Best Practices for Solving Linear Equations with MATLAB Matrices: Improving Efficiency by Choosing the Right Method, 3 Common Approaches
发布时间: 2024-09-15 01:37:16 阅读量: 35 订阅数: 37 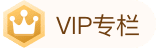
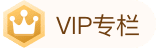
# Best Practices for Solving Linear Equations with MATLAB: Choosing the Right Method for Efficiency, 3 Common Approaches
## 1. Fundamentals of Solving Linear Equations in MATLAB
Linear equation systems are a common problem in mathematics that involve finding a set of unknown variables satisfying a series of linear equations. MATLAB offers a suite of powerful tools to solve linear equation systems, including direct and iterative methods.
In this chapter, we will cover the basic knowledge of solving linear equation systems in MATLAB. We will discuss the mathematical model of linear equation systems and introduce the two main methods of solving linear equation systems in MATLAB: direct methods and iterative methods.
## 2. Theoretical Foundations of Solving Linear Equation Systems in MATLAB
### 2.1 Mathematical Model of Linear Equation Systems
Linear equation systems are a set of equations in the following form:
```
a11x1 + a12x2 + ... + a1nxn = b1
a21x1 + a22x2 + ... + a2nxn = b2
am1x1 + am2x2 + ... + amnxn = bm
```
Where `aij` are the elements of the coefficient matrix, `x1`, `x2`, ..., `xn` are the unknowns, and `b1`, `b2`, ..., `bm` are the elements of the constant vector.
### 2.2 Matrix Inversion Method
The matrix inversion method is a direct method for solving linear equation systems. It obtains the unknowns by inverting the coefficient matrix.
**Steps:**
1. Write the linear equation system in matrix form: `Ax = b`, where `A` is the coefficient matrix, `x` is the unknown vector, and `b` is the constant vector.
2. Find the inverse of the coefficient matrix `A`, `A^-1`.
3. Solve for the unknown vector: `x = A^-1b`.
**Code Example:**
```matlab
% Coefficient matrix
A = [2 1; 3 4];
% Constant vector
b = [5; 11];
% Calculate the inverse of the coefficient matrix
A_inv = inv(A);
% Solve for the unknown vector
x = A_inv * b;
% Display the result
disp(x);
```
**Logical Analysis:**
* The `inv(A)` function is used to calculate the inverse matrix of `A`.
* `x = A_inv * b` computes the unknown vector `x`.
### 2.3 Cramer's Rule
Cramer's rule is another direct method for solving linear equation systems. It obtains the unknowns by calculating the Cramer determinant for each unknown.
**Cramer Determinant:**
For an unknown `xi`, its Cramer determinant is:
```
C_i = det(A_i) / det(A)
```
Where `A_i` is the matrix with the `i`th column replaced by the constant vector `b`, and `det(A)` is the determinant of the coefficient matrix `A`.
**Steps:**
1. Calculate the determinant of the coefficient matrix `A`.
2. For each unknown `xi`, calculate its Cramer determinant `C_i`.
3. Solve for the unknown: `xi = C_i / det(A)`.
**Code Example:**
```matlab
% Coefficient matrix
A = [2 1; 3 4];
% Constant vector
b = [5; 11];
% Calculate the determinant of the coefficient matrix
det_A = det(A);
% Calculate the Cramer determinant for each unknown
C1 = det([b, 1; 3, 4]);
C2 = det([2, b; 3, 11]);
% Solve for the unknowns
x1 = C1 / det_A;
x2 = C2 / det_A;
% Display the result
disp([x1, x2]);
```
**Logical Analysis:**
* The `det(A)` function calculates the determinant of the matrix `A`.
* `det([b, 1; 3, 4])` calculates the Cramer determinant for the unknown `x1`.
* `det([2, b; 3, 11])` calculates the Cramer determinant for the unknown `x2`.
## 3.1 Direct Solution Methods
Direct solution methods involve a series of operations on the coefficient matrix of the linear equation system to transform it into an upper triangular or diagonal matrix, and then solve the equation system through back substitution. Direct solution methods mainly include Gauss elimination and LU decomposition.
#### 3.1.1 Gauss Elimination Method
The Gauss elimination method is a classic direct solution method. Its basic idea is to transform the coefficient matrix into an upper triangular matrix through row transformations, and then solve the equation system through back substitution. The specific steps of the Gauss elimination method are as follows:
1. **Elimination:** Starting with the first equation, perform elimination operations on each equation in turn, i.e., multiply the coefficients of that equation by the corresponding coefficients of other equations and add them to obtain a new equation system. In this new system, the first equation contains only one unknown, the second equation contains only two unknowns, and so on.
2. **Back substitution:** Starting with the last equation, perform back substitution operations on each equation in turn, i.e., use the values of the known unknowns to solve for the values of other unknowns.
Gauss elimination method code example:
```matlab
% Given coefficient matrix A and right-hand vector b
A = [2 1 1; 4 3 2; 8 7 4];
b = [1; 2; 3];
% Perform Gauss elimination
for i = 1:size(A, 1)
```
0
0
相关推荐








