Advanced Techniques in MATLAB Genetic Algorithm: Ultimate Weapon for Optimization Challenges
发布时间: 2024-09-15 04:41:22 阅读量: 44 订阅数: 35 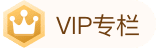
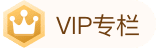
#MATLAB Genetic Algorithm Advanced Techniques Unveiled: The Ultimate Weapon for Optimization Puzzles
# 1. Fundamentals of MATLAB Genetic Algorithms**
Genetic algorithms (GAs) are optimization algorithms inspired by the theory of evolution, which simulate the process of natural selection to solve complex problems. MATLAB provides a Genetic Algorithm and Direct Search toolbox for implementing and optimizing GA.
The basic principles of GA include:
- **Population:** A group of candidate solutions, each represented by a set of genes.
- **Selection:** Choosing individuals for reproduction based on their fitness values.
- **Crossover:** Combining genes from two parent individuals to create new offspring.
- **Mutation:** Randomly altering the genes of offspring to introduce diversity.
# 2. Optimization Techniques for Genetic Algorithms
### 2.1 Parameter Optimization for Genetic Algorithms
Genetic algorithms are optimization algorithms based on natural selection and genetic principles. Their performance largely depends on the settings of their parameters. Here are some optimization tips for key parameters of genetic algorithms:
#### 2.1.1 Population Size and Generations
**Population size** refers to the number of individuals in the algorithm. A larger population size can enhance the algorithm's exploration ability but also increases computation time. Typically, the population size should be adjusted based on the complexity of the problem and the size of the search space.
**Generations** refer to the number of iterations the algorithm undergoes. More generations can improve the algorithm's convergence accuracy but also increase computation time. The setting of generations should consider the complexity of the problem and the convergence speed of the algorithm.
#### 2.1.2 Selection Strategy and Crossover Probability
**Selection strategy***mon selection strategies include roulette wheel selection, tournament selection, and elitism. Different selection strategies will affect the convergence speed and diversity of the algorithm.
**Crossover probability** refers to the likelihood of an individual undergoing a crossover operation. A higher crossover probability can enhance the algorithm's exploration ability but also increase its destructiveness. The setting of crossover probability should be adjusted based on the complexity of the problem and the convergence speed of the algorithm.
#### 2.1.3 Mutation Probability and Mutation Operators
**Mutation probability** refers to the likelihood of an individual undergoing a mutation operation. A higher mutation probability can enhance the algorithm's exploration ability but also increase its randomness. The setting of mutation probability should be adjusted based on the complexity of the problem and the convergence speed of the algorithm.
**Mutation operators***mon mutation operators include single-point mutation, multi-point mutation, and Gaussian mutation. Different mutation operators will affect the algorithm's exploration ability and convergence speed.
### 2.2 Handling Constraint Conditions
Genetic algorithms may encounter constraint conditions during optimization. Methods for handling constraint conditions include:
#### 2.2.1 Penalty Function Method
The **penalty function method** deals with constraint conditions by adding a penalty term to the objective function. The value of the penalty term is proportional to the degree of constraint violation. This method is simple and easy to use, but may cause the algorithm to converge to suboptimal solutions.
#### 2.2.2 Feasible Domain Restriction Method
The **feasible domain restriction method** handles constraint conditions by restricting individuals to search within the feasible domain. This method can ensure that the algorithm finds feasible solutions but may limit the algorithm's exploration ability.
### 2.3 Multi-objective Optimization
Genetic algorithms can be used to optimize problems with multiple objectives. Methods for handling multi-objective optimization include:
#### 2.3.1 Weighted Sum Method
The **weighted sum method** deals with multi-objective optimization by forming a single objective function from the weighted sum of multiple objectives. This method is simple and easy to use, but may cause the algorithm to converge to solutions that are sensitive to weight settings.
#### 2.3.2 NSGA-II Algorithm
The **NSGA-II algorithm** is a genetic algorithm specifically designed for multi-objective optimization. The algorithm uses non-dominated sorting and crowding distance calculations to select individuals for crossover and mutation. The NSGA-II algorithm can find a set of Pareto optimal solutions, i.e., solutions where it is impossible to improve one objective without impairing the others.
# 3. Practical Applications of MATLAB Genetic Algorithms
### 3.1 Function Optimization
#### 3.1.1 Classic Function Optimization Examples
Genetic algorithms have wide applications in function optimization. Classic function optimization examples include:
- **Rosenbrock Function:** A non-convex function with multiple local optima, used to test the algorithm's global search ability.
- **Rastrigin Function:** A function with a large number of local optima, used to evaluate the algorithm's local search ability.
- **Sphere Function:** A simple convex function, used to compare the convergence speed of different algorithms.
#### 3.1.2 Multi-peak Function Optimization Challenges
For multi-peak functions, genetic algorithms face the challenge of avoiding local optima. Methods to solve this challenge include:
- **Increasing Population Size:** Expanding the search space and increasing the likelihood of finding the global optimal solution.
- **Adjusting Mutation Probability:** Increasing the mutation probability can help the algorithm explore a wider search space.
- **Using Hybrid Algorithms:** Combining genetic algorithms with other optimization algorithms, such as particle swarm optimization, can improve global search ability.
### 3.2 Image Processing
#### 3.2.1 Image Enhancement Optimization
Genetic algorithms can be used to optimize image enhancement parameters, such as contrast, brightness, and sharpness. By minimizing image quality metrics, such as peak signal-to-noise ratio (PSNR) or structural similarity (SSIM), the optimal parameter combination can be found.
#### 3.2.2 Image Segmentation Optimization
Genetic algorithms can also be used to optimize the parameters of image segmentation algorithms. For example, in threshold segmentation, genetic algorithms can find the optimal threshold to maximize segmentation quality.
### 3.3 Machine Learning
#### 3.3.1 Neural Network Hyperparameter Optimization
Genetic algorithms can be used to optimize hyperparameters of neural networks, such as learning rate, weight decay, and number of layers. By minimizing the loss function on the validation set, the optimal hyperparameter combination can be found.
#### 3.3.2 Support Vector Machine Model Selection
Genetic algorithms can be used to select the best kernel function and regularization parameters for support vector machine (SVM) models. The best parameter combination in terms of performance on the training and test sets can be found through cross-validation.
**Code Block 1: MATLAB Genetic Algorithm Function Optimization Example**
```matlab
% Define Rosenbrock function
rosenbrock = @(x) 100 * (x(2) - x(1)^2)^2 + (1 - x(1))^2;
% Set genetic algorithm parameters
options = gaoptimset('PopulationSize', 50, 'Generations', 100);
% Run genetic algorithm
[x_opt, fval] = ga(rosenbrock, 2, [], [], [], [], [-5, -5], [5, 5], [], options);
% Output optimal solution
disp(['Optimal solution: ', num2str(x_opt)]);
disp(['Optimal value: ', num2str(fval)]);
```
**Logical Analysis:**
This code block demonstrates an example of using MATLAB genetic algorithms to optimize the Rosenbrock function. The `gaoptimset` function is used to set genetic algorithm parameters such as population size and number of generations. The `ga` function runs the genetic algorithm and returns the optimal solution and value.
**Parameter Explanation:**
- `rosenbrock`: The objective function (Rosenbrock function).
- `2`: The number of variables (The Rosenbrock function has 2 variables).
- `[]`: Linear constraints (None).
- `[]`: Non-linear constraints (None).
- `[]`: Initial population (Randomly generated).
- `[-5, -5]`: Variable lower bounds.
- `[5, 5]`: Variable upper bounds.
- `[]`: Other options (None).
- `options`: Genetic algorithm parameters.
# 4. Advanced Extensions of Genetic Algorithms
### 4.1 Distributed Genetic Algorithms
Distributed genetic algorithms (DGA) improve the efficiency and scalability of genetic algorithms by distributing the population across different subpopulations and allowing communication between them. There are two main ways to implement DGA:
**4.1.1 Parallel Computing**
Parallel computing improves computation speed by dividing the population into multiple subpopulations and executing them in parallel on different processors or computers. Each subpopulation evolves independently and periodically exchanges individuals with other subpopulations.
```matlab
% Parallel genetic algorithm
parfor i = 1:num_subpopulations
% Execute genetic algorithm in each subpopulation
[best_individual, best_fitness] = ga(..., 'SubpopulationSize', subpop_size);
% Send the best individual to the main population
best_individuals(i) = best_individual;
best_fitnesses(i) = best_fitness;
end
```
**4.1.2 Island Model**
The island model divides the population into multiple isolated subpopulations, with each subpopulation evolving on its own "island." Occasionally, individuals migrate between islands to promote diversity and prevent the population from getting stuck in local optima.
```matlab
% Island model genetic algorithm
for i = 1:num_islands
% Execute genetic algorithm on each island
[best_individual, best_fitness] = ga(..., 'MigrationInterval', migration_interval);
% Send the best individual to the main population
best_individuals(i) = best_individual;
best_fitnesses(i) = best_fitness;
end
```
### 4.2 Multimodal Optimization
Genetic algorithms may struggle when optimizing functions with multiple local optima. Multimodal optimization techniques aim to solve this problem by promoting population diversity and exploring different search areas.
**4.2.1 Hybrid Genetic Algorithms**
Hybrid genetic algorithms combine genetic algorithms with other optimization algorithms to enhance their exploration capabilities. For example, genetic algorithms can be combined with simulated annealing or particle swarm optimization algorithms.
```matlab
% Hybrid genetic algorithm
% Genetic algorithm phase
[pop, fitness] = ga(...);
% Simulated annealing phase
temperature = initial_temperature;
while temperature > cooling_rate
% Randomly select an individual
individual = pop(randi(size(pop, 1)));
% Produce a mutated individual
mutant = mutate(individual);
% Calculate the fitness of the mutated individual
mutant_fitness = evaluate(mutant);
% Accept or reject the mutation based on Metropolis-Hastings criterion
if mutant_fitness > fitness || rand() < exp((mutant_fitness - fitness) / temperature)
pop(pop == individual) = mutant;
fitness(pop == individual) = mutant_fitness;
end
% Decrease temperature
temperature = temperature * cooling_rate;
end
```
**4.2.2 Particle Swarm Optimization Algorithm**
Particle swarm optimization (PSO) is an optimization algorithm based on swarm intelligence. Particles in PSO explore the search space by sharing information and updating their positions.
```matlab
% Particle swarm optimization algorithm
% Initialize particle swarm
particles = initialize_particles(num_particles);
% Iteratively update particle swarm
for i = 1:num_iterations
% Update particle velocity and position
particles = update_particles(particles);
% Evaluate particle fitness
fitness = evaluate(particles);
% Update the best particle
[best_particle, best_fitness] = find_best_particle(particles, fitness);
% Update particle best positions
particles = update_best_positions(particles, best_particle);
end
```
### 4.3 Evolution Strategies
Evolution strategies (ES) are optimization algorithms based on probability distributions. ES uses a covariance matrix to guide the search direction of the population and updates the distribution through mutation and selection.
**4.3.1 Covariance Matrix Adapting Evolution Strategy**
The covariance matrix adapting evolution strategy (CMA-ES) is an adaptive evolution strategy that continuously adjusts the covariance matrix to optimize the search direction.
```matlab
% Covariance matrix adapting evolution strategy
% Initialize parameters
mean = initial_mean;
covariance = initial_covariance;
% Iteratively update distribution
for i = 1:num_iterations
% Generate samples
samples = sample_gaussian(mean, covariance, num_samples);
% Evaluate sample fitness
fitness = evaluate(samples);
% Update distribution parameters
[mean, covariance] = update_parameters(mean, covariance, samples, fitness);
end
```
**4.3.2 Natural Gradient Evolution Strategy**
The natural gradient evolution strategy (NES) is an evolution strategy that uses the natural gradient instead of the traditional gradient to guide the search direction. The natural gradient considers the curvature of the search space, allowing for more efficient exploration of complex functions.
```matlab
% Natural gradient evolution strategy
% Initialize parameters
mean = initial_mean;
covariance = initial_covariance;
% Iteratively update distribution
for i = 1:num_iterations
% Generate samples
samples = sample_gaussian(mean, covariance, num_samples);
% Evaluate sample fitness
fitness = evaluate(samples);
% Calculate the natural gradient
natural_gradient = compute_natural_gradient(samples, fitness);
% Update distribution parameters
[mean, covariance] = update_parameters(mean, covariance, natural_gradient);
end
```
# 5. MATLAB Genetic Algorithm Application Cases**
Genetic algorithms have extensive real-world applications, and here are some MATLAB genetic algorithm application cases:
**5.1 Supply Chain Management Optimization**
Genetic algorithms can be used to optimize supply chain management, such as:
- **Inventory Management:** Optimizing inventory levels to maximize service levels and minimize costs.
- **Logistics Planning:** Optimizing delivery routes and vehicle allocation to improve efficiency and reduce costs.
- **Production Planning:** Optimizing production plans to balance demand and capacity, maximizing profits.
**5.2 Logistics Distribution Optimization**
Genetic algorithms can be used to optimize logistics distribution, such as:
- **Vehicle Routing Planning:** Optimizing vehicle routes to minimize driving distance and time.
- **Loading Optimization:** Optimizing cargo loading to maximize space utilization and safety.
- **Warehouse Management:** Optimizing warehouse layout and inventory allocation to improve efficiency.
**5.3 Financial Portfolio Optimization**
Genetic algorithms can be used to optimize financial portfolios, such as:
- **Asset Allocation:** Optimizing the allocation of different asset classes in the portfolio to achieve risk and return goals.
- **Stock Selection:** Optimizing stock selection to maximize portfolio returns.
- **Risk Management:** Optimizing the portfolio to manage risk and maximize returns.
0
0
相关推荐








