MATLAB Matrix Parallel Computing: Leveraging Multi-core Advantages to Boost Computing Speed, A Three-Step Guide
发布时间: 2024-09-15 01:30:29 阅读量: 28 订阅数: 30 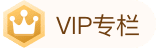
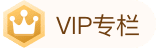
# 1. Overview of MATLAB Parallel Computing
MATLAB parallel computing is a technique that utilizes multi-core processors or computer clusters to enhance computational performance. It allows for the simultaneous execution of multiple tasks, thereby reducing computation time and increasing efficiency. MATLAB offers a variety of parallel computing tools and programming models, enabling developers to easily leverage parallel computing capabilities.
Advantages of parallel computing include:
- Shortened computation time: By executing multiple tasks simultaneously, computation time can be significantly reduced.
- Increased efficiency: Parallel computing can effectively utilize the computational resources of multi-core processors or computer clusters, thereby enhancing overall efficiency.
- Expanded computational capabilities: Parallel computing enables developers to handle large and complex problems that may be too time-consuming or infeasible for serial computing.
# 2. Fundamentals of MATLAB Parallel Computing
### 2.1 Principles of MATLAB Parallel Computing
#### 2.1.1 Concept of Multi-core Parallel Computing
Multi-core parallel computing is a technique that utilizes multi-core processors to execute multiple tasks simultaneously. In a multi-core processor, each core can independently execute instructions, thereby improving computational efficiency. MATLAB parallel computing achieves multi-core parallel computing by distributing computational tasks to different cores.
#### 2.1.2 Implementation Methods of MATLAB Parallel Computing
There are two primary ways to implement MATLAB parallel computing:
- **Shared Memory Parallel Computing:** Multiple cores share the same memory and can directly access and modify each other's data. This method is suitable for tasks with a smaller amount of data communication.
- **Distributed Memory Parallel Computing:** Each core has its own memory and needs to exchange data through message passing. This method is suitable for tasks with a larger amount of data communication.
### 2.2 MATLAB Parallel Computing Programming Models
MATLAB offers two parallel computing programming models:
#### 2.2.1 SPMD Programming Model
SPMD (Single Program Multiple Data) is a shared memory parallel computing model. In the SPMD model, all cores execute the same code but use different data. The `parfor` loop and `spmd` block in MATLAB are used to implement SPMD parallel computing.
```
% SPMD parallel computing example
parfor i = 1:1000
% Assign different data to each core
data = i * ones(1000);
% Compute data for each core
result(i) = sum(data);
end
```
#### 2.2.2 Message Passing Interface (MPI)
MPI (Message Passing Interface) is a distributed memory parallel computing model. In the MPI model, each core has its own memory and needs to exchange data through message passing. The MATLAB `MPI` toolbox is used to implement MPI parallel computing.
```
% MPI parallel computing example
% Create MPI environment
mpi_init;
% Get the number of cores
num_cores = mpi_comm_size;
% Get the core number
my_rank = mpi_comm_rank;
% Assign different data to each core
data = my_rank * ones(1000);
% Compute data for each core
result = sum(data);
% Gather results from all cores
total_result = mpi_allreduce(result);
% Close MPI environment
mpi_finalize;
```
# 3. Practice of MATLAB Parallel Computing
### 3.1 Matrix Parallel Computing
#### 3.1.1 Principles of Matrix Parallel Computing
Matrix parallel computing involves dividing a large matrix into multiple smaller blocks and then computing these blocks in parallel on different processors. This method can significantly improve the efficiency of matrix computations, especially for large matrices.
The principles of matrix parallel computing are as follows:
1. **Matrix Decomposition:** Divide the large matrix into several smaller blocks, each called a submatrix.
2. **Task Allocation:** Assign submatrices to different processors.
3. **Parallel Computing:** Each processor computes the assigned submatrix in parallel.
4. **Result Merging:** Merge the computed results from each processor into the final result.
#### 3.1.2 Steps
0
0
相关推荐
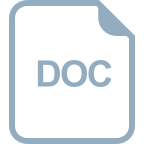
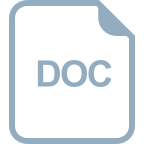
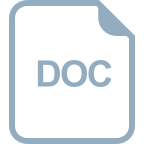
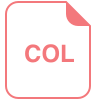
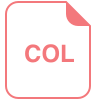
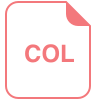
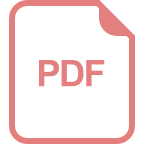
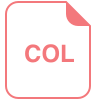
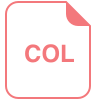