MATLAB Matrix Singular Value Decomposition (SVD): Exploring Low-Rank Approximations with 4 Application Scenarios
发布时间: 2024-09-15 01:27:20 阅读量: 10 订阅数: 18 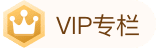
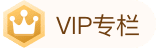
# 1. Singular Value Decomposition (SVD) Overview
Singular Value Decomposition (SVD) is a powerful linear algebra technique used to factorize a matrix into the product of three matrices: a left singular vector matrix, a singular value matrix, and a right singular vector matrix. SVD is widely applied across various fields, including data science, image processing, and natural language processing.
The essence of SVD is to decompose a matrix into a set of singular values and singular vectors. Singular values represent the variance in the data within the matrix, while singular vectors indicate the distribution of the data across different dimensions. Through matrix decomposition, SVD can uncover the latent structure and patterns within the data.
# 2. Theoretical Foundations of SVD
**2.1 Singular Values and Singular Vectors**
Singular Value Decomposition (SVD) is a mathematical technique that decomposes a matrix into singular values and corresponding singular vectors. Singular values are non-negative real numbers that are the square roots of the eigenvalues of the matrix, while singular vectors are the eigenvectors corresponding to these singular values.
For an m×n matrix A, its SVD can be represented as:
```
A = UΣV^T
```
Where:
* U is an m×m unitary matrix whose column vectors are the left singular vectors of A.
* Σ is an m×n diagonal matrix whose diagonal elements are the singular values of A, arranged in descending order.
* V is an n×n unitary matrix whose column vectors are the right singular vectors of A.
**2.2 Geometric Interpretation of SVD**
SVD can be understood geometrically as decomposing matrix A into a series of orthogonal transformations.
***Left Singular Vectors U:** Project the row space of A onto an m-dimensional unit hyperplane.
***Singular Values Σ:** Describe the lengths of the projected row vectors, with larger singular values indicating longer projected row vectors.
***Right Singular Vectors V:** Project the column space of A onto an n-dimensional unit hyperplane.
**2.3 Computational Methods for SVD**
Common methods for computing SVD include:
***Jacobi Method:** Diagonalize the matrix through a series of orthogonal transformations.
***QR Algorithm:** Decompose the matrix into a product of a series of unitary matrices using QR decomposition.
***Singular Value Decomposition Theorem:** Factorize the matrix into the product of singular values and corresponding singular vectors using the SVD theorem.
**Code Block:**
```python
import numpy as np
# Using NumPy to compute the SVD of matrix A
A = np.array([[1, 2], [3, 4]])
U, S, Vh = np.linalg.svd(A, full_matrices=False)
# Print singular values and singular vectors
print("Singular values:", S)
print("Left singular vectors:", U)
print("Right singular vectors:", Vh)
```
**Logical Analysis:**
This code uses NumPy's `linalg.svd()` function to compute the SVD of matrix A. The parameter `full_matrices=False` specifies that the reduced U and Vh matrices should be returned, containing only the columns corresponding to the non-zero singular values.
**Parameter Explanation:**
* `A`: The matrix to be decomposed.
* `full_matrices`: If True, return the full U and Vh matrices; if False, return the reduced U and Vh matrices.
# 3.1 Low-Rank Approximation
**3.1.1 Singular Value Truncation**
Singular value truncation is a low-rank approximation technique that approximates the original matrix by truncating smaller singular values. Specifically, for an m×n matrix A, its singular value decomposition is:
```
A = UΣV^T
```
Where U is an m×m orthogonal matrix, Σ is an m×n diagonal matrix with the singular values of A on its diagonal, and V is an n×n orthogonal matrix.
The idea behind singular value truncation is that smaller singular values correspond to singular vectors that contribute less to A. Therefore, we can truncate these smaller singular values to obtain a low-rank approximation matrix:
```
A_k = UΣ_kV^T
```
Where Σ_k is an m×n diagonal matrix that retains only the first k singular values.
**3.1.2 Compressed Sensing**
Compressed sensing is a technique for reconstructing signals from undersampled data. It leverages the sparsity of the signal, meaning that most of the signal's energy is concentrated in a few components.
In compressed sensing, the original signal is represented as a matrix A, whose SVD is:
```
A = UΣV^T
```
If A is sparse, then most of the singular values in Σ will be zero. Therefore, we can use singular value truncation to approximate A and recover the original signal from the undersampled data.
**Code Block:**
```python
import numpy as np
from scipy.linalg import svd
# Original matrix
A = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
# Singular value decomposition
U, S, Vh = svd(A, full_matrices=False)
# Singular value truncation
k = 2
A_k = np.dot(U[:, :k], np.dot(np.diag(S[:k]), Vh[:k, :]))
# Print the original matrix and the low-rank approximation matrix
print("Original matrix:")
print(A)
print("Low-rank approximati
```
0
0
相关推荐
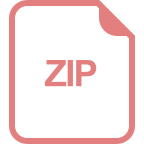
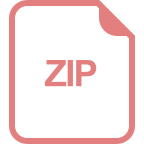
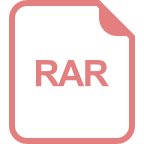
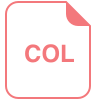
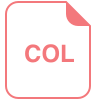
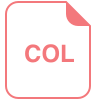
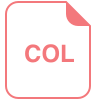

