MATLAB Genetic Algorithm vs Other Optimization Algorithms: A Comprehensive Analysis of Pros and Cons, Choosing the Right Algorithm for Twice the Work in Half the Time
发布时间: 2024-09-15 04:45:24 阅读量: 63 订阅数: 35 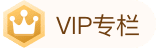
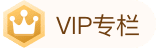
# 1. Overview of Optimization Algorithms
Optimization algorithms are mathematical tools used to find the optimal solution to a given problem. They are widely applied in fields such as engineering, science, and finance.
Optimization algorithms generally follow an iterative process, where the algorithm evaluates the current solution and generates a new solution in each iteration. This process continues until a termination condition is met, such as reaching the maximum number of iterations or finding a solution that satisfies specific criteria.
There are various types of optimization algorithms, each with its unique advantages and disadvantages. When choosing an optimization algorithm, consider the nature of the problem, the required accuracy, and the available computational resources.
# 2. Genetic Algorithms
Genetic Algorithms (GA) are heuristic optimization algorithms inspired by the biological evolution process. They simulate natural selection, crossover, and mutation mechanisms to search for the optimal solution in the solution space.
## 2.1 Fundamental Principles of Genetic Algorithms
### 2.1.1 Natural Selection and Fitness
In GA, the solutions to the problem are represented as chromosomes, each composed of a series of genes. The fitness of a chromosome is determined by an objective function that measures the effectiveness of the chromosome in solving the problem. Chromosomes with higher fitness are more likely to be selected for reproduction.
### 2.1.2 Crossover and Mutation
The crossover operation combines the genes of two parent chromosomes to produce a new offspring chromosome. The mutation operation randomly changes the genes of the offspring chromosome, introducing diversity and preventing the algorithm from getting stuck in local optimum solutions.
## 2.2 Implementation of Genetic Algorithms in MATLAB
### 2.2.1 MATLAB Genetic Algorithm Toolbox
MATLAB provides a genetic algorithm toolbox containing functions and classes for implementing GA. The toolbox provides predefined fitness functions, crossover and mutation operators, and classes for managing populations and selection operations.
```matlab
% Using MATLAB Genetic Algorithm Toolbox
ga = gaoptimset('PopulationSize', 100, 'Generations', 100);
[x, fval, exitflag, output] = ga(@(x) -x^2, 1);
```
### 2.2.2 Implementing Genetic Algorithms Manually
GA can also be implemented manually, providing greater flexibility and allowing custom fitness functions, crossover, and mutation operators.
```matlab
% Manually implement genetic algorithms
population = rand(100, 10); % Randomly initialize population
for i = 1:100 % Iteration count
% Calculate fitness
fitness = -population.^2;
% Selection
parents = selection(population, fitness);
% Crossover
children = crossover(parents);
% Mutation
children = mutation(children);
% Update population
population = [parents; children];
end
```
## Line-by-line Code Logic Interpretation:
1. `population = rand(100, 10);`: Randomly initialize a population of 100 individuals with 10 genes each.
2. `for i = 1:100`: Start an iteration loop, iterate 100 times.
3. `fitness = -population.^2;`: Calculate the fitness of each individual using the negative square function as the objective function.
4. `parents = selection(population, fitness);`: Select parent individuals based on fitness.
5. `children = crossover(parents);`: Perform crossover on parent individuals to produce offspring.
6. `children = mutation(children);`: Perform mutation on offspring individuals to introduce diversity.
7. `population = [parents; children];`: Update the population by merging parent and offspring individuals.
# 3. Other Optimization Algorithms
While genetic algorithms are powerful optimization algorithms, they are not the only ones. In MATLAB, there are many other optimization algorithms available, each with its unique advantages and disadvantages. This chapter will introduce three popular optimization algorithms: Particle Swarm Optimization, Simulated Annealing, and Ant Colony Optimization.
### 3.1 Particle Swarm Optimization Algorithm
#### 3.1.1 Basic Principles of Particle Swarm Optimization
The Particle Swarm Optimization Algorithm (PSO) is an optimization algorithm inspired by the behavior of bird flocks or fish schools. In PSO, each particle represents a potential solution, and these particles move through the search space to find the best solution. Each particle has the following attributes:
* Position: The current location of the particle, representing a potential solution.
* Velocity: The speed of the particle, indicating the direction of movement in the search space.
* Personal best position (pbest): The best position found by the particle.
* Global best position (gbest): The best position found by all particles.
Particles update their speed and position using the following formulas:
```
v_i(t+1) = w * v_i(t) + c1 * rand() * (pbest_i(t) - x_i(t)) + c2 * rand(
```
0
0
相关推荐








