MATLAB Genetic Algorithm Guide for Big Data Optimization: Tackling the Challenges of Massive Data, Unearthing Data Value
发布时间: 2024-09-15 05:01:40 阅读量: 25 订阅数: 27 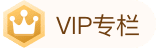
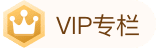
# Guide to MATLAB Genetic Algorithm for Big Data Optimization: Overcoming Massive Data Challenges and Unlocking Data Value
# 1. Introduction to Genetic Algorithms**
Genetic algorithms (GAs) are optimization algorithms inspired by the theory of evolution, which mimic the process of natural selection and genetic inheritance in organisms. GAs iteratively search for the optimal solution through the following steps:
- **Initialization:** Randomly generate a population where each individual represents a potential solution.
- **Selection:** Select individuals for reproduction based on their fitness (objective function value).
- **Crossover:** Pair selected individuals and exchange genes to produce new offspring.
- **Mutation:** Introduce random modifications to the new offspring to introduce diversity.
- **Iteration:** Repeat the selection, crossover, and mutation steps until a termination condition is met (e.g., maximum iterations or convergence).
# 2. Implementation of Genetic Algorithms in MATLAB**
## 2.1 MATLAB Code Framework for Genetic Algorithms
The implementation of genetic algorithms in MATLAB mainly involves the following steps:
1. **Initialize the population:** Create a set of random solutions, known as a population.
2. **Evaluate fitness:** Calculate the fitness of each solution, which is the value of its objective function.
3. **Selection:** Select the fittest solutions to produce the next generation based on their fitness.
4. **Crossover:** Combine two selected solutions to produce new offspring.
5. **Mutation:** Randomly modify the new offspring to explore the search space.
6. **Termination condition:** The algorithm stops when it reaches the maximum number of iterations or meets specific termination criteria.
The following code snippet demonstrates the basic framework of a genetic algorithm in MATLAB:
```matlab
% Initialize population
population = InitializePopulation(populationSize);
% Evaluate fitness
fitness = EvaluateFitness(population);
% Iterate genetic algorithm
for i = 1:maxIterations
% Selection
selectedParents = SelectParents(population, fitness);
% Crossover
newPopulation = Crossover(selectedParents);
% Mutation
newPopulation = Mutate(newPopulation);
% Evaluate fitness
fitness = EvaluateFitness(newPopulation);
% Update population
population = newPopulation;
end
% Return the best solution
bestSolution = population(1);
```
## 2.2 Setting and Optimizing Genetic Algorithm Parameters
The parameters of genetic algorithms can significantly affect the performance of the algorithm. Key parameters include:
- **Population size:** The number of solutions in the population.
- **Crossover probability:** The probability of crossover operations occurring.
- **Mutation probability:** The probability of mutation operations occurring.
- **Maximum number of iterations:** The maximum number of iterations the algorithm will run.
Parameter optimization can be performed through the following methods:
- **Rule of thumb:** Use经验值 as initial settings and adjust based on algorithm performance.
- **Grid search:** Systematically explore the parameter space to find the optimal combination.
- **Adaptive parameters:** Dynamically adjust parameters based on the progress of the algorithm.
## 2.3 Performance Evaluation of Genetic Algorithms
The performance of genetic algorithms can be evaluated using the following metrics:
- **Convergence:** The time taken by the algorithm to find the best solution.
- **Robustness:** The stability of the algorithm with different inputs and parameter settings.
- **Efficiency:** The utilization of computing resources by the algorithm.
Performance evaluation is crucial to determining whether a genetic algorithm is suitable for a specific optimization problem.
# 3. Application of Genetic Algorithms in Big Data Optimization
### 3.1 Characteristics and Challenges of Big Data Optimization Problems
Compared to traditional optimization problems, big data optimization problems have the following characteristics and challenges:
- **Massive data volume:
0
0