MATLAB Genetic Algorithm Constraint Handling Guide: Solving Complex Optimization Problems and Breaking Through Limitations
发布时间: 2024-09-15 04:55:06 阅读量: 31 订阅数: 35 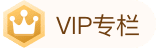
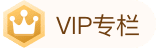
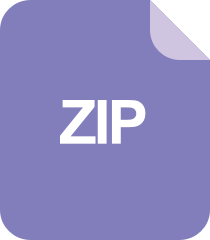
《COMSOL顺层钻孔瓦斯抽采实践案例分析与技术探讨》,COMSOL模拟技术在顺层钻孔瓦斯抽采案例中的应用研究与实践,comsol顺层钻孔瓦斯抽采案例 ,comsol;顺层钻孔;瓦斯抽采;案例,COM
# MATLAB Genetic Algorithm Constraint Handling Guide: Overcoming Limits to Solve Complex Optimization Problems
## 1.1 Fundamental Principles of Genetic Algorithms
A genetic algorithm is an optimization algorithm inspired by the theory of natural evolution. It simulates the process of biological evolution, constantly optimizing a population of candidate solutions through operations such as selection, crossover, and mutation, ultimately finding the optimal solution.
Genetic algorithms encode candidate solutions as chromosomes, with each chromosome consisting of a set of genes. Genes represent the values of different parameters in the candidate solution. The algorithm selects superior individuals for the next generation based on the fitness of the chromosome (i.e., the value of the objective function) through selection operations. Crossover operations exchange parts of the genes between two chromosomes to produce new individuals. Mutation operations randomly alter certain genes in the chromosome to introduce diversity.
## 2. Implementation of Genetic Algorithms in MATLAB
### 2.1 Introduction to the MATLAB Genetic Algorithm Toolbox
MATLAB provides a powerful genetic algorithm toolbox that offers a complete framework for users to implement genetic algorithms. This toolbox includes a series of functions for creating, configuring, and running genetic algorithms, streamlining the development and application of genetic algorithms.
```matlab
% Create a genetic algorithm object
ga = gaoptimset;
```
### 2.2 Genetic Algorithm Parameter Settings and Optimization
The performance of a genetic algorithm largely depends on its parameter settings. The MATLAB genetic algorithm toolbox allows users to specify and adjust various parameters, including:
- **Population size:** The number of individuals in the population.
- **Maximum number of generations:** The maximum number of iterations the algorithm runs.
- **Crossover probability:** The probability of two parent individuals exchanging genes.
- **Mutation probability:** The probability of an individual's genes mutating.
- **Selection method:** The algorithm used to select parent individuals, such as roulette wheel selection or tournament selection.
```matlab
% Set genetic algorithm parameters
ga.PopulationSize = 100;
ga.MaxGenerations = 100;
ga.CrossoverFraction = 0.8;
ga.MutationRate = 0.05;
ga.SelectionFcn = @selectionroulette;
```
**Parameter Optimization:**
To achieve optimal performance, genetic algorithm parameters can be optimized. The MATLAB genetic algorithm toolbox provides optimization functions, such as `gaoptimset`, for automatically adjusting parameters to suit specific problems.
```matlab
% Use gaoptimset to optimize parameters
ga = gaoptimset(ga, 'Display', 'iter', 'PlotFcns', @gaplotbestf);
```
**Logical Analysis:**
- `PopulationSize`: A larger population size can enhance the algorithm's search capability but increases computation time.
- `MaxGenerations`: A larger maximum number of iterations increases the chance of algorithm convergence but also increases computation time.
- `CrossoverFraction`: A higher crossover probability can promote population diversity, but too high a probability may lead to premature convergence.
- `MutationRate`: A higher mutation probability can prevent the algorithm from getting stuck in local optima, but too high a probability may damage valuable genes.
- `SelectionFcn`: Different selection methods affect the convergence speed and final results of the algorithm.
## 3. Genetic Algorithm Constraint Handling
### 3.1 Overview of Constraint Handling Methods
When solving constrained optimization problems, genetic algorithms face the challenge of dealing with constraint conditions. Constraint conditions limit the feasible solution space, and if not properly handled, the algorithm may get stuck in infeasible solutions or converge to local optima. To address this issue, various constraint handling methods have been proposed, each with its own advantages and disadvantages.
### 3.2 Penalty Function Method
The penalty function method is a widely used constraint handling approach. Its basic idea is to convert constraint conditions into penalty terms and add them to the objective function. By adjusting the penalty coefficient, the degree of violation of the constraint conditions can be controlled. The penalty function method is easy to implement, but it may lead to slower convergence and requires careful selection of the penalty coefficient.
**Code Block:**
```matlab
function fitness = fitnessFunction(chromosome)
% Calculate the objective function value
objectiveValue = calculateObjective(chromosome);
% Calculate the penalty term for constraint violations
penalty = 0;
for i = 1:numConstraints
constraintValue = calculateConstraint(chromosome, i);
if constraintValue > 0
penalty = penalty + constraintValue^2;
end
end
% Return the fitness value after penalization
fitness = objectiveValue + penalty * penaltyFactor;
end
```
**Logical Analysis:**
This
0
0
相关推荐





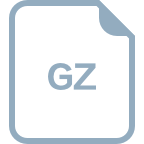
