MATLAB Genetic Algorithm and Deep Learning Integration Guide: Empowering Complex Optimization Tasks, Solving Puzzles
发布时间: 2024-09-15 05:02:41 阅读量: 24 订阅数: 30 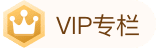
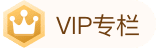
# 1. Introduction to MATLAB Genetic Algorithms
Genetic Algorithms (GA) are optimization algorithms inspired by the process of natural selection. They simulate the biological evolution process to solve complex problems. In MATLAB, various toolboxes and functions can be used to implement GA.
The fundamental principles of GA include:
- **Natural Selection and Fitness Functions:** GA selects individuals for reproduction based on their fitness (i.e., their ability to solve problems). Individuals with higher fitness are more likely to be selected.
- **Crossover and Mutation Operations:** GA creates new individuals through crossover and mutation operations. Crossover combines the genes (solutions) of two individuals, while mutation introduces random changes to explore new solution spaces.
# 2.1 Fundamental Principles of Genetic Algorithms
### 2.1.1 Natural Selection and Fitness Functions
Genetic Algorithm (GA) is an optimization algorithm inspired by Darwin's theory of evolution. It solves complex problems by simulating the biological evolution process. In GA, each potential solution is called an individual, while a group of individuals is known as a population.
The central concept of GA is natural selection, which simulates the process of organisms competing for survival in nature. Each individual has a fitness value that measures the effectiveness of its problem-solving. Individuals with higher fitness are more likely to be selected for reproduction, thereby passing their genes on to the next generation.
The fitness function is a critical component of GA, defining how individual fitness is calculated. The fitness function can be designed according to the specific requirements of the problem. For example, in an image classification task, the fitness function could be the classification accuracy.
### 2.1.2 Crossover and Mutation Operations
Crossover and mutation are the two basic operations used in GA to generate new individuals.
***Crossover:** The crossover operation combines the genes of two parent individuals to produce a child individual. This simulates the process of genetic recombination in organisms.
***Mutation:** The mutation operation randomly changes the genes of the child individual, introducing diversity. This simulates the genetic changes that occur in organisms due to mutations.
Crossover and mutation operations work together to explore the search space and generate individuals with higher fitness. By repeating these operations, GA gradually converges to the optimal or near-optimal solution.
```matlab
% Genetic algorithm parameter settings
populationSize = 100; % Population size
numGenerations = 100; % Number of generations
crossoverProbability = 0.8; % Crossover probability
mutationProbability = 0.1; % Mutation probability
% Initialize population
population = rand(populationSize, numGenes);
% Iterative evolution
for generation = 1:numGenerations
% Calculate fitness
fitness = evaluateFitness(population);
% Selection
parents = selectParents(population, fitness);
% Crossover
offspring = crossover(parents, crossoverProbability);
% Mutation
offspring = mutate(offspring, mutationProbability);
% Update population
population = [population; offspring];
end
% Get the best individual
bestIndividual = population(find(fitness == max(fitness), 1), :);
```
**Line-by-line code logic interpretation:**
1. Set the parameters of the genetic algorithm, including population size, number of generations, crossover probability, and mutation probability.
2. Initialize the population, where each individual consists of a set of randomly generated genes.
3. Iterate the evolutionary process, where each generation includes calculating fitness, selecting parents, crossover, mutation, and updating the population.
4. Calculate the fitness of each individual, measuring its effectiveness in solving problems.
5. Select parents based on fitness, where individuals with higher fitness are more likely to be chosen.
6. Use the crossover operation to combine the genes of the
0
0
相关推荐
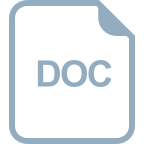
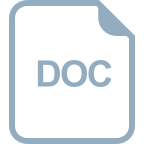
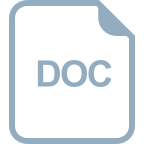
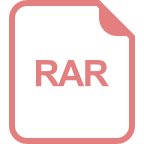
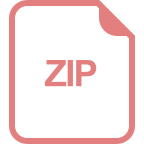
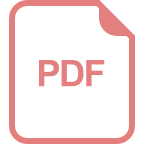
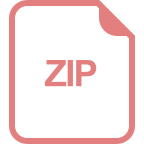
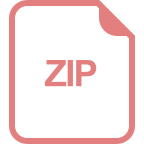
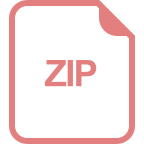