【MATLAB Genetic Algorithm Performance Booster】: Expert Optimization Strategies and Practical Guide
发布时间: 2024-09-15 03:50:44 阅读量: 42 订阅数: 22 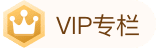
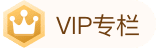
# Chapter 1: Introduction to Genetic Algorithms and MATLAB Implementation Overview
A genetic algorithm is a search and optimization algorithm inspired by the principles of natural selection and genetics, reflecting the Darwinian idea of "survival of the fittest, elimination of the unfit". Implementing a genetic algorithm in MATLAB is an effective means to analyze and solve optimization problems. This chapter will introduce the basic concepts of genetic algorithms, fundamental methods for their implementation in MATLAB, and discuss their applications in solving problems.
Genetic algorithms simulate the process of biological evolution, iteratively optimizing individuals within a population through operations such as selection, crossover, and mutation, to find the optimal solution within the given problem space. MATLAB, as a high-performance numerical computing language, provides a genetic algorithm toolbox that makes the algorithm implementation more intuitive and user-friendly.
This chapter will start with the basic principles of genetic algorithms and delve into their specific implementation in MATLAB. Through some simple examples, the reader will be shown how to use MATLAB's built-in genetic algorithm toolbox to solve practical problems, laying the foundation for subsequent chapters on parameter optimization and deep application of the algorithm.
# Chapter 2: Key Parameters Optimization of Genetic Algorithms
## 2.1 Introduction to Genetic Algorithm Parameters
### 2.1.1 Population Size and Generations Settings
The performance of a genetic algorithm largely depends on the size of the population and the number of generations it iterates. The population size determines the breadth of the search space, while the number of generations dictates the depth of the search process. In practice, choosing the population size requires a balance between exploration and exploitation. A larger population helps maintain diversity and prevents the algorithm from converging prematurely to a local optimum, but it also increases computational costs. The number of generations determines how long the algorithm can run. Generally, longer runtime means a better chance of finding an optimal solution, but it may also lead to overfitting, especially when evaluating the quality of solutions in real-world problems is costly.
The basic code for setting population size and generations in MATLAB is as follows:
```matlab
% Set genetic algorithm parameters
options = optimoptions('ga', 'PopulationSize', 100, 'MaxGenerations', 100, ...);
```
In the above code, `PopulationSize` is set to 100, indicating the number of individuals per generation, and `MaxGenerations` is set to 100, indicating that the algorithm will run for 100 generations. Optimizing these parameters requires multiple trials based on specific problems to determine the best configuration.
### 2.1.2 Selection, Crossover, and M***
***mon strategies include roulette wheel selection, tournament selection, etc. The crossover strategy pairs selected individuals and exchanges their genes; common methods include single-point crossover, multi-point crossover, and uniform crossover. The mutation strategy introduces new genetic variations by randomly changing certain genes in individuals; common methods include bit-flip mutation, uniform mutation, etc. Adjusting the parameters of these strategies can further refine the algorithm's search behavior to adapt to the characteristics of specific problems.
In MATLAB's genetic algorithm toolbox, the following code can be used for strategy and parameter adjustments:
```matlab
% Set selection, crossover, and mutation strategies
options = optimoptions(options, 'SelectionFunction', @selectionstochunif, ...
'CrossoverFunction', @crossoverintermediate, ...
'MutationFunction', @mutationuniform);
```
In this code, `SelectionFunction`, `CrossoverFunction`, and `MutationFunction` set the functions for selection, crossover, and mutation, respectively, and can be replaced with other built-in functions or custom functions as needed by the user.
## 2.2 Performance Evaluation of Genetic Algorithms
### 2.2.1 Convergence Speed and Solution Quality Analysis
Convergence speed is an important indicator of genetic algorithm performance, referring to the number of iterations required for the algorithm to find a satisfactory solution. Evaluating convergence speed often involves statistical analysis of the accuracy and stability of the solutions. Solution quality is typically measured by the value of the fitness function, which needs to accurately reflect the goodness of the solution.
Here is a simple MATLAB code block for analyzing the convergence performance of a genetic algorithm:
```matlab
% Record the best fitness value for each generation
fitness = zeros(options.MaxGenerations, 1);
for gen = 1:options.MaxGenerations
[sol, fval, exitflag, output, population, scores] = ga(@fitnessfun, nvars, ...
[], [], [], [], [], [], [], options);
fitness(gen) = fval;
end
% Plot the convergence curve
figure;
plot(1:options.MaxGenerations, fitness);
xlabel('Generation');
ylabel('Best Fitness');
title('Convergence plot');
```
### 2.2.2 Algorithm Stability and Robustness Assessment
Algorithm stability reflects the consistency of results obtained under different running conditions. Robustness indicates the algorithm's ability to adapt to changes in problem parameters. Stability assessment usually involves multiple runs of the algorithm and analysis of the result distribution; robustness assessment requires testing the algorithm's performance on different problem instances.
MATLAB provides a method to assess the stability of genetic algorithms:
```matlab
% Run the genetic algorithm multiple times
numRounds = 10; % Number of runs
bestFitness = zeros(numRounds, 1); % Store the best fitness value for each run
for i = 1:numRounds
[sol, fval, exitflag, output, population, scores] = ga(@fitnessfun, nvars, ...
[], [], [], [], [], [], [], options);
bestFitness(i) = fval;
end
% Analyze stability
meanFitness = mean(bestFitness);
stdFitness = std(bestFitness);
% Output statistical information
fprintf('Average best fitness value: %f\n', meanFitness);
fprintf('Fitness standard deviation: %f\n', stdFitness);
```
## 2.3 Advanced Parameter Adjustment Techniques
### 2.3.1 Adaptive and Dynamic Parameter Adjustments
To further improve the performance of genetic algorithms, introducing adaptive and dynamic parameter adjustments is an effective method. Adaptive strategies allow parameters to automatically adjust as the algorithm runs, better adapting to the current search state. Dynamic parameter adjustments change parameter values at certain stages of the algorithm to respond to changes in the quality of solutions or to stagnation in the search process.
In MATLAB, the following code can be used to implement a simple adaptive mutation rate:
```matlab
% Adaptive mutation rate
initialMutationRate = 0.01; % Initial mutation rate
mutationRate = initialMutationRate;
for gen = 1:options.MaxGenerations
% Update mutation rate
if ismember(gen, floor(options.MaxGenerations * [0.25, 0.75]))
mutationRate = mutationRate * 2;
end
options = optimoptions(options, 'MutationFcn', {@mutationuniform, mutationRate});
% Run genetic algorithm
[sol, fval, exitflag, output, population, scores] = ga(@fitnessfun, nvars, ...
[], [], [], [], [], [], [], options);
end
```
### 2.3.2 Multi-Objective Optimization Parameter Settings
In multi-objective optimization problems, genetic algorithms need to be adjusted to optimize multiple conflicting objectives simultaneously. The parameter settings for multi-objective optimization must consider the trade-offs between objectives and the decision-maker's preferences for different objectives. In MATLAB, functions such as NSGA-II, SPEA2, etc., for multi-objective genetic algorithms can be used, and the algorithm's behavior can be controlled by adjusting the corresponding parameters.
An example of MATLAB code for a multi-objective optimization problem is as follows:
```matlab
% Define multi-objective problem
multiObjFun = @(x)[x(1)^2, (x(2)-2)^2];
% Configure options for multi-objective genetic algorithm
options = optimoptions('gamultiobj', 'PopulationSize', 100, ...
'ParetoFraction', 0.35, 'MaxGenerations', 150);
% Run the multi-objective genetic algorithm
[x, fval] = gamultiobj(multiObjFun, 2, [], [], [], [], [], [], [], options);
% Output results
disp('Pareto front solutions');
disp(x);
disp('Pareto front objective values');
disp(fval);
```
In the above code, `gamultiobj` is a built-in MATLAB function for solving multi-objective optimization problems using genetic algorithms. By adjusting parameters such as `PopulationSize`, `ParetoFraction`, and `MaxGenerations`, the algorithm's performance can be optimized to suit specific multi-objective problems.
# Chapter 3: In-Depth Application of MATLAB Genetic Algorithm Toolbox
## 3.1 Built-In Functions and Usage Strategies of the Toolbox
### 3.1.1 Introduction to Common Functions and Case Analysis
The MATLAB genetic algorithm toolbox offers a series of built-in functions that simplify the implementation process of genetic algorithms and provide powerful customization capabilities. Here are several commonly used built-in functions and their applications:
1. `ga` - The basic genetic algorithm function. It can solve both linear and nonlinear optimization problems.
2. `gamultiobj` - The multi-objective genetic algorithm function, specifically used for optimization problems with multiple objectives to be optimized simultaneously.
3. `Hybrid Function` - Allows further optimization using local search techniques after the basic iteration process of the genetic algorithm.
**Case Analysis**: Suppose we need to solve a multi-objective optimization problem where one objective is to maximize efficiency and another is to minimize costs. We can use the `gamultiobj` function as follows:
```matlab
function multiobj_demo()
% Define the objective function
fun = @(x)deal(x(1)^2 + x(2)^2, (1-x(1))^2 + (1-x(2))^2);
% Define the variable bounds
lb = [0, 0];
ub = [1, 1];
% Call the gamultiobj function
[x, fval] = gamultiobj(fun, 2, [], [], [], [], lb, ub);
% Plot the Pareto front
plot(fval(:,1), fval(:,2), 'bo');
end
```
In this case, we define a function `fun` with two objectives and set the bounds for the variables. Then, we call the `gamultiobj` function and plot the Pareto front.
### 3.1.2 Custom Functions and Toolbox Integration
Custom functions provide flexibility, allowing us to modify the default behavior of genetic algorithms according to specific problem needs. For example, we can customize the fitness function, crossover function, and mutation function to better fit the problem requirements.
**Case Analysis**: We have a specific problem to solve and can write a custom fitness function and integrate it into the genetic algorithm. For example:
```matlab
function y = custom_fitness(x)
% Define a custom fitness function here
y = x(1)^2 + x(2)^2; % A simple sum of squares function
end
```
To integrate this custom function, we need to specify the `'FitnessFcn'` option in the `ga` function:
```matlab
% Set the parameters for the genetic algorithm
options = optimoptions('ga', 'FitnessFcn', @custom_fitness);
% Call the genetic algorithm
[x, fval] = ga(@custom_fitness, 2, [], [], [], [], [], [], [], options);
```
In this example, we create a function called `custom_fitness` and specify it as the fitness function through the `'FitnessFcn'` option in the `ga` function.
## 3.2 Implementation of Advanced Features of Genetic Algorithms
### 3.2.1 Parallel Computing and Acceleration Techniques
Parallel computing is an effective means to improve the efficiency of genetic algorithms, especially when dealing with large-scale problems. MATLAB provides a parallel computing toolbox that can be used in conjunction with the genetic algorithm toolbox.
**Case Analysis**: Consider a complex problem where we want to accelerate the solution using parallel computing. We can enable `ga` to execute in parallel on a multi-core processor by setting the `'UseParallel'` option to `true`.
```matlab
options = optimoptions('ga', 'UseParallel', true);
[x, fval] = ga(@custom_fitness, 2, [], [], [], [], [], [], [], options);
```
Parallel computing can be implemented by setting up multiple populations and running genetic algorithms simultaneously on multiple processor cores. This significantly reduces the total computation time.
### 3.2.2 Multi-Population Coevolution and Diversity Maintenance
To maintain population diversity and avoid premature convergence, a multi-population coevolution strategy can be used. In MATLAB, this can be achieved by creating multiple populations and allowing them to evolve independently on different processors, and then through a migration mechanism to share information, thus increasing search efficiency and the likelihood of finding global optima.
**Case Analysis**: We can create multiple populations and set different parameters for each. At certain generation intervals, by performing migration operations to exchange superior individuals, as shown below:
```matlab
nPopulations = 3; % Create 3 populations
populations = cell(nPopulations, 1); % Initialize population set
for i = 1:nPopulations
populations{i} = initPopulation(ngen, popSize, nvars); % Initialize each population
end
% Perform coevolution
for gen = 1:ngen
for i = 1:nPopulations
populations{i} = ga(@custom_fitness, nvars, [], [], [], [], [], [], [], options);
if mod(gen, migrationRate) == 0
% Perform migration operation to share information
populations{i} = migration(populations{i});
end
end
end
```
In this example, the `migration` function is responsible for individual migration and information sharing between populations.
## 3.3 Troubleshooting and Debugging of Algorithms
### 3.3.1 Common Problem Diagnostics and Solutions
When running genetic algorithms, various problems may arise, such as non-convergence, premature convergence, and poor performance. These faults can usually be solved by debugging the parameters of the genetic algorithm.
**Case Analysis**: If the algorithm is found not to converge, attempts can be made to increase the population size or the number of generations, or adjust the crossover and mutation probabilities. Here is an example of troubleshooting:
```matlab
options = optimoptions('ga', 'PopulationSize', 100, 'MaxGenerations', 100, 'CrossoverFraction', 0.8, 'MutationRate', 0.01);
[x, fval] = ga(@custom_fitness, 2, [], [], [], [], [], [], [], options);
```
### 3.3.2 Precautions During the Debugging Process
During the debugging process, the following points need special attention:
- Ensure the fitness function is correct and logical, meeting the problem requirements.
- When adjusting parameters, do not blindly increase the population size and number of generations, as this may lead to excessively high computational costs.
- Gradually adjust the crossover and mutation rates, observing changes in algorithm performance.
- Utilize MATLAB's graphical tools, such as `gaoptimset` and `gatool`, to help understand the algorithm's running status.
By following these steps, one can gain in-depth understanding and effectively debug the implementation and running process of genetic algorithms.
# Chapter 4: Advanced Methods for Improving the Performance of Genetic Algorithms
As genetic algorithms are widely applied in various fields, continuously improving their performance and efficiency has become particularly important. This chapter will delve into algorithmic innovations, variant research, and case studies and optimization of applications to provide guidance for in-depth research and practical application of genetic algorithms.
## 4.1 Algorithm Innovation and Variant Research
### 4.1.1 Hybridization of Genetic Algorithms with Other Algori***
***bining genetic algorithms with other optimization algorithms, such as local search algorithms, Particle Swarm Optimization (PSO), Ant Colony Optimization, etc., can compensate for each other's shortcomings and improve overall search efficiency and solution quality. For example, combining a genetic algorithm with a Simulated Annealing algorithm can utilize the randomness and diversity of the genetic algorithm in the global search phase, while taking advantage of the rapid convergence of Simulated Annealing in the local search phase.
### 4.1.2 Introduction to Emerging Genetic Algorithm Variants
In recent years, numerous researchers have proposed various variants based on genetic algorithms, such as Differential Evolution (DE), Evolution Strategies (ES), Adaptive Genetic Algorithm (AGA), etc. These algorithms have shown outstanding performance in specific problems. These variant algorithms improve the performance of genetic algorithms by introducing new genetic mechanisms or optimizing genetic operations. For example, Differential Evolution algorithms use differential operations to guide the search process, allowing the algorithm to have faster convergence speed and stronger robustness in dealing with continuous parameter optimization problems.
## 4.2 Application Case Analysis and Optimization
### 4.2.1 Modeling and Solution of Practical Problems
In practical applications, genetic algorithms need to be properly modeled and adjusted according to specific problems. Taking the Traveling Salesman Problem (TSP) as an example, by defining an appropriate fitness function and a reasonable encoding method, genetic algorithms can effectively find approximate optimal solutions. The key to case analysis is understanding the essence of the problem and how to design a fitness function and choose appropriate genetic operations to guide the algorithm to find a satisfactory solution.
### 4.2.2 Comparative Evaluation Before and After Algorithm Optimization
By comparing the performance of genetic algorithms before and after optimization on specific problems, the effects of algorithm optimization can be intuitively demonstrated. Evaluation criteria may include convergence speed, solution quality, and algorithm running time. For example, through comparative experiments, it can be found that genetic algorithms with adaptive mutation rates are superior to traditional genetic algorithms in terms of convergence speed and solution quality.
## 4.3 Future Trends and Research Directions
### 4.3.1 Theoretical Research Progress of Genetic Algorithms
Theoretical research on genetic algorithms is deepening, involving convergence analysis, computational complexity, and parameter adaptive mechanisms. Currently, researchers are committed to proposing more universal and robust theoretical frameworks to guide the practical application of genetic algorithms. For example, by mathematically modeling and analyzing the algorithm, its performance can be more accurately predicted, providing a theoretical basis for parameter adjustment.
### 4.3.2 Potential Optimization Space in the MATLAB Environment
MATLAB, as a powerful mathematical computing and engineering simulation platform, provides convenience for the research and application of genetic algorithms. Future research can seek algorithm optimization in the MATLAB environment, such as enhancing parallel computing capabilities, improving visualization tools, and expanding the algorithm library. For example, using MATLAB's parallel computing toolbox can significantly improve the computational efficiency of genetic algorithms for large-scale problems.
Through the discussion in this chapter, we can see that continuous innovation and optimization of genetic algorithms can bring out more powerful capabilities in solving real-world problems. At the same time, the deepening of research and the in-depth application of the MATLAB platform provide broad space for the future development of genetic algorithms.
# Chapter 5: Practical Drills and Code Examples
In Chapter 4, we explored how to improve the performance of genetic algorithms through innovative methods and analyzed and optimized some practical cases. In this chapter, we will delve into practical drills, explaining how to use MATLAB to solve linear and nonlinear problems and optimize complex system models through specific code examples.
## 5.1 Solving Linear and Nonlinear Problems
Solving linear and nonlinear problems in MATLAB can be achieved through the optimization toolbox. We will start with simple linear programming problems and gradually delve into more complex nonlinear optimization problems.
### 5.1.1 MATLAB Implementation of Linear Programming Problems
In MATLAB, linear programming can be solved using the `linprog` function. This function uses the simplex algorithm or the interior-point algorithm to find the optimal solution to linear programming problems.
```matlab
% Linear programming problem definition
f = [-1; -1]; % Objective function coefficients
A = [1, 2; 1, -1; -2, 1]; % Inequality constraint coefficient matrix
b = [2; 2; 3]; % Right-hand side values for inequality constraints
lb = zeros(2,1); % Variable lower bounds
ub = []; % Variable upper bounds (no upper bound)
% Solve the linear programming problem
[x, fval] = linprog(f, A, b, [], [], lb, ub);
% Output results
disp('Optimal solution:');
disp(x);
disp('Minimum value of the objective function:');
disp(fval);
```
### 5.1.2 MATLAB Implementation of Nonlinear Optimization Problems
The solution of nonlinear problems generally uses functions such as `fminunc` or `fmincon`. `fminunc` is used for unconstrained nonlinear optimization, while `fmincon` can be used for constrained nonlinear optimization problems.
```matlab
% Nonlinear optimization problem definition
fun = @(x) (x(1)-1)^2 + (x(2)-2.5)^2; % Objective function
% Initial guess
x0 = [0, 0];
% Option settings
options = optimoptions('fminunc', 'Algorithm', 'quasi-newton');
% Call the function to solve the unconstrained optimization problem
[x_minunc, fval_minunc] = fminunc(fun, x0, options);
% Output results
disp('Result of solving the unconstrained nonlinear optimization problem:');
disp(x_minunc);
disp(fval_minunc);
```
## 5.2 Optimization of Complex System Models Using Genetic Algorithms
Optimization of complex system models often involves various parameters and constraints. Genetic algorithms can play a significant role in this context.
### 5.2.1 Case Analysis of Engineering Optimization Problems
Suppose we need to solve an engineering optimization problem involving various material combinations and structural parameters. We aim to find the combination of materials that is the lowest cost while meeting strength requirements.
```matlab
% Define the objective function and constraints
% Since genetic algorithms involve complex solutions, only a simplified form is given here
function cost = engineeringProblem(x)
cost = x(1)*100 + x(2)*200 + ...; % Material cost calculation
% Add other constraints such as strength, size, etc.
end
% Genetic algorithm parameter settings
nvars = 10; % Number of variables
options = optimoptions('ga', 'PopulationSize', 100, 'MaxGenerations', 100);
% Call the genetic algorithm solver
[x_ga, fval_ga] = ga(@engineeringProblem, nvars, [], [], [], [], [], [], [], options);
% Output results
disp('Result of using genetic algorithms to solve engineering optimization problems:');
disp(x_ga);
disp(fval_ga);
```
### 5.2.2 Application Cases in Biology and Genetics
In biology and genetics, genetic algorithms can be used to solve problems such as gene localization and protein folding. Suppose we need to optimize a gene analysis model based on population genetic information.
```matlab
% Assume gene information is encoded as a binary string
% Define the fitness function
function fitness = geneAnalysis(x)
fitness = ...; % Calculate fitness based on gene data
end
% Genetic algorithm parameter settings
nvars = 50; % Length of each gene
options = optimoptions('ga', 'PopulationSize', 200, 'MaxGenerations', 200);
% Call the genetic algorithm solver
[x_ga, fval_ga] = ga(@geneAnalysis, nvars, [], [], [], [], [], [], [], options);
% Output results
disp('Result of using genetic algorithms for gene analysis model solving:');
disp(x_ga);
disp(fval_ga);
```
## 5.3 Code Writing and Sharing of Optimization Techniques
Writing efficient code directly affects the performance of genetic algorithms. In this section, we share some best practices for MATLAB programming and techniques for performance optimization.
### 5.3.1 Best Practices for Writing Efficient Code
- Use array operations instead of loops, leveraging MATLAB's vectorization capabilities.
- Avoid creating new variables or modifying large data structures within loops whenever possible.
- Cache parts that are repeatedly calculated.
- Use preallocated memory space for efficiency, especially during iterative processes.
- Utilize MATLAB's built-in functions, which are often optimized.
### 5.3.2 Code Optimization and Performance Enhancement Techniques
- Set option parameters reasonably in functions like `fminunc` or `fmincon`, such as algorithm types and convergence conditions.
- When using genetic algorithms, carefully design crossover and mutation strategies to prevent premature convergence.
- Use parallel computing to reduce execution time, especially when dealing with large-scale problems.
- Simplify the problem size appropriately, and for overly complex models, try breaking them into multiple sub-problems.
Through the above code examples and optimization techniques, we can effectively implement and optimize genetic algorithms in MATLAB to solve various linear and nonlinear problems. In the next chapter, we will summarize the practical effects of genetic algorithms and look forward to their future development trends.
0
0
相关推荐








