MATLAB Genetic Algorithm Parameter Tuning Guide: Enhancing Performance, Optimizing Results
发布时间: 2024-09-15 04:53:45 阅读量: 18 订阅数: 24 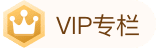
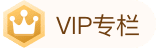
# Genetic Algorithm Parameter Tuning Guide in MATLAB: Enhancing Performance and Optimizing Results
## 1. Overview of Genetic Algorithms
A Genetic Algorithm (GA) is an optimization algorithm inspired by the process of biological evolution. It explores and optimizes solutions in the search space by simulating natural selection and genetic variation. A GA consists of the following key elements:
- **Chromosome:** The encoded structure representing potential solutions.
- **Population:** A group of chromosomes representing the current search space.
- **Selection:** Choosing chromosomes for reproduction based on fitness (the quality of the solution).
- **Crossover:** Combining the genetic information of two chromosomes to produce new offspring.
- **Mutation:** Randomly altering the genetic information of chromosomes at a certain probability to introduce diversity.
## 2. Parameter Tuning of Genetic Algorithms in MATLAB
### 2.1 Population Size and Selection Pressure
**Population Size**
The population size refers to the number of individuals in each generation of a genetic algorithm. It affects the algorithm's exploration and exploitation capabilities. A larger population size can offer greater diversity and increase the chance of finding the optimal solution. However, a larger population size also requires more computational resources.
**Selection Pressure**
Selection pressure refers to the intensity of choosing individuals for reproduction in the algorithm. Higher selection pressure favors better individuals and accelerates the convergence speed. Yet, excessively high selection pressure may lead to premature convergence to local optima.
### 2.2 Crossover and Mutation Probability
**Crossover Probability**
Crossover probability is the likelihood of two parent individuals swapping genes to produce offspring. Higher crossover probability can promote population diversity and increase the chances of the algorithm finding the optimal solution.
**Mutation Probability**
Mutation probability is the likelihood of an individual's genes mutating. Higher mutation probability can introduce new genes and prevent the algorithm from getting stuck in local optima. However, excessively high mutation probability can disrupt individual fitness.
### 2.3 Termination Conditions
**Maximum Generations**
The maximum generations specify the maximum number of generations the algorithm will run. The algorithm will terminate upon reaching the maximum generations.
**Fitness Threshold**
The fitness threshold is a fitness value that, when the average fitness of individuals in the population reaches or exceeds this threshold, the algorithm will terminate.
**Code Example**
```matlab
% Set genetic algorithm parameters
options = gaoptimset('PopulationSize', 100, ...
'SelectionPressure', 1.2, ...
'CrossoverFraction', 0.8, ...
'MutationRate', 0.1, ...
'Generations', 100, ...
'FitnessLimit', 0.95);
% Run genetic algorithm
[x, fval, exitflag, output] = ga(@fitnessFunction, nvars, ...
[], [], [], [], lb, ub, [], options);
```
**Logical Analysis**
* The `gaoptimset` function is used to set genetic algorithm parameters.
* The `PopulationSize` parameter specifies a population size of 100.
* The `SelectionPressure` parameter specifies a selection pressure of 1.2.
* The `CrossoverFraction` parameter specifies a crossover probability of 0.8.
* The `MutationRate` parameter specifies a mutation probability of 0.1.
* The `Generations` parameter specifies a maximum of 100 generations.
* The `FitnessLimit` parameter specifies a fitness threshold of 0.95.
* The `ga` function runs the genetic algorithm, where `fitnessFunction` is the objective function, `nvars` is the number of decision variables, and `lb` and `ub` are the lower and upper bounds of the decision variables.
* `x` is the optimal solution, `fval` is the fitness of the optimal solution, `exitflag` is the algorithm termination flag, and `output` is the algorithm output information.
## 3.1 Optimization Function
In genetic algorithms, the optimization function defines the algorithm's objective, which is the target function that needs to be minimized or maximized. The optimization function can be any evaluable function, typically represented as:
```
f(x)
```
Where:
* `f` is the optimization function.
* `x` is the vector of variables to be optimized.
#### Types of Optimization Functions
Optimization functions can be divided into two categories:
***Continuous optimization functions:** Variable `x` is continuous and can take any real value.
***Discrete optimization functions:** Variable `x` is discrete and can only take a finite number of values.
#### Evaluation of Optimization Functions
Genetic algorithms guide the search process by evaluating the optimization function. The number of evaluations of the optimization function is a key factor affecting the algorithm's performance. The more evaluations, the better the solution the algorithm typically finds, but the computational cost will also be higher.
#### Constraints on Optimization Functions
Optimization functions may be subject to constraints. Constraints define the feasible range of values for variable `x`. Genetic algorithms can handle constraints through the following methods:
***Penalty Function Method:** So
0
0
相关推荐
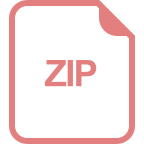
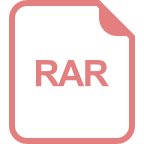
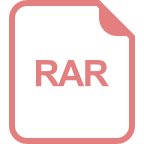
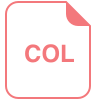
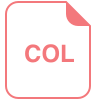
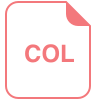
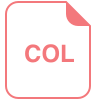
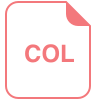
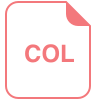