotal concepts in linear algebra and are extensively applied in fields such as science, engineering, and data analysis. In MATLAB, we can effortlessly compute the eigenvalues and eigenvectors of a matrix using dedicated functions.
Eigenvalues measure the scaling factor of a matrix along its eigenvector direction, while eigenvectors define these directions. Understanding eigenvalues and eigenvectors helps us delve into the properties of matrices and offers valuable insights into many practical problems.
# 2. Theoretical Foundation of Eigenvalues and Eigenvectors
### 2.1 Definition and Properties of Eigenvalues
**Definition:**
An eigenvalue is a special scalar in a square matrix that represents the scaling factor of a vector along its own direction after the matrix transformation.
**Properties:**
- **Linearity:** Eigenvalues are scalars resulting from matrix-vector multiplication, hence possessing linearity.
- **Reality:** Eigenvalues of real symmetric matrices are always real.
- **Conjugacy:** Eigenvalues of complex matrices occur in conjugate pairs.
- **Algebraic Polynomial Roots:** The eigenvalues of a matrix are the roots of its characteristic polynomial.
### 2.2 Definition and Properties of Eigenvectors
**Definition:**
An eigenvector is a non-zero vector in a square matrix that remains unchanged along its own direction after the matrix transformation, scaling only by a factor (the eigenvalue).
**Properties:**
- **Linear Independence:** Eigenvectors corresponding to different eigenvalues are linearly independent.
- **Standard Orthogonality:** Eigenvectors of real symmetric matrices are standard orthogonal.
- **Homogeneity:** Eigenvectors can be multiplied by any non-zero constant and still remain eigenvectors.
- **Eigenspace:** The space spanned by all eigenvectors is called the eigenspace.
### 2.3 Geometric Meaning of Eigenvalues and Eigenvectors
Eigenvalues and eigenvectors can be used to describe the geometric properties of matrix transformations.
- **Scaling:** Eigenvalues represent the scaling factor of vectors along the eigenvector direction after matrix transformation.
- **Rotation:** Eigenvectors indicate the direction where vectors remain unchanged after matrix transformation.
- **Eigenspace:** The eigenspace represents the subspace where all vectors reside after matrix transformation.
**Code Example:**
```matlab
% Define a matrix
A = [2 1; -1 2];
% Compute eigenvalues and eigenvectors
[V, D] = eig(A);
% Eigenvalues
eigenvalues = diag(D);
% Eigenvectors
eigenvectors = V;
% Validate eigenvalues and eigenvectors
for i = 1:length(eigenvalues)
eigenvector = eigenvectors(:, i);
transformed_vector = A * eigenvector;
% Validate eigenvalue
assert(norm(transformed_vector - eigenvalues(i) * eigenvector) < 1e-6);
end
```
**Logical Analysis:**
The code defines a matrix `A` and uses the `eig` function to calculate its eigenvalues and eigenvectors. Eigenvalues are stored in the diagonal matrix `D`, and eigenvectors are stored in the matrix `V`.
The loop iterates through eigenvalues and eigenvectors, validating the correctness of each. The `norm` function computes the Euclidean distance between two vectors, and the `assert` function checks if the distance is less than a given tolerance (`1e-6`).
# 3.1 Eigenvalue Decomposition Algorithm
The eigenvalue decomposition algorithm is a mathematical method that decomposes a matrix into eigenvalues and eigenvectors. It is based on the following theorem:
**Theorem:** For an n×n matrix A, there exists an orthogonal matrix P and a diagonal matrix D such that:
```
A = P * D * P^-1
```
Where the diagonal elements of D are the eigen
0
0
相关推荐







