MATLAB Genetic Algorithm Parallel Computing Guide: Accelerating Optimization, Saving Time
发布时间: 2024-09-15 04:46:32 阅读量: 38 订阅数: 36 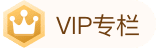
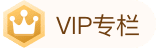
# 1. MATLAB Genetic Algorithm Basics
Genetic Algorithms (GA) are optimization algorithms inspired by biological evolution, which simulate the process of natural selection to solve complex problems. GA works through the following steps:
- **Initialization:** Randomly generate a set called population of potential solutions.
- **Evaluation:** Calculate the fitness of each solution, i.e., the extent to which it solves the problem.
- **Selection:** Select the fittest solutions for reproduction based on their fitness.
- **Crossover:** Combine selected solutions to create new solutions.
- **Mutation:** Introduce random changes in new solutions to introduce diversity.
- **Repeat:** Repeat the above steps until a solution that meets the objective conditions is found.
# 2. MATLAB Parallel Computing Basics
### 2.1 Concepts and Advantages of Parallel Computing
Parallel computing is a computational technique that utilizes multiple processors or computers to execute tasks simultaneously. It enhances computation speed and efficiency by breaking down c***
***pared to serial computing, parallel computing offers the following advantages:
- **Speed Boost:** Parallel computing can significantly reduce computation time, especially when dealing with large datasets or complex computational tasks.
- **Efficiency Enhancement:** Parallel computing can make more efficient use of computational resources, preventing processor idleness.
- **Scalability:** Parallel computing can easily scale to more processors or computers, further enhancing computational power.
### 2.2 MATLAB Parallel Computing Toolbox
MATLAB offers a rich set of parallel computing tools, including:
#### 2.2.1 Parallel Pool
A parallel pool is a tool for managing multiple worker processes for parallel task execution. It allows users to create and manage multiple worker processes and assign tasks to these worker processes.
**Code Block:**
```
% Create a parallel pool
parpool(4);
% Parallelly execute tasks
parfor i = 1:10000
% Execute task
end
% Close the parallel pool
delete(gcp);
```
**Logical Analysis:**
- `parpool(4)` creates a parallel pool with 4 worker processes.
- The `parfor` loop parallelly executes tasks, assigning each iteration to a different worker process.
- `delete(gcp)` closes the parallel pool, releasing resources.
#### 2.2.2 Distributed Computing
Distributed computing is a computational technique that performs tasks in parallel on multiple computers. MATLAB supports distributed computing, allowing users to assign tasks to other computers on the network.
**Code Block:**
```
% Create a distributed computing job
job = createJob('myCluster');
% Add tasks to the job
addTask(job, @myFunction, 0, {input1, input2});
% Submit the job
submit(job);
% Wait for the job to complete
waitFor(job);
% Get job results
results = getAllOutputArguments(job);
```
**Logical Analysis:**
- `createJob('myCluster')` creates a distributed computing job named "myCluster".
- `addTask(job, @myFunction, 0, {input1, input2})` adds a task to the job that calls the function `myFunction`, with input parameters `input1` and `input2`, and dependent on other tasks in the job.
- `submit(job)` submits the job to the distributed computing environment.
- `waitFor(job)` waits for the job to complete.
- `getAllOutputArguments(job)` retrieves the output results of the job.
### 2.3 Performance Optimization of Parallel Computing
To optimize the performance of parallel computing, the following measures can be taken:
- **Task Decomposition:** Decompose computational tasks into smaller sub-tasks to maximize parallelism.
- **Load Balancing:** Ensure that each processor or computer has roughly equal load to avoid resource idleness.
- **Communication Optimization:** Reduce communication overhead between worker processes to improve efficiency.
- **Algorithm Selection:** Choose algorithms suitable for parallel computing to fully utilize parallel resources.
# 3.1 Principles of Parallelizing Genetic Algorithms
The parallelization of genetic algorithms is a technique that leverages parallel computing technology to accelerate the solving process of genetic algorithms. Its basic principle is to decompose the computational tasks of genetic algorithms into multiple independent sub-tasks and then assign these sub-tasks to different processors or computing nodes for parallel execution.
The main advantages of parallelizing genetic algorithms are:
- **Increased Computation Speed:** By parallelly executing computational tasks, the solving time for genetic algorithms can be significantly shortened, especially when dealing with large-scale problems.
- **Improved Algorithm Effici
0
0
相关推荐









