MATLAB Sparse Matrix Representation: Efficient Processing of Large-Scale Sparse Data, 2 Key Techniques
发布时间: 2024-09-15 01:29:35 阅读量: 31 订阅数: 30 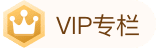
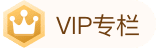
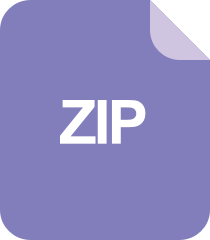
Face-classification-based-on-sparse-representation:基于稀疏表示的人脸分类
# Basic Concepts of Sparse Matrices
A sparse matrix is a special type of matrix in which most of the elements are zero. This characteristic gives it significant advantages when dealing with large datasets, as it can save substantial storage space and computation time.
The sparsity of a sparse matrix is usually measured by its sparsity, which is the ratio of the number of non-zero elements to the total number of elements in the matrix. The lower the sparsity, the sparser the matrix.
Sparse matrices are widely used in scientific computing, data analysis, and machine learning. For instance, in graph theory, sparse matrices can be used to represent the adjacency matrix of a graph; in finite element analysis, sparse matrices can be used to represent the stiffness matrix of a problem; in image processing, sparse matrices can be used to represent the Laplacian matrix of an image.
# Storage Formats of Sparse Matrices
### Compressed Sparse Row (CSR) Format
**Definition:**
The Compressed Sparse Row (CSR) format is a storage format for sparse matrices, where the column indices and values of the non-zero elements in each row are stored in two separate one-dimensional arrays.
**Structure:**
* `values` array: stores the values of the non-zero elements.
* `col_ind` array: stores the column indices of the non-zero elements.
* `row_ptr` array: stores the starting positions of the non-zero elements in each row within the `values` and `col_ind` arrays.
**Example:**
Consider the following sparse matrix:
```
A = [1 0 0; 0 2 0; 0 0 3]
```
Its CSR format storage would be:
```
values = [1, 2, 3]
col_ind = [1, 2, 3]
row_ptr = [1, 2, 3]
```
**Advantages:**
* Efficient access to non-zero elements within a row.
* Storage space is efficient, only non-zero elements are stored.
* Suitable for row-sparse matrices.
### Compressed Sparse Column (CSC) Format
**Definition:**
Compressed Sparse Column (CSC) format is similar to CSR format but is designed for column-sparse matrices. The row indices and values of the non-zero elements in each column are stored in two separate one-dimensional arrays.
**Structure:**
* `values` array: stores the values of the non-zero elements.
* `row_ind` array: stores the row indices of the non-zero elements.
* `col_ptr` array: stores the starting positions of the non-zero elements in each column within the `values` and `row_ind` arrays.
**Advantages:**
* Efficient access to non-zero elements within a column.
* Storage space is efficient, only non-zero elements are stored.
* Suitable for column-sparse matrices.
### Coordinate (COO) Format
**Definition:**
Coordinate (COO) format is a simple storage format for sparse matrices, where the row indices, column indices, and values of the non-zero elements are stored in three separate one-dimensional arrays.
**Structure:**
* `values` array: stores the values of the non-zero elements.
* `row_ind` array: stores the row indices of the non-zero elements.
* `col_ind` array: stores the column indices of the non-zero elements.
**Example:**
Considering the previous sparse matrix A, its COO format storage would be:
```
values = [1, 2, 3]
row_ind = [1, 2, 3]
col_ind = [1, 2, 3]
```
**Advantages:**
* Simple storage format, easy to understand and implement.
* Suitable for any sparse matrix.
**Disadvantages:**
* Less efficient access to non-zero elements within a row or column.
* Larger storage overhead as zero elements are also stored.
# Operations on Sparse Matrices
### Addition and Subtraction of Sparse Matrices
The addition and subtraction of sparse matrices are performed element-wise, i.e., corresponding positions are added or subtracted. For CSR format sparse matrices, the addition and subtraction operations are as follows:
```matlab
function C = sparse_add(A, B)
% Check if matrix sizes are consistent
if size(A) ~= size(B)
error('Matrix sizes do not match');
end
% Create a new sparse matrix
C = sparse(size(A));
% Iterate over row pointers
for i = 1:size(A, 1)
% Iterate over column indices
for j = A.col_ind(i):A.col_ind(i+1)-1
% Accumulate element values
C.val(j) = A.val(j) + B.val(j);
end
end
end
```
**Code Logic Analysis:**
1. Check if the sizes of the two sparse matrices are consistent.
2. Create a new sparse matrix `C`, with the same size as the input matrices.
3. Iterate over `A`'s row pointers, processing row by row.
4. For each row, iterate over `A`'s column indices, accumulating element values column by column.
5. Store the accumulated results in `C`'s `val` array.
### Multiplication of Sparse Matrices
The multiplication of sparse matrices is similar to that of dense matrices, but because most elements in sparse matrices are zero, the sparsity can be utilized to optimize the calculation. For CSR format sparse matrices, the multiplication operation is as follows:
```matlab
function C = sparse_multiply(A, B)
% Check matrix compatibility
if size(A, 2) ~= size(B, 1)
error('Matrix compatibility error');
end
% Create a new sparse matrix
C = sparse(size(A, 1), size(B, 2));
% Iterate over A's row pointers
for i = 1:size(A, 1)
% Iterate over B's colum
```
0
0
相关推荐
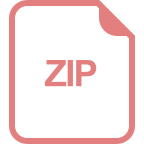
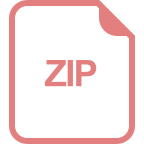
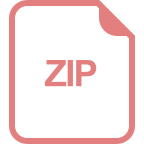
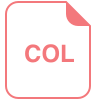
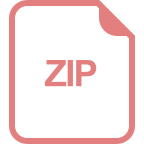
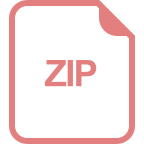
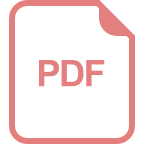